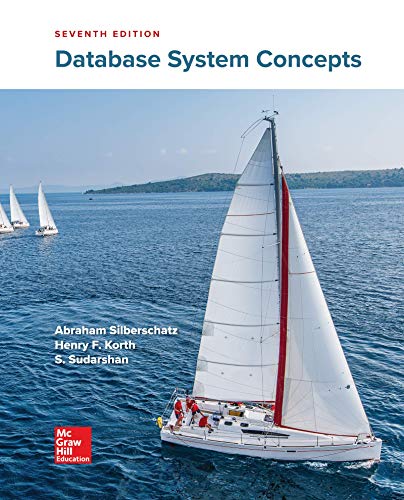
Do the following in Java and provided source code with screenshots of correct output!
1. Create an interface Vehicle that contains three void, abstract methods named Start, Stop and Change Speed.
2 .Create an Airplane class (not abstract) that uses the Vehicle interface in Q1. The code for all methods should print simple messages to the screen using System.out.println(). Add an integer speed variable that is changed using the Change Speed method. ChangeSpeed adds 5 to the speed each time it is called. Create a default constructor that sets the initial speed to 0. Don't create other constructors or any setter/getter methods.
3. Create an abstract class Car that also uses the Vehicle interface in Q1 and has three variables, Year, Make, and Speed. Include the following:
- One constructor that accepts the car's year and make as arguments. The constructor should also assign 0 to the speed variable. Do not create other constructors.
- Create a void method sound but leave it unimplemented.
- Create a variable Count that keeps track of the number of car instances. When each new car is created, the counter should increment by 1.
- Create a getCount method that returns the number of car instances held in the Count variable.
- Don't implement the Vehicle interface methods. Don't implement any setters/getters.
4.Create a class SportsCar that inherits from the Car class in Q3. Include the following:
- A variable Roof that holds the type of roof (Ex: Convertible, Hard-Top, Soft-Top)
- A variable Doors that holds the car's number of doors (Ex: 2, 4)
- Implement the Change Speed method to add 20 to the speed each time it is called.
- Add exception handling to the Change Speed method to keep the speed under 65.
- Implement the sound method to print, "broooom," to the screen.
- Create one constructor that accepts the car's roof, doors, year and make as arguments and assigns the values appropriately. Do not create any other constructors.
- The interface methods should print simple messages to the screen using System.out.println().
5.Create a driver class Playground that contains the function, public static void main(String[] args) {}.
- Create 2 SportsCar and 2 Airplane instances using their constructors.
- Add all 4 instances into a single array called, "elements."
- Create a loop that examines each element in the array, "elements." If the elements item is a SportsCar, run the sound method and if the item is an Aeroplane, run it's ChangeSpeed method.
- Run the getCount() method (created in Q3) to display how many total cars were created.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- In JavaScript, how are an object and an interface related to each other? a. The object’s interface consists of the code and data needed for that object. b. An object’s interface is analogous to a pocket calculator’s inner workings. c. Built-in JavaScript objects do not require an interface, but custom objects do. d. An interface allows another program to access an object’s properties and methods.arrow_forwardRefer to the following interface: Provide two examples (screenshots) that in many ways could improve the above or similar interface using the principles of direct manipulation. Explain the relevant improvements as well. (Examples might have different object, labels and organization)arrow_forwardDraw the UML diagram for the class. Implement the class. Write a test program that creates an Account object with an account ID of 1122, a balance of $20,000, and an annual interest rate of 4.5%. Use the withdraw method to withdraw $2,500, use the deposit method to deposit $3,000, and print the balance, the monthly interest, and the date when this account was created.arrow_forward
- Create a subclass named Square from the superclass GeometricObject. Create an instance variable named width with initial value 0. Create a no-arg constructor. Create the another constructor, which has one parameter named wid. Assign the wid to the instance variable named width. Create the getWidth() method, the setWidth() method. Override the getArea() method. Override the getPerimeter() method. Create a test class named Test to create an instance with wid= 6 from Square class. Call the getArea() and getPermeter() methods to print out the area and perimeter of the square with width=6.arrow_forwardplease answer with outputarrow_forwardUSING JAVA Write these: An interface called Shape that has two methods: area and perimeter. A class Rectangle that implements Shape and has a length and a width. A class Circle that implements Shape and has a radius. For each class, write the needed constructors, getters, setters, and a toString method that stringifies the instance variables in a readable format. Supply a test program that instantiates an instance of each class and exercises each method defined for the class.arrow_forward
- Java: According to this diagram, what are the methods that the StagBeetle class can use? (Select all that apply) Group of answer choices: A: hornSize B: fly() C: emitPheromone() D: eat()arrow_forwardDesign and implement a set of classes that define the employees of a hospital: doctor, nurse, administrator, surgeon, receptionist, janitor, and so on. Include methods in each class that are named according to the services provided by that person and that print an appropriate message. Create a main driver class to instantiate and exercise several of the classes.arrow_forwardwrite a program using java Create a class named “Rectangle” having length and width as attribute. There should be behaviors to set the values of length and width (individually and collectively), and get their values individually. There should also be behavior to display the perimeters and area of the rectangle. Create another class named as Main which has two objects of Rectangle (r1, r2) having length (3.2, 20) and width (4.5, 36) respectively. Also display the length, width, perimeter and area of both rectangles.arrow_forward
- In Java!!!!!This is a multi-part question. Consider the following UML diagram on attached image: 1.Write Java classes corresponding to the UML diagram. 2. Write getters and setters for all classes 3. Write non-default constructors for each class that initializes all instance variables to values passed as arguments to the constructor 4. Write toString method for each class 5. Modify Vehicle class to maintain inventory (list of all vehicles created) and print the list. What variable(s) you need to add? What additional method(s) you need? What else needs to be modified in the Vehicle class? Do you need to modify any of the descendant classes and explain your answer (if not: why not; if yes, describe what changes). Vehicle {Abstract} manufacturer:String - color:String Car Truck TwoWheeler {Abstract} -numberOfDoors:int -numberOfAxels:int -passengerSeats:int -fuelType:String -fuelLevel:int -fuelType:String -numberOfSeats:int -fuelLevel:int Truck Motorcycle -numberOfAxels:int -fuelType:String…arrow_forwardPlease write the code in Java eclipse.arrow_forwardI need some help figuring out how to do this in Java pleasearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
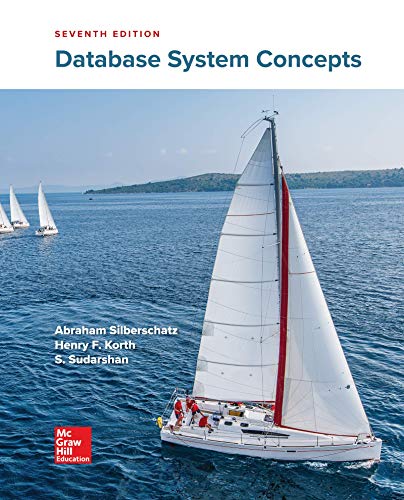
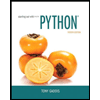
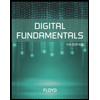
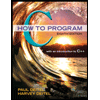
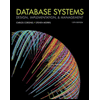
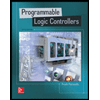