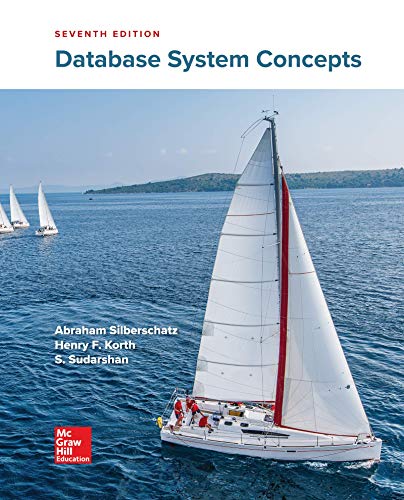
Concept explainers
Do the following in C++.
(1) Create three files to submit.
- ContactNode.h - Class declaration
- ContactNode.cpp - Class definition
- main.cpp - main() function
(2) Build the ContactNode class per the following specifications:
Public member functions
- Build the ContactNode class per the following specifications:
- Public member functions
- InsertAfter()
- GetName() - Acessor
- GetPhoneNumber - Accessor
- GetNext() - Accessor
- PrintContactNode()
Private data members
- string contactName
- string contactPhoneNum
- ContactNode* nextNodePtr
Ex. of PrintContactNode() output:
Name: Roxanne Hughes
Phone number: 443-555-2864
(3) In main(), prompt the user for three contacts and output the user's input. Create three ContactNodes and use the nodes to build a linked list.
Ex:
Person 1
Enter name:
Roxanne Hughes
Enter phone number:
443-555-2864
You entered: Roxanne Hughes, 443-555-2864
Person 2
Enter name: Juan Alberto Jr.
Enter phone number:
410-555-9385
You entered: Juan Alberto Jr., 410-555-9385
Person 3
Enter name:
Rachel Phillips
Enter phone number: 310-555-6610
You entered: Rachel Phillips, 310-555-6610
(4) Output the linked list
Ex:
CONTACT LIST
Name: Roxanne Hughes
Phone number: 443-555-2864
Name: Juan Alberto Jr.
Phone number: 410-555-9385
Name: Rachel Phillips
Phone number: 310-555-6610

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Written in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardGiven this class specification: Class: Student Data members: int ID Write these function definitions: 1. An external definition of a member function that can be a const member function. 2. An external definition for a member function that should not be a const member function.arrow_forwardProgram: Circle.h and newCircle.cpp 1: Create a class specification file, Circle.h, that contains the Circle class declaration 2: Create a class implementation file, Circle.cpp, that contains the member function definition, with a constructor that can accept arguments 3. Based on Program 13-8 in the textbook, create a program, newCircle.cpp, that generate two instances of the Circle class, pizza1 and pizza2, which can have different size (radius). Hint: Use the example source code in “Rectangle Version 4.zip” to create a multi-file project. Step 1: In Dev-C++, go to file à New à Project to create an empty project Setp 2: Go to ProjectàAdd to project, add new cpp files (e.g. Rectangle.cpp and Pr13-8.cpp files). Step 3: Compile and run Problem 2: Read the example source code in “HW2Problem2.zip” and modify it as follows: 1. Modify the code and create three rectangle objects, box1, box2, and box3. 2. Modify the code and create some static member function(s) that can count…arrow_forward
- C++ Assignment---This is an unfinished code please complete it. Make changes if necessary. Upvote for finished working code. Objectives • To apply knowledge of classes and objects Instructions Implement all of the following, each in a separate Visual Studio project (and/or solution). 1. Define a class called CounterType to implement a simple counter. The class must have a private field (data member) counter of type int. You must also provide methods (member functions), including the following: a. A constructor to set the counter to the value specified by the user b. A constructor to set the counter to 0 c. A method to allow the user to set the counter to a value they specify d. A method to increment the counter by 1 e. A method to decrement the counter by 1 Your code must ensure the value of counter is never negative. If the user calls a method to attempt to make the counter negative, set the counter to 0 and print out an error to the user (e.g., “The counter must not be negative.”) Do…arrow_forwardDefine member access specifiers.arrow_forwardWhen a class contains a data member that is a pointer to dynamically-allocated storage, which of the following member functions should be implemented? All three of the member functions listed below should be implemented. The copy assignment operator. The copy constructor. The destructor.arrow_forward
- please assist me C++ language you are the owner of a pet store and you want to create a database of the different types of pets you sell you will create a base class called Pet and will include the following member variables: gender size (small, medium, or large) trained (yes or no) age long haired (yes or no) create two constructors default will initialize member variable with blanks or zeros second constructor will assign parameter values to each variable create your mutator functions create your accessor functions and save your Pet.h file create a new header file for dogs: dog.h it will be a derived class of Pet.h it will inherit the public functions of Pet.h it will have its own member variable breed (ie: beagle, collie, etc.) create the .cpp file, pet store create two instances of dog use the default constructor for the first dog use the second constructor for the second dog display both dog's data labeling each line report should look something like this…arrow_forwardUsing C# language: Programming PLO-2 Measured: Design, implement, and evaluate computer solutions utilizing structured and object-oriented programming methodologies. Design a class named Contractor. The class should keep the following information: Contractor name Contractor number Contractor start date Write one or more constructors and the appropriate accessor and mutator functions for the class.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
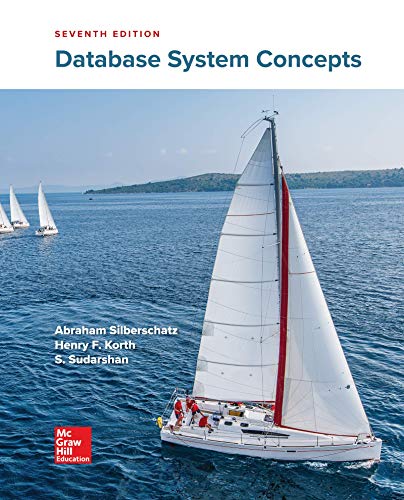
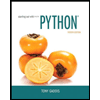
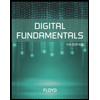
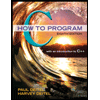
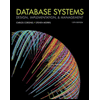
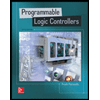