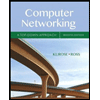
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
![Task 3
def print_table(
values: tuple [float, ...], drag_coeff: float, increments: int, step: float
) -> None:
Parameters:
values (tuple [float, ...]): mass, force, ref_area, density,
init_velocity, lift_velocity, start_position, time_inc
drag_coeff (float): The drag coefficient.
increments (int): The number of drag coefficients displayed.
step (float): The difference between each drag coefficient.
10
11
12
Returns:
13
None
14
15
For this function you need to compute the distance before lift-off for a range of drag coefficients
and then you need to print these results in a table.
The drag coefficient of an aeroplane has a significant impact on the plane's ability to lift-off. If the
drag coefficient is sufficiently high, the plane will not actually be able to generate enough speed to
lift off. In this task you will write a function which will explore this phenomenon.
Example
>>> values - (50000.0, 600000.0, 800.0, 1.0, 0.0, 70.0, 0.0, 0.1)
>>> drag_coeff - 0.015
>>> print_table(values, drag_coeff, 10, 0.03)
...
• Drag coefficient • Runway distance •
0.015
214.218
0.045
224.707
0.075
234.859
0.105
251.633
0.135
267.901
0.165
297.560
0.195
326.334
0.225
367.907
0.255
435.575
0.285
583.499
Hint: You should create the above table by using f-string formatting. Check the appendix for layout
dimensions.](https://content.bartleby.com/qna-images/question/e4190eb8-85d2-4f99-ad74-135ce0e52615/790faa80-7151-45f5-92ee-83ae7f5f1148/nuzewai_thumbnail.png)
Transcribed Image Text:Task 3
def print_table(
values: tuple [float, ...], drag_coeff: float, increments: int, step: float
) -> None:
Parameters:
values (tuple [float, ...]): mass, force, ref_area, density,
init_velocity, lift_velocity, start_position, time_inc
drag_coeff (float): The drag coefficient.
increments (int): The number of drag coefficients displayed.
step (float): The difference between each drag coefficient.
10
11
12
Returns:
13
None
14
15
For this function you need to compute the distance before lift-off for a range of drag coefficients
and then you need to print these results in a table.
The drag coefficient of an aeroplane has a significant impact on the plane's ability to lift-off. If the
drag coefficient is sufficiently high, the plane will not actually be able to generate enough speed to
lift off. In this task you will write a function which will explore this phenomenon.
Example
>>> values - (50000.0, 600000.0, 800.0, 1.0, 0.0, 70.0, 0.0, 0.1)
>>> drag_coeff - 0.015
>>> print_table(values, drag_coeff, 10, 0.03)
...
• Drag coefficient • Runway distance •
0.015
214.218
0.045
224.707
0.075
234.859
0.105
251.633
0.135
267.901
0.165
297.560
0.195
326.334
0.225
367.907
0.255
435.575
0.285
583.499
Hint: You should create the above table by using f-string formatting. Check the appendix for layout
dimensions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- food_wastage_record.hpp class FoodWastageRecord {public:void SetDate(const std::string &date);void SetMeal(const std::string &meal);void SetFoodName(const std::string &food_name);void SetQuantityInOz(double qty_in_oz);void SetWastageReason(const std::string &wastage_reason);void SetDisposalMechanism(const std::string &disposal_mechanism);void SetCost(double cost); std::string Date() const;std::string Meal() const;std::string FoodName() const;double QuantityInOz() const;std::string WastageReason() const;std::string DisposalMechanism() const;double Cost() const; private:std::string date_;std::string meal_;std::string food_name_;double qty_in_oz_;std::string wastage_reason_;std::string disposal_mechanism_;double cost_;}; food_wastage_record.cpp #include "food_wastage_record.h" void FoodWastageRecord::SetDate(const std::string &date) { date_ = date; }void FoodWastageRecord::SetMeal(const std::string &meal) { meal_ = meal; }void…arrow_forwardC language codearrow_forwardIn Python, grades_dict = {'Wally': [87, 96, 70], 'Eva': [100, 87, 90], 'Sam': [94, 77, 90], 'Katie': [100, 81, 82], 'Bob': [83, 65, 85]} plot 5 box plots one for each student within a single graph. Note: Rename the x-axis data to students’ names using: plt.xticks([1, 2, 3, 4 ,5], [’Student Name 1’, ’Student Name 2’, ’Student Name 3’, ’Student Name 4’, ’Student Name 5’]), see Figure in the next page. The values in these two arguments should be retrieved automatically and should work for any number of students not just 5'''arrow_forward
- Unique Values This function will receive a single map parameter known as a_map. a_map will contain a single letter as a string and numbers as values. This function is supposed to search a_map to find all values that appear only once in a_map. The function will create another map named to_ret. For all values that appear once in a_map the function will add the value as a key in to_ret and set the value of the key to the key of the value in a_map (swap the key-value pairs, since a_map contains letters to numbers, to_ret will contain Numbers to Letters). Finally, the function should return to_ret. Signature: public static HashMap<String, Integer> uniqueValues(HashMap<Integer, String> a_map) Example: INPUT: {0=M, 2=M, 3=M, 5=M, 6=n, 7=M, 9=M}OUTPUT: {n=6} INPUT: {0=A, 1=c, 2=c, 4=c, 5=a, 8=Q, 9=c}OUTPUT: {A=0, a=5, Q=8}arrow_forwardData structures return_growing_num_list(max:int) -> list This function will be given a single number, it should return a list of strings of numbers. Each string in the list will only contain a single number repeated an arbitrary amount of times. The number each string will contain will be equal to the current string's index+1. The number in the string should be repeated the same number of times as the string's index+1. Each number in the string should be separated by a space. This list should stop when its size equals the max number specified. Example: max = 3 output = ['1', '2 2', '3 3 3'] max = 4 output = ['1', '2 2', '3 3 3', '4 4 4 4']arrow_forwardPlease help with Java code. Create student class Name Id Marks Create array of 10 student and print all studentsarrow_forward
- 6. sum_highest_five This function takes a list of numbers, finds the five largest numbers in the list, and returns their sum. If the list of numbers contains fewer than five elements, raise a ValueError with whatever error message you like. Sample calls should look like: >>> sum_highest_five([10, 10, 10, 10, 10, 5, -17, 2, 3.1])50>>> sum_highest_five([5])Traceback (most recent call last): File "/usr/lib/python3.8/idlelib/run.py", line 559, in runcode exec(code, self.locals) File "<pyshell#44>", line 1, in <module> File "/homework/final.py", line 102, in sum_highest_five raise ValueError("need at least 5 numbers")ValueError: need at least 5 numbersarrow_forwardvrite a each eler the original assigns the element with 0. Ex: lowerScores = {5, 0, 2, -3} becomes {4, 0, 1, 0}. 406554.2871636.qx3zqy7 1 #include 2 #include 3 using namespace std; 4 5 int main() { 6 7 9 10 11 12 13 14 15 16 17 Run const int SCORES_SIZE = 4; vector lowerScores (SCORES_SIZE); unsigned int i; for (i 0; i > lowerScores.at(i); } /* Your solution goes here */ for (i = 0; i < lowerScores.size(); ++i) { cout << lowerScores.at (i) << " "; nent was greater than 0, and oth wise.arrow_forward@Build ER diagramarrow_forward
- The subscript identifies the of a particular element in the array. O range value O location namearrow_forwardthe statement if (form.element.length>=1) is a form of ___ validationarrow_forwardWhen we use the Group-Object cmdlet, it will create this new property, which displays the number of objects in each grouping: Group of answer choices Number Count Entry Namearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
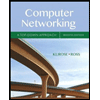
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
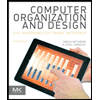
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
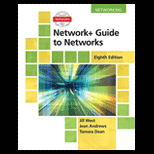
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
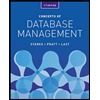
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
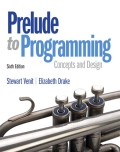
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
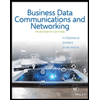
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY