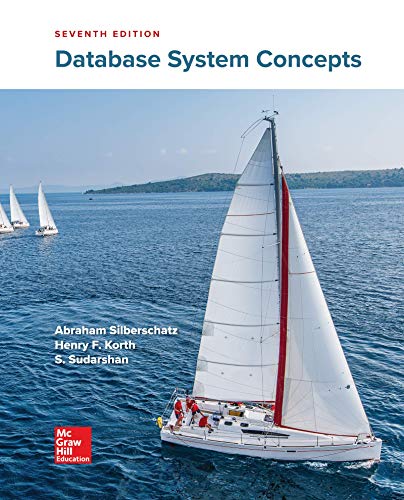
Concept explainers
- Create a program that reads the data into an array or
vector . .
Run the program with 3 grades per student using the sample data below. Calculate averages and a letter grade A= 90 to 100, B= 80 to 89, C= 70 to 79, D=60 to69, F= anything 59 or less.
Student Name |
Score 1 |
Score 2 |
Score 3 |
Average |
Mary Brown |
100 |
90 |
90 |
? |
George Smith |
90 |
30 |
50 |
? |
Dale Barnes |
80 |
78 |
82 |
? |
Sally Dolittle |
70 |
65 |
80 |
? |
Conrad Bailer |
60 |
58 |
71 |
? |
You should get the following results:
Mary Brown has an average of 93.33 which gives the letter grade of A
George Smith has an average of 56.67 which gives the letter grade of F
Dale Barnes has an average of 80.00 which gives the letter grade of B
Sally Dolittle has an average of 71.67 which gives the letter grade of C
Conrad Bailer has an average of 63.00 which gives the letter grade of D

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Write a program that simulates a lottery. The program should have an array of five integers named winningDigits, with a randomly generated number in the range of 0 through 9 for each element in the array. The program should ask the user to enter five digits and should store them in a second integer array named player. The program must compare the corresponding elements in the two arrays and count how many digits match. For example, the following shows the winningDigits array and the Player array with sample numbers stored in each. There are two matching digits, elements 2 and 4. The grand prize winner is determined by all 5 matching digits. The program should use functions for the following processes (3):Random number generation for lotteryPrompt for user data entryMatch Processing and Winner Determinationarrow_forwardEach of two players rolls a dice and whoever rolls the highest number (between 1 and 6 inclusive) gets 4 points, and if it is a draw, both players get 2 points. The player with the highest number of points after 10 rounds of the game is the winner (or the game could be drawn). The following arrays contain the results of dice rolls of each of two players in 10 consecutive rounds of the game.player1=[3, 2, 5, 6, 2, 5, 1, 6, 4, 5]player2=[4, 2, 4, 3, 6, 5, 3, 2, 4, 1] Write a MATLAB program that computes the running total points of each player and displays one of the following messages (whichever applies) after each round n for n = 1, 2, . . . , 10:Player 1 is leadingPlayer 2 is leadingIt is a tie [Hint: You should use an if construct.]Also, at the end of your program, use the find command to identify (and display) the rounds in which dice rolls were the same for the two players, and use the length command to compute (and display) the number of such rounds. Include appropriate headings in…arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a 'map' of all the underground resources in the mine. This map comes as atwo-dimensional integer array. The array has n rows and k columns. Each integer is between zero and one hundred (inclusive). This array represents a grid map of the mine – with each row representing a different height level. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and k. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit…arrow_forward
- Create a mathematical function which will print out a number in the first index of an array, the square of the number in the second index of an array, the cube of the number in the third index of an array, the square root of the number in the fourth index of an array, and the reciprocal of the number in the fifth index of an array. Run the program for the number 9. Include appropriate column headings and the main heading MATHEMATICAL TABLE. HINT: You will need to use [if-elif] structure. use phytonarrow_forwardNO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for missing or invalid…arrow_forwardThe Chat-A-While phone company provides service to six area codes and charges the per-minute rates for phone calls shown in Figure 6-25 (below). Write a program named ChatAWhile that stores the area codes and rates in parallel arrays and allows a user to enter an area code and the length of time for a call in minutes, and then display the total cost of the call. For example if the area code is in the array, such as 715, and the call length is 22 minutes, the output should be: Your phone call to area 715 costs $0.16 per minute For 22 minutes the total is $3.52 If the area code is not in the array, such as 111, the program should not accept a call length, and instead output Sorry - no calls allowed to area 111. Area Code Per-Minute Rate ($) 262 0.07 414 0.10 608 0.05 715 0.16 815 0.24 920 0.14 Figure 6-25 Per-minute phone call rates In order to prepend the $ to currency values, the program will need to use the CultureInfo.GetCultureInfo method. In order to do this,…arrow_forward
- Write a program called DeliveryCharges for the package delivery service in Exercise 4. The program should again use an array that holds the 10 zip codes of areas to which the company makes deliveries. Create a parallel array containing 10 delivery charges that differ for each zip code. Prompt a user to enter a zip code, and then display either a message indicating the price of delivery to that zip code or a message indicating that the company does not deliver to the requested zip code. In C# form please.arrow_forwardIn C++ language, write a program to print "Junior" if the given array element starts with 'J' and print "Senior" if the array element starts with 's'. Print the name of the player and the message Junior" or "Senior" accordingly. string names [5] = {"J-Liam", "S-Naoh", "S-Elijah", "J-James", "S-Henry"};arrow_forwardThis program will store roster and rating information for a soccer team. Coaches rate players during tryouts to ensure a balanced team. (1) Prompt the user to input five pairs of numbers: A player's jersey number (0 - 99) and the player's rating (1 - 9). Store the jersey numbers in one int vector and the ratings in another int vector. Output these vectors (i.e., output the roster).Ex: Enter player 1's jersey number: 84 Enter player 1's rating: 7 Enter player 2's jersey number: 23 Enter player 2's rating: 4 Enter player 3's jersey number: 4 Enter player 3's rating: 5 Enter player 4's jersey number: 30 Enter player 4's rating: 2 Enter player 5's jersey number: 66 Enter player 5's rating: 9 ROSTER Player 1 -- Jersey number: 84, Rating: 7 Player 2 -- Jersey number: 23, Rating: 4 ... (2) Implement a menu of options for a user to modify the roster. Each option is represented by a single character. Following the initial 5 players' input and roster output, the program outputs the menu. The…arrow_forward
- In Python: Write a program that reads two sets of exam scores of five students from user input and stores the scores into two NumPy arrays (exam1 and exam2). The program then calculates the average of the two exams for each of the five students and outputs the average scores as a NumPy array. The program finally counts the number of average scores that are 80 or higher and outputs the count. Ex: If the input of the two sets of scores are: 75 80 65 82 92 88 85 75 95 73 where [75 80 65 82 92] is stored in NumPy array exam1, and [88 85 75 95 73] is stored in NumPy array exam2. The program then outputs: Average scores: [81.5 82.5 70. 88.5 82.5] Number of students who received 80 and above: 4arrow_forwardSuppose a teacher has five students who have taken four tests. The teacher uses the following grading scales to assign a letter grade to a student, based on the average of his or her four test scores. Test Score Letter Grade 90-100 80-89 70-79 60-69 0-59 A B C D F Create an application that uses an array of strings to hold the five student names, an array of five strings to hold each student's letter grades, and five arrays of four single precision numbers to hold each student's set of test scores.arrow_forwardPlease give me correct solution.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
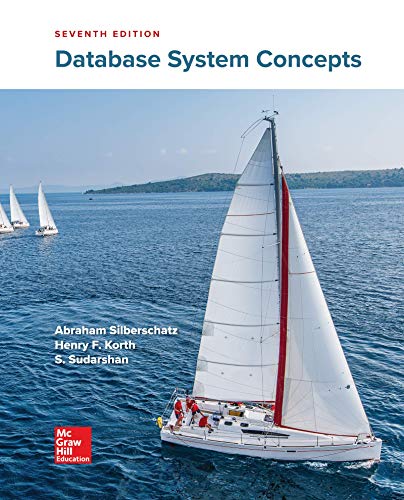
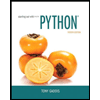
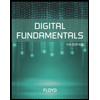
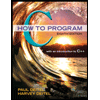
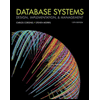
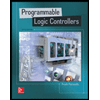