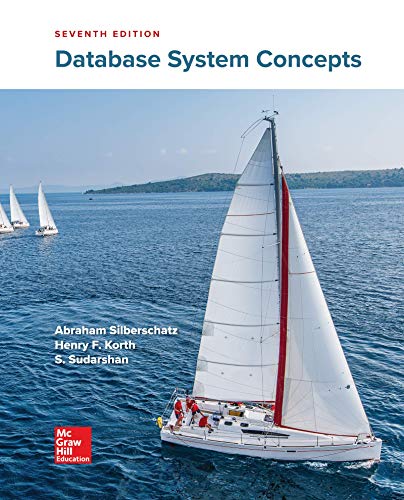
Create a void function, “displayMenu”, for the menu that is used for
selecting the options. The function declaration is void displayMenu();
Create a function “callExponentialFunction”. The function body contains
all code for getting user input and returning the value of the call of the
predefined “exp” library function. The function declaration is below.
double callExponentialFunction();
Create a function “callLogarithmFunction”. The function body contains all
code for getting user input and returning the value of the call of the
predefined “log10” library function. The function declaration is below.
double callLogarithmFunction();
Create a function “callMaximumFunction”. The function body contains all
code for getting user input and returning the value of the call of the
predefined “fmax” library function. The function declaration is below.
double callMaximumFunction();
Create a function “callMinimumFunction”. The function body contains all
code for getting user input and returning the value of the call of the
predefined “fmin” library function. The function declaration is below.
double callMinimumFunction();
Create a function “callIntegerDivisonFunction”. The function body contains
all code for getting user input and returning the value of the call of the
predefined “div” library function. The function declaration is below.
div_t callIntegerDivisionFunction();
The return value will not be displayed (i.e., call like void function).
The “main” function of the application should call the appropriate functions.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Codingarrow_forwardWhich of the following is/are true of a function marked as constexpr in C++17? Select all that apply. Pick ONE OR MORE options Any call to this function will be evaluated at compile time Any call to this function that is assigned to a constexpr variable will be evaluated at compile time The function cannot be a virtual member function, including a virtual special member function The function may only invoke other constexpr functions The function may only use ~if constexpr~ and may not use "regular" ~if~arrow_forward1.In your game console class, add a start function which should prompt the user for an email address. -Use a regular expression to validate that it is a valid email address and prompt them again until they enter a valid one. -Next, prompt the user to enter a credit card number and use a regular expression to validate the credit card. -Prompt them again until they enter a valid one and once done, display the game menu. Existing Code below: class Game {constructor(name){this.name = name;}} class GameConsole{constructor(){this.games = [];} load(){var gameNames = ["Zelda", "Halo", "Mario", "The God Among Us\n"];for (var i = 0; i < gameNames.length; i++) {let game = new Game(gameNames[i]);this.games.push(game);}} log(){console.log("Games Loaded:");for (var i = 0; i < this.games.length; i++) {var name = this.games[i].name;console.log(name);}}} let gamecon = new GameConsole();gamecon.load();gamecon.log(); const prompt = require('prompt-sync')(); var adventurersName = ["Captain…arrow_forward
- C++ programming 48.In the function prototype void action(int& value); The argument value is said to be a ___________ parameter. 49.In the previous problem, suppose the function action updated its argument. True or false: the variable value in the caller would be updated. ________________ 50.True or false: the following two prototypes represent valid overloaded functions _________:double fna(double,int);float fna(float,int);arrow_forwardAssume there is a function called mystery. It already has the following prototype: void mystery(bool flag); Which of the following prototypes would not be considered a proper overload for mystery? void mystery(bool valid); void mystery(string msg, double x); void mystery(int num); void mysteryO:arrow_forwardin phython language Create a package folder named shapes. Inside this package, place two more package folders: 2d and 3d. Inside 2d package, place a module file: circle.py circle.py module has two function definitions: areaCircle(), circCircle(). areaCircle() function takes a parameter: radius, calculates and returns the area of the circle. You may assume PI is 3.14. circCircle() function also takes a parameter: radius, calculates and returns the circumference of the circle. Inside 3d package, place two module files: sphere.py and cylindir.py sphere.py module has two function definitions: areaSphere() and volumeSphere() which both take a parameter for radius, calculates and returns area and volume of a sphere respectively. cylindir.py module has two function definitions: areaCylindir() and volumeCylindir() which both take two parameters: height and radius. They calculate area and volume of a cylindir and return the values respectively. You may find the formulas for those shapes…arrow_forward
- 1. Write a function that has 2 parameters. The first parameter represents the id of the 1st textbox, and the second parameter represents the id of the 2nd textbox. In the function, write the text from the 1st textbox to the 2nd textbox concatenated with the text this was in textbox 1?arrow_forward3. Which among the following shows a valid use of the Direction enumeration as a parameter to the moveCharacter function? Select al that apply. enum Direction { case north, south, west, east}func moveCharacter(x: Int, y: Int, facing: Direction) {// code here} moveCharacter(x: 0, y: 0, facing: .southwest) moveCharacter(x: 0, y: 0, facing: Direction.north) moveCharacter(x: 0, y: 0, facing: .south) moveCharacter(x: 0, y: 0, facing: Direction.northeast)arrow_forwardC++ Write a function named “createPurchaseOrder” that accepts the quantity(integer), the cost per item (double), and the description (string). It will return anewly created PurchaseOrder object holding that information if they are valid:quantity and cost per item cannot be negative and the description cannot be empty or blank(s). When it is invalid, it will return NULL to indicate that it cannot create such PurchaseOrder object. PurchaseOrder createPurchaseOrder(int quantity, double costPerItem, string description) { if(quantity < 0 || costPerItem < 0.0 || description == "") return NULL; else { PurchaseOrder p = new PurchaseOrder(quantity,costPerItem,description); return p; } } what's the issue with this function and how to rewrite itarrow_forward
- C++ Create a Temperature class that internally stores a temperature in degrees Kelvin. However, create functions named setTempKelvin, setTempFahrenheit, and setTempCelsius that takes an input temperature in the specified temperature scale, converts the temperature to Kelvin, and stores that temperature in the class member variable. Also create functions that return the stored temperature in degrees Kelvin, Fahrenheit, or Celsius. Write a main function to test your class. Use the equations below to convert between the three temperature scales. Kelvin = Celsius + 273.15Celsius = (5/9) X (Fahrenheit - 32)arrow_forwardCreate a new module named beautyid.py. Define a function named beautyidtag that accepts 4 parameters: face, hair, number, vitamins. The function should create a 10-digit identifier composed of the first 2 letters of the user input for the variable face, first 2 letters from the user input for the variable hair, the number from the variable number, the number of vitamins, and a random integer between 11 and 90. Identifiers must all be uppercase. Return the value of the 10-digit identifier back to the calling method when called. I have made another module called beautyinventory.py where the variables are stored. I'm going to import this module back into beautyinventory.pyarrow_forwardC++YOU ONLY NEED TO EDIT date.h, dateOFYear.h, and dateOFYear.cpp date.h | date.cpp | dateOfYear.h | dateOfYear.cpp | dateTest.cpp You have been provided a Date class. Each date contains day and month parameters, a default and a custom constructor, mutator and accessor functions for the parameters, and input and output functions called within operator >> and <<. You will be writing a DateOfYear class, which expands on the Date class by adding a year parameter. This will be achieved via inheritance – DateOfYear is the derived class of the Date base class. In DateOfYear, will need to write… The include guards for the class A default constructor that initializes all parameters to 1 A custom constructor that takes in a month, day, and year to initialize the parameters Mutator and accessor for the year parameter Redefined versions of inputDate and outputDate that takes into account the new year parameter. The driver program, dateTest.cpp, is already set for use with both Date…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
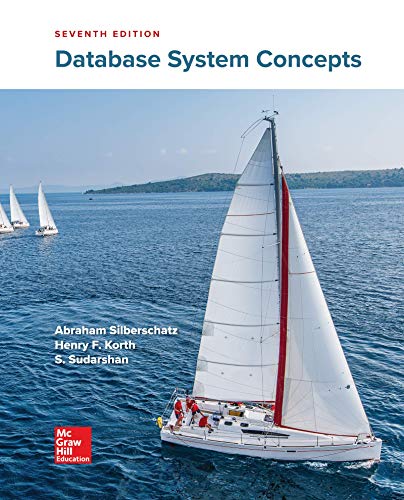
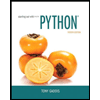
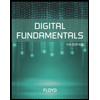
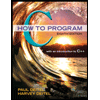
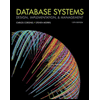
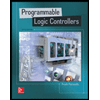