Data structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects. 2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject) 3) Write a program that thoroughly tests all the methods in the class "AList". 4) Include comments to all source code.
Data structure & Algorithum java program 1) Add a constructor to the class "AList" that creates a list from a given array of objects. 2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject) 3) Write a program that thoroughly tests all the methods in the class "AList". 4) Include comments to all source code.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Data structure & Algorithum java
1) Add a constructor to the class "AList" that creates a list from a given array of objects.
2) Add a method "getPosition" to the "AList" class that returns the position of a given object in the list. The header of the method is as follows : public int getPosition(T anObject)
3) Write a program that thoroughly tests all the methods in the class "AList".
4) Include comments to all source code.
![public class AList<T> implements ListInterface<T> {
private T[] list;
private int number0fEntries;
private boolean integrity0K;
private static final int DEFAULT CAPACITY =
private static final int MAX CAPACITY =
25;
1000;
public AList() throws Exception {
this(DEFAULT CAPACITY);
public AList(int initialCapacity) throws Exception {
= false;
integrityOK
// is capacity too small?
if(initialCapacity < DEFAULT_CAPACITY)
initialCapacity
= DEFAULT CAPACITY;
else
checkCapacity(initialCapacity);
(T[]) new Object[initialCapacity + 1];
T[] tempList =
list = tempList;
number0fEntries = 0;
integrityOK
%3D
true;
private void checkCapacity(int capacity) throws Eception {
if(capacity > MAX_CAPACITY)
throw new Exception ("Attempt to create a list whos capacity exceeds the max.");
}
@Override
public void add(T newEntry) {
add (number0fEntries + 1, newEntry);
@Override
public void add(int newPosition, T newEntry) {
try {
checkIntegrity();
if((newPosition >= 1) && (newPosition <= number0fEntries + 1)) {
if(newPosition <= numberOfEntries)
makeRoom(newPosition);
list[newPosition] = newEntry;
numberofEntries++;
ensureCapacity();
%3D
} catch(Exception e) {
System.out..println(e.getMessage (0);
private void ensureCan](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdad5a646-4583-4c4b-beba-29e57d936914%2F5368ca6d-ccb5-40bd-bb12-92101512392a%2Fpb8czqc_processed.jpeg&w=3840&q=75)
Transcribed Image Text:public class AList<T> implements ListInterface<T> {
private T[] list;
private int number0fEntries;
private boolean integrity0K;
private static final int DEFAULT CAPACITY =
private static final int MAX CAPACITY =
25;
1000;
public AList() throws Exception {
this(DEFAULT CAPACITY);
public AList(int initialCapacity) throws Exception {
= false;
integrityOK
// is capacity too small?
if(initialCapacity < DEFAULT_CAPACITY)
initialCapacity
= DEFAULT CAPACITY;
else
checkCapacity(initialCapacity);
(T[]) new Object[initialCapacity + 1];
T[] tempList =
list = tempList;
number0fEntries = 0;
integrityOK
%3D
true;
private void checkCapacity(int capacity) throws Eception {
if(capacity > MAX_CAPACITY)
throw new Exception ("Attempt to create a list whos capacity exceeds the max.");
}
@Override
public void add(T newEntry) {
add (number0fEntries + 1, newEntry);
@Override
public void add(int newPosition, T newEntry) {
try {
checkIntegrity();
if((newPosition >= 1) && (newPosition <= number0fEntries + 1)) {
if(newPosition <= numberOfEntries)
makeRoom(newPosition);
list[newPosition] = newEntry;
numberofEntries++;
ensureCapacity();
%3D
} catch(Exception e) {
System.out..println(e.getMessage (0);
private void ensureCan
![private void ensureCapacity() throws Exception {
= list.length
if (numberofEntries >= capacity) {
int capacity
1;
int newCapacity = 2 * capacity;
checkCapacity(newCapacity);
list =
Arrays.copy0f(list, newCapacity + 1);
%3D
private void makeRoom(int newPosition) {
int newIndex =
newPosition;
int lastIndex = number0fEntries;
for (int index = lastIndex; index >= newIndex; index--) {
list[index + 1] = list[index];
private void checkIntegrity() throws Exception {
if(lintegrityOK)
throw new Exception("AList object is corrupt.");
}
@Override
public T remove (int givenPosition) {
// TODO Auto-generated method stub
return null;
@Override
public void clear() {
// TODO Auto-generated method stub
@override
public T replace(int givenPosition, T newEntry) {
// TODO Auto-generated method stub
return null;
@override
public T getEntry(int givenPosition) {
// TODO Auto-generated method stub
return null;
@override
public T[] toArray() {
try {
checkIntegrity();
T[] result = (T[]) new Object[numberofEntries];
for (int index = 0; index < number0fEntries; index++)
result[index] = list[index + 1];
%3D
return result;
} catch (Exception e) {
e.printStackTrace();
return null;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdad5a646-4583-4c4b-beba-29e57d936914%2F5368ca6d-ccb5-40bd-bb12-92101512392a%2F47l508l_processed.jpeg&w=3840&q=75)
Transcribed Image Text:private void ensureCapacity() throws Exception {
= list.length
if (numberofEntries >= capacity) {
int capacity
1;
int newCapacity = 2 * capacity;
checkCapacity(newCapacity);
list =
Arrays.copy0f(list, newCapacity + 1);
%3D
private void makeRoom(int newPosition) {
int newIndex =
newPosition;
int lastIndex = number0fEntries;
for (int index = lastIndex; index >= newIndex; index--) {
list[index + 1] = list[index];
private void checkIntegrity() throws Exception {
if(lintegrityOK)
throw new Exception("AList object is corrupt.");
}
@Override
public T remove (int givenPosition) {
// TODO Auto-generated method stub
return null;
@Override
public void clear() {
// TODO Auto-generated method stub
@override
public T replace(int givenPosition, T newEntry) {
// TODO Auto-generated method stub
return null;
@override
public T getEntry(int givenPosition) {
// TODO Auto-generated method stub
return null;
@override
public T[] toArray() {
try {
checkIntegrity();
T[] result = (T[]) new Object[numberofEntries];
for (int index = 0; index < number0fEntries; index++)
result[index] = list[index + 1];
%3D
return result;
} catch (Exception e) {
e.printStackTrace();
return null;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Recommended textbooks for you
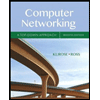
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
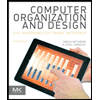
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
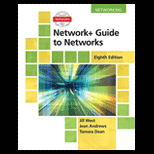
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
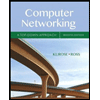
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
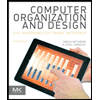
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
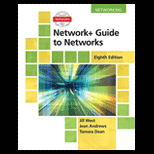
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
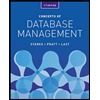
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
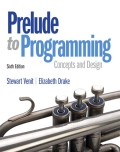
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
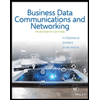
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY