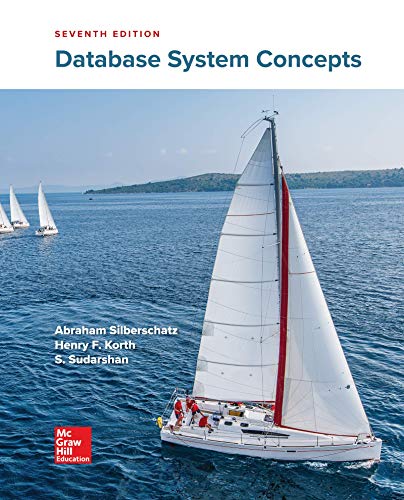
Concept explainers
Create class that performs different functions on Strings that are sent to it.
All the methods you create will be static. Only two methods (mentioned below) and the main
method need to be public. Any other methods that you write to help these methods should be
private because they are only used internally within the class.
Requirements:
1. Prompt users to enter 3 string inputs. Consider strings with alphabetic characters (a - z, A - Z)
and/or numbers (0 - 9) as valid. Strings contain only non-word characters are invalid. Print the following usage when an input string is invalid or is
empty.
Usage: Enter a string that contains alphabetic characters and numbers
2. Create a method "isPalindrome" that takes a String as a parameter and returns true if the
given String parameter is a palindrome and false if it is not. In your main method, this method
should be call with the first user input and print either "The given string is a palindrome." or
"The given string is not a palindrome." as output based on the returned value.
A word is a palindrome if it reads the same forwards and backwards. For example, the
word "level" is a palindrome. The idea of a palindrome can be extended to phrases or
sentences if we ignore details like punctuation. Here are two familiar examples:
Madam, I'm Adam
A man, a plan, a canal: Panama
We can recognize these more elaborate examples as palindromes by considering the text
that is obtained by removing all spaces and punctuation marks and converting all letters
to their lower-case form.
Madam, I'm Adam ==> madamimadam
A man, a plan, a canal: Panama ==> amanaplanacanalpanama
If the "word" obtained from a phrase in this manner is a palindrome, then the phrase is a
palindrome. Your method should also ignore the case of the letters. A palindrome is
determined by considering only alphabetic characters (a - z, A - Z) and numbers (0 - 9)
as valid text.
3. Create another method named "findSubstring" that takes 3 strings as parameters and checks if
the first string contains a substring that starts with the second string, ends with the third string,
and has an even number of characters in between†. Return the substring if it exists and return
null if not. This method will be called in your main method with all three user inputs and print
either "The substring is <substring>" or "No such substring in the given string."‡ as output
based on the returned value. Your method should ignore the letter case and assume that no more
than one such substring exists in the initial string.
4. Your output should be like the following test samples.
Sample Test Cases:
Test Case 1:
Please enter a string:
ATGCGATAC6TA
Please enter a string:
atg
Please enter a string:
6ta
The given string is "ATGCGATAC6TA" Notes:Count only alphabetic characters and numbers.
Replace <substring> with corresponding values.
The prefix is "atg"
The suffix is "6ta"
The given string is not a palindrome.
The substring is "ATGCGATAC6TA"
Program Completed
Test Case 2:
Please enter a string:
*^_^*
Usage: Enter a string that contains alphabetic characters and numbers
Please enter a string:
Was it a car or a cat i saw?
Please enter a string:
car
Please enter a string:
cow
The given string is "Was it a car or a cat i saw?"
The prefix is "car"
The suffix is "cow"
The given string is a palindrome.
No such substring in the given string.
Program Completed
Test Case 3:
Please enter a string:
Lewd did I live, & evil I did dwel.
Please enter a string:
did
Please enter a string:
did
The given string is "Lewd did I live, & evil I did dwel."
The prefix is "did"
The suffix is "did"
The given string is a palindrome.
The substring is "did I live, & evil I did"
Program Completed
Notes:
The program should always print 'Program Completed' before exiting.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- bootstrap class for form control is class="form-control-file" class="input-group-text" class="form-control"arrow_forward• Use a while loop • Use multiple loop controlling conditions • Use a boolean method • Use the increment operator • Extra credit: Reuse earlier code and call two methods from main Details: This assignment will be completed using the Eclipse IDE. Cut and paste your code from Eclipse into the Assignment text window. This is another password program. In this case, your code is simply going to ask for a username and password, and then check the input against four users. The program will give the user three tries to input the correct username-password combination. There will be four acceptable user-password combinations: • alpha-alpha1 • beta-beta1 • gamma-gamma1 • delta - delta1 If the user types in one of the correct username-password combinations, then the program will output: "Login successful." Here are a couple of example runs (but your code needs to work for all four user-password combinations): Username: beta Type your current password: beta1 Login successful. Username: delta Type…arrow_forwardPendant Publishing edits multi-volume manuscripts for many authors. For each volume, they want a label that contains the author’s name, the title of the work, and a volume number in the form Volume 9 of 9. For example, a set of three volumes requires three labels: Volume 1 of 3, Volume 2 of 3, and Volume 3 of 3. Design an application that reads records that contain an author’s name, the title of the work, and the number of volumes. The application must read the records until eof is encountered and produce enough labels for each work. The flowchart must include a call symbol, at the beginning, to redirect the input to the external data file. create a solution algorithm using pseudocode create a flowchart using RAPTORarrow_forward
- Javaarrow_forwardChange this code to show strings that help you cast twelve dice. They should be shown one at a time like this:The first one was A. The second one was B. Afterward, the sum should be shown on a different line.arrow_forwardPlease help me this I need answer typing clear urjent no chatgpt used i will give 5 upvotesarrow_forward
- Please submit a flowchart of your program for your project below. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the final average or grade. I would like the system to display the…arrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forwardIn python Add the following four methods to your Crew class: move(self, location): This takes in a location as a string, along with self, and attempts to move the crew member to the specified location. If location is one of the five valid location options ("Bridge", "Medbay", "Engine", "Lasers", or "Sleep Pods"), then this should change self.location to that new value. Otherwise, the function should print out the message: Not a valid location. repair(self, ship): first_aid(self, ship): fire_lasers(self, ship, target_ship, target_location): The above three methods represent tasks that a basic Crew member is not capable of (but one of its derived classes will be able to accomplish). So each of them should simply print out a message of the form: <Name> doesn't know how to do that. Examples: Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. if __name__ == '__main__': crew1…arrow_forward
- python: def shakespeare_position(role, section): """ Question 2 - Regex You are reading a Shakespeare play with your friends (as one frequently does) and are given a role. You want to know what line immediately precedes YOUR first line in a given section so that you are ready to go when it is your turn. Return this line as a string, excluding the character's name. Lines will always begin with the character's name followed by a ':' and end in a "." or a "?" Each line is separated by a single space. THIS MUST BE DONE IN ONE LINE. "" Args: role (str) section (str) Returns: str section_1 = 'Benvolio: By my head, here come the Capulets. Mercutio: By my heel, I care not. ' + 'Tybalt: Gentlemen, good den - a word with one of you. Mercutio: And but one word with one of us?' >>> shakespeare_position('Tybalt', section_1) 'By my heel, I care not.' >>> shakespeare_position('Mercutio', section_1) 'By my head, here…arrow_forwardJAVA PROGRAM ASAP Please CREATE A program ASAP BECAUSE IT IS LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #16. Morse Code Converter Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Write a program that asks the user to enter a string, and then converts that string to Morse code and prints on the screen. Use hyphens for dashes and periods for dots. The Morse code table is given in a text file morse.txt. When printing morse code, display eight codes on each line except the last line. Codes should be separated from each other with one space. There should be no extra spaces at the beginning and at the end of the output. Uppercase and lowercase letters are translated the same way. Morse.txt 0 -----1 .----2 ..---3 ...--4 ....-5 .....6 -....7 --...8 ---..9 ----.,…arrow_forwardPractice / Frequency of Characters Write a method that returns the frequency of each characters of a given String parameter. If the given String is null, then return null If the given String is empty return an empty map Example input: output: empty map explanation: input is empty Example input: null output: null explanation: input is null. Since problem output is focused on the frequency we can comfortably use Map data structure. Because we can use characters as key and the occurrences of them as value. Example input: responsible output: {r=1, e=2, s=2, p=1, o=1, n=1, i=1, b=1, l=1} explanation: characters are keys and oc values ences arearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
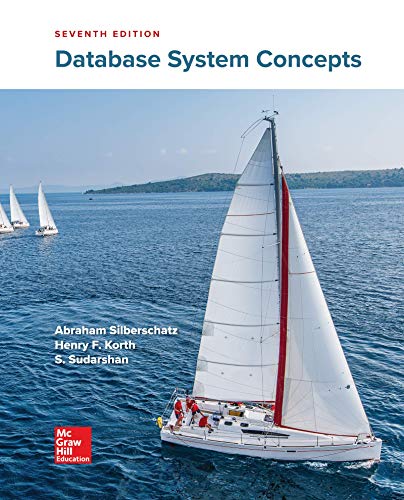
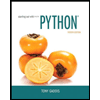
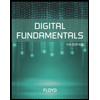
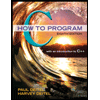
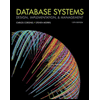
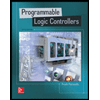