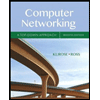
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
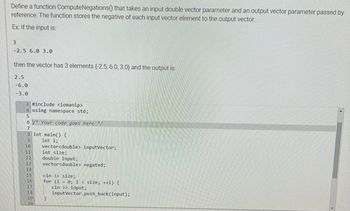
Transcribed Image Text:Define a function ComputeNegations() that takes an input double vector parameter and an output vector parameter passed by
reference. The function stores the negative of each input vector element to the output vector.
Ex: If the input is:
3
-2.5 6.0 3.0
then the vector has 3 elements (-2.5, 6.0, 3.0) and the output is:
2.5
-6.0
-3.0
3 #include <iomanip>
4 using namespace std;
5
6 * Your code goes here */
7
8 int main() {
9
int i;
10
11
12
13
14
15
16
17
18
19
20
vector<double> inputVector;
int size;
double input;
vector<double> negated;
cin >> size;
for (i = 0; i < size; ++i) {
cin >> input;
1}
inputVector.push_back(input);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Similar questions
- struct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward6) While there is a built-in pop_back() method for vectors, there is no built-in pop_front method. Suppose a program needs a pop_front() method that will remove the first element from the vector. For example if the original vector is [1, 2, 3, 4, 5], then after passing in this vector to pop_front() the new resulting vector will be [2, 3, 4, 5]. Which of the options below is the correct implementation of pop_front()? Group of answer choices D-) void pop_front(vector<int> &v){ for(int i=0; i<(v.size()-1); i++)v[i+1] = v[i]; v.pop_back(); } C-) void pop_front(vector<int> &v){ for(int i=0; i<(v.size()-1); i++)v[i] = v[i+1]; v.pop_back(); } B-) void pop_front(vector<int> &v){ for(int i=0; i<v.size(); i++)v[i+1] = v[i]; v.pop_back(); } A-) void pop_front(vector<int> &v){ for(int i=0; i<v.size(); i++)v[i] = v[i+1]; v.pop_back(); } 7) Suppose a program has the following vector: [99, 23, 55, 71, 87, 64, 35, 42] The goal is to…arrow_forwardWrite the lines of code to remove the book at the back of "my_vector". Remember, attempting to remove an element from an empty data structure is a logic error. Include code to avoid that.arrow_forward
- Write a function named addStars that accepts as a parameter a reference to a vector of strings, and modifies the vector by placing a "*" element between elements, as well as at the start and end of the vector. For example, if the vector v contains {"the", "quick", "brown", "fox"}, the call of addStars(v); should modify it to store {"*", "the", "*", "quick", "*", "brown", "*", "fox", "*"}.arrow_forwardHow is a double-valued vector created? How can a vector have a double attached to it? What is the most effective method for calculating a vector's size? How should a vector element be removed?arrow_forward1. Write two ways to display the following matrix 3 4 5 6 ( 13 14 15 16 17 2. 4 3 3 4 Let A 9 6 a) using the columns operator (:) , creat a column vector that contains all the columns of A. b) using the columns operator (:) , creat a column vector that contains all the Rows of A. c) using the columns operator (:),creat a Row vector that contains the 1*t row and 3rd columns of A. d) using the columns operator (:) , creat a column vector that contains all the columns of A. e) using the columns operator (:) , creat a column vector that contains all the columns of A.arrow_forward
- System Requirements: The Full Adder must be implemented as function entitled Full_Adder. Full_Adder will take in 2 vectors of type bool, as input parameters. Full_Adder will return a vector of type bool. The 2 input parameters are the unsigned binary numbers that will be added. The returned vector will be the summation of the 2 vector parameters. The main function will call Full_Adder (directly or indirectly) with different argument lists to demonstrate that the function works with different inputs. Full_Adder must work with any possible vectors of type bool. This includes input vectors of different sizes.arrow_forwardEvery data structure that we use in computer science has its weaknesses and strengthsHaving a full understanding of each will help make us better programmers!For this experiment, let's work with STL vectors and STL dequesFull requirements descriptions are found in the source code file Part 1Work with inserting elements at the front of a vector and a deque (30%) Part 2Work with inserting elements at the back of a vector and a deque (30%) Part 3Work with inserting elements in the middle, and removing elements from, a vector and a deque (40%) Please make sure to put your code specifically where it is asked for, and no where elseDo not modify any of the code you already see in the template file This C++ source code file is required to complete this problemarrow_forwardWrite a C++ program define a vector object and initialize it with 3 values and then define an iterator that points to elements of this object, then ask the user to enter 3 more values and then add them to the previous vector and then print all elements in the vector.arrow_forward
- MATLAB: Create a vector named n which starts at -10 and steps by 1 to end at +10 by using the start:step:stop syntax. Next, generate a vector x1 so that x1 satisfies the mathematical relationship ?ଵ[?]=(0.60). Since n is a vector, one will need to utilize 0.60.^n (i.e., .^ is used in place of ^) to define x1. Redo the work just completed, but for a vector x2 where x2 satisfies the mathematical relationship ?ଶ[?]=(0.85). Repeat the work just completed, but for a vector x3 where x3 satisfies the mathematical relationship ?ଷ[?]=(1.10). In one figure window, plot x1 as a function of n using the stem command. The stem plot must show BLACK (versus the default blue) lines that have filled circles and a line width of 2; refer to the lecture example script file. Further, the horizontal axis label (xlabel) must be n, the title (title) must include your name and the vertical axis label (ylabel) must show ?ଵ[?]=(0.60)(note the subscript and superscript). In addition, turn on the grid (doc grid),…arrow_forwardResize vector countDown to have newSize elements. Populate the vector with integers {newSize, newSize - 1, …, 1}. Ex: If newSize = 3, then countDown = {3, 2, 1}, and the sample program outputs:3 2 1 Go! #include <iostream>#include <vector>using namespace std; int main() { vector<int> countDown(0); int newSize; unsigned int i; cin >> newSize; /* Your solution goes here */ for (i = 0; i < countDown.size(); ++i) { cout << countDown.at(i) << " "; } cout << "Go!" << endl; return 0;}arrow_forwardC++.Write a function, named SecondNegative, that takes a const reference to a vector of doubles, it should return an const_iterator to the element in the vector that is the second value that is negative. If no such element exists, have the iterator point to one past the end.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
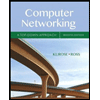
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
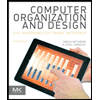
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
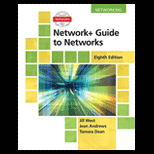
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
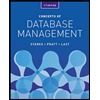
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
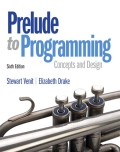
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
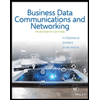
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY