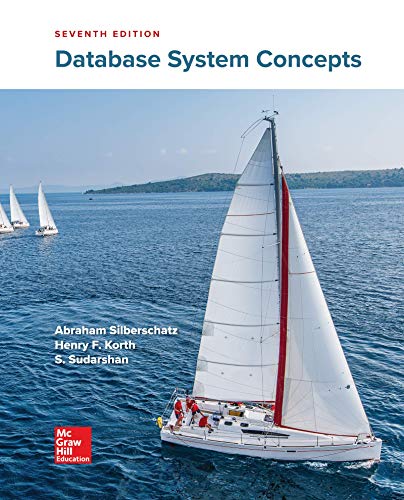
Concept explainers
8.19 LAB*: Program: Playlist
You will be building a linked list. Make sure to keep track of both the head and tail nodes.
(1) Create three files to submit.
- PlaylistNode.h - Class declaration
- PlaylistNode.cpp - Class definition
- main.cpp - main() function
Build the PlaylistNode class per the following specifications. Note: Some functions can initially be function stubs (empty functions), to be completed in later steps.
- Default constructor (1 pt)
- Parameterized constructor (1 pt)
- Public member functions
- InsertAfter() - Mutator (1 pt)
- SetNext() - Mutator (1 pt)
- GetID() - Accessor
- GetSongName() - Accessor
- GetArtistName() - Accessor
- GetSongLength() - Accessor
- GetNext() - Accessor
- PrintPlaylistNode()
- Private data members
- string uniqueID - Initialized to "none" in default constructor
- string songName - Initialized to "none" in default constructor
- string artistName - Initialized to "none" in default constructor
- int songLength - Initialized to 0 in default constructor
- PlaylistNode* nextNodePtr - Initialized to 0 in default constructor
Ex. of PrintPlaylistNode output:
Unique ID: S123(2) In main(), prompt the user for the title of the playlist. (1 pt)
Ex:
(3) Implement the PrintMenu() function. PrintMenu() takes the playlist title as a parameter and outputs a menu of options to manipulate the playlist. Each option is represented by a single character. Build and output the menu within the function.
If an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. Call PrintMenu() in the main() function. Continue to execute the menu until the user enters q to Quit. (3 pts)
Ex:
(4) Implement "Output full playlist" menu option. If the list is empty, output: Playlist is empty (3 pts)
Ex:
(5) Implement the "Add song" menu item. New additions are added to the end of the list. (2 pts)
Ex:
(6) Implement the "Remove song" function. Prompt the user for the unique ID of the song to be removed.(4 pts)
Ex:
(7) Implement the "Change position of song" menu option. Prompt the user for the current position of the song and the desired new position. Valid new positions are 1 - n (the number of nodes). If the user enters a new position that is less than 1, move the node to the position 1 (the head). If the user enters a new position greater than n, move the node to position n (the tail). 6 cases will be tested:
- Moving the head node (1 pt)
- Moving the tail node (1 pt)
- Moving a node to the head (1 pt)
- Moving a node to the tail (1 pt)
- Moving a node up the list (1 pt)
- Moving a node down the list (1 pt)
Ex:
CHANGE POSITION OF SONG
(8) Implement the "Output songs by specific artist" menu option. Prompt the user for the artist's name, and output the node's information, starting with the node's current position. (2 pt)
Ex:
(9) Implement the "Output total time of playlist" menu option. Output the sum of the time of the playlist's songs (in seconds). (2 pts)
Ex:

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- c++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A FastCritter moves twice as fast as a regular critter.*/class . . .{public:void move(int steps);}; . . . int main(){FastCritter speedy;speedy.move(10);cout << speedy.get_history() << endl;cout << "Expected: [move to 20]" << endl;speedy.move(-1);cout << speedy.get_history() << endl;cout << "Expected: [move to 20, move to 18]" << endl;return 0;} Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include <string> #include <vector> using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank history. */ Critter();…arrow_forwardFirst programming homework Create a class of records for a gradebook called Rec. The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade. Build two constructors: a default constructor and a constructor that takes the first and last name. Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade. Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields. Build a function to calculate the average grade field. Build an overloaded operator == to compare two records. This should be implemented as a friend function. Declare the constructors and functions in the body of the class, but implement them outside the class. I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same…arrow_forwardPart a) Create a Rectangle class. This class stores only the Cartesian coordinates of the four corners of the rectangle. 2,3 4,3 2,0 4,0 The constructor calls a set function that accepts four sets of coordinates and verifies that each of these is in the first quadrant with no single x- or y-coordinate larger than 20.0. The set function also verifies that the supplied coordinates do, in fact, specify a rectangle.(Hint is given for a shape to be rectangle) Part b) Provide member functions that calculate the length, width, perimeter, and area. The length is the larger of the two dimensions. Part c) Include a predicate function IsSquare that determines whether the rectangle is a square. (all sides are equal) and prints a message accordingly. Please attach your algorithm/steps you choose for your problem solution.arrow_forward
- Computer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardJAVA 8.13 LAB: Movie show time display Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program…arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forward
- C++arrow_forwardc++ A FastCritter moves twice as fast as a regular critter. When asked to move by n steps, it actually moves by 2 * n steps. Implement a FastCritter class derived from Critter whose move function behaves as described. Complete the following file: fastcritter_Tester.cpp #include <iostream>using namespace std; #include "critter.h" /**A FastCritter moves twice as fast as a regular critter.*/class FastCritter:public Critter{public:void move(int steps);}; . . . int main(){FastCritter speedy;speedy.move(10);cout << speedy.get_history() << endl;cout << "Expected: [move to 20]" << endl;speedy.move(-1);cout << speedy.get_history() << endl;cout << "Expected: [move to 20, move to 18]" << endl;return 0;} Use the following file: critter.h #ifndef CRITTER_H #define CRITTER_H #include <string> #include <vector> using namespace std; /** A simulated critter. */ class Critter { public: /** Constructs a critter at position 0 with blank…arrow_forward#include <iostream> #include <fstream> using namespace std; /* This program declares a class called Inventory that has itemnNumber (which contains the id number of a product) and numOfItem (which contains the quantity on hand of the corresponding product)as private data members.The program will read these values from a file and store them in an array of objects (of type Inventory). It will then print these values to the screen. Example: Given the following data file: 986 8 432 24 This program reads these values into an array of objects and prints the following: Item number 986 has 8 items in stock Item number 432 has 24 items in stock */ const NUMOFPROD = 10; // This holds the number of products a store sells class Inventory {public: void getId(int item); // This puts item in the private data member // itemNumber of the object that calls it. void getAmount(int num); // This puts num in the private data member // numOfItem of the object that calls it.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
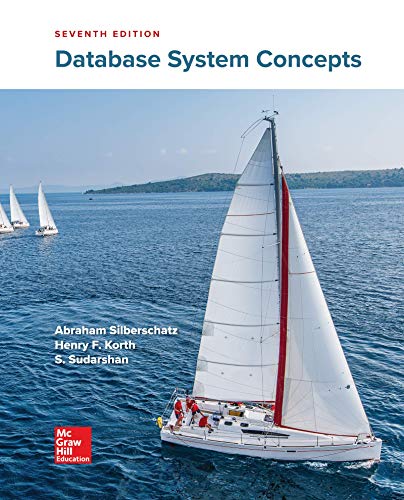
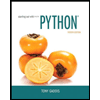
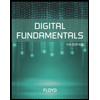
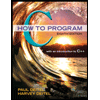
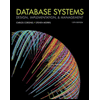
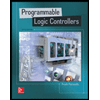