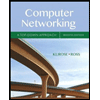
Create a python script following the below guidelines. I will enter my python starter script at the bottom under the requirements. I will also attach a screenshot of my excel file and desired outcome. This is an exercise I'm trying to understand.
complete a
Specifically, open the starter code given in monthly_sales_starter.pyfile. Read and understand the existing code –it has implemented the functions that handle printing welcome messages and the rules, and also the main function that handles the command loop. You are supposed to implement the following functions that either read/write data or perform one of the commands. Here’s what each function is supposed to do:
1.read_sales: Function to read contents of FILENAME and return the contents list of lists. Since csv returns string values for all columns, the function should convert the sales number (2nditem in each row) to integers. Use exception handling code so that if the file is not found, the function gives feedback that the file was not found and exits the program.(See sample run #2 below)
2.write_sales: Function to write the contents of sales, alist of lists, to FILENAME.
3.view_monthly_salesFunction accepts a list of lists with monthly sales values and prints the values of the monthly sales.(See sample run #1below)
4.view_yearly_summary: Function accepts a list of lists with monthly sales data, and prints the yearly summary of the sales values: yearly total and the monthly average sales.Print average using up to 2 decimal places.(See sample run #1below)
5.edit: Function accepts a list of lists with monthly sales values, and lets the user edit the sales data for one of the months. Specifically,
a.promptsthe user for a 3-letter month code. Raises an exception if the user enters an invalid code. (See sample run #3below)
b.promptsthe user for the new sales numbers as an integer.
c.Use a try/except block that will handle invalid input cases, give user correct feedback and return.
d.In case of valid input, program should update the csv file with new data.
See the sample run below using the sample input csv file.
##my start code
import csv
import sys
# a file in the current directory
FILENAME = "monthly_sales.csv"
def read_sales():
''' Function to read contents of FILENAME and return the contents as
a list of lists. If the file is not found, the function should give feedback that
the file was not found and exit the program.
Since CSV returns string values for all columns, the function should convert
the sales number to integers.'''
pass
def write_sales(sales):
''' Function to write the contents of sales, a list of lists, to
FILENAME.'''
pass
def view_monthly_sales(sales):
''' Function to print the values of the monthly sales.
'''
pass
def view_yearly_summary(sales):
''' Function to print the yearly summary of the sales values: it should print the
yearly total and the monthly average sales.'''
# helper list
names = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
pass
def edit(sales):
''' Let the user edit the sales value for one of the months.
- Prompt the user for a 3-letter month code. Raise an exception if the user enters an
invalid code.
- Prompt the user for the new sales numbers.
- Use a try/except block that will handle invalid input in both cases.
'''
pass
def display_menu():
print("COMMAND MENU")
print("monthly - View monthly sales")
print("yearly - View yearly summary")
print("edit - Edit sales for a month")
print("exit - Exit program")
print()
def main():
print("Monthly Sales program")
print()
sales = read_sales()
display_menu()
while True:
command = input("Command: ")
if command == "monthly":
view_monthly_sales(sales)
elif command == "yearly":
view_yearly_summary(sales)
elif command == "edit":
edit(sales)
elif command == "exit":
break
else:
print("Not a valid command. Please try again.\n")
print("Bye!")
if __name__ == "__main__":
main()
##end of my code things called in main
CSV/Excel doc
Jan | 14317 |
Feb | 5892 |
Mar | 1073 |
Apr | 3463 |
May | 2429 |
Jun | 4324 |
Jul | 9762 |
Aug | 15578 |
Sep | 2437 |
Oct | 6735 |
Nov | 88 |
Dec | 239 |
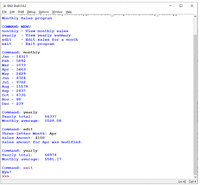
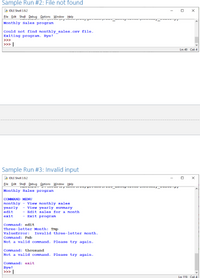

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- In this exercise, you modify the Gross Pay application from this lesson. Use Windows to make a copy of the Gross Pay Solution folder. Rename the copy Modified Gross Pay Solution. Open the Gross Pay Solution (Gross Pay Solution.sln) file contained in the Modified Gross Pay Solution folder. Open the designer and Code Editor windows. Locate the form’s Load event procedure. Change both For…Next statements to Do…Loop statements. Save the solution and then start and test the application. Close the Code Editor window and then close the solution.arrow_forwardCurve the final exam grades 14 points and rank students from 1 to 50 with 1 being the highestscore and 50 (being the lowest). The rank must appear in the output. It’s ok if some ranks “tie”.arrow_forwardThis should be done with Visual Studio C# console app net core Thanks!arrow_forward
- I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide! CODE STARTS HERE: #include<stdio.h>#include<stdlib.h> struct node{ int key; struct node *left, *right;}; // A utility function to create a new BST nodestruct node *newNode(int item){ struct node *temp = (struct node *)malloc(sizeof(struct node)); temp->key = item; temp->left = temp->right = NULL; return temp;} // A utility function to do inorder traversal of BSTvoid inorder(struct node *root){ if (root != NULL) { printf("("); inorder(root->left); printf("%d", root->key); inorder(root->right); printf(")");…arrow_forwardFor this lab, you will be doing the following in C#: 1) Create a Home form with buttons that when clicked, will open the Account, Email, and eventually the Contact forms. The Account form should be opened in "View" mode. 2) Add code to the Login form so the Account form opens when the user clicks "Create New Account". The Account form should be opened in "Modify" mode. 3) Add code to the Login form that validates the user input before allowing the user the access the Home form. For now, just verify the user enters "user1" and "12345" for the username and password respectively.arrow_forwardPlease make sure the output be the same exact output on the picture (In python)arrow_forward
- please do not change variable name. will give thumbs down if variable name is changed. also please have it so I can copy and pastearrow_forwardHello can you please provide the flowgorithm chart for this assignment? Thaarrow_forwardIn cengage mindtap The credit plan at TidBit Computer Store specifies a 10% down payment and an annual interest rate of 12%. Monthly payments are 5% of the listed purchase price, minus the down payment. Write a program that takes the purchase price as input. The program should display a table, with appropriate headers, of a payment schedule for the lifetime of the loan. Each row of the table should contain the following items: The month number (beginning with 1) The current total balance owed The interest owed for that month The amount of principal owed for that month The payment for that month The balance remaining after payment The amount of interest for a month is equal to balance × rate / 12. The amount of principal for a month is equal to the monthly payment minus the interest owed.arrow_forward
- How do you separate this code into a header file, implementation file, and the application file?arrow_forwardInheritance and Polymorphism This assignment starts with the Account class from Labs 4 and 5. Our new and improved bank has two kinds of accounts, savings, and checking. In addition to an ID and a balance, savings accounts have an annual interest rate and a minimum balance. Checking accounts have a minimum balance and a monthly fee. Withdrawals and deposits can be made to both kinds of accounts. At the end of each month, savings accounts pay interest, but only if the account's balance is above the minimum. Also at the end of each month, a fee is deducted from each checking account, but the fee is waived if the account's balance is over the minimum. Tasks 1. Create a complete UML diagram which shows the relationships between the three classes, Account, Savings, and Checking and all of the fields and methods in each. The only modifications required to the Account class is the addition of two abstract methods called closeMonth and accountString. 2. The closeMonth function performs the…arrow_forwardA company approaches you to create an algorithm for calculating the tax and the tip on a restaurant bill. Assume that the application will only tip on the food and beverage total without the tax added in. Your algorithm will allow the user to input the amount of the tip in custom mode or your algorithm will give the tip in 12%, 15%, 18%, 20% recommended increments. Your job is to create the first three steps of the problem-solving process for this algorithm design. Please make sure that you explore the problem space to determine the criteria and constraints in Part 1. Be creative think of all the inputs and outputs you will be using. For Part 2 think of all the questions that you might have in determining how to actually make such a program. And for Part 3 make sure that you specify (pencil and paper – take a picture of it and include it in your document) a couple of examples of your algorithmic process.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
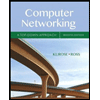
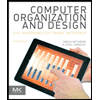
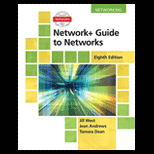
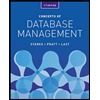
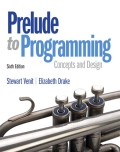
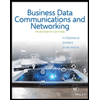