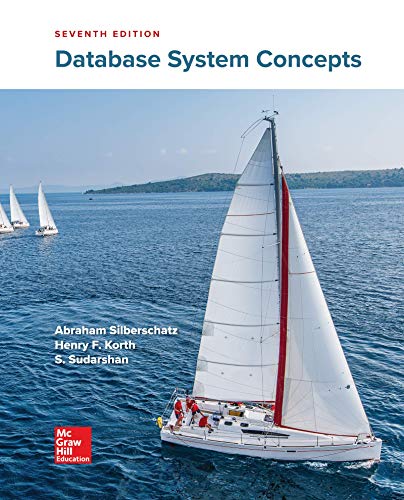

Do the following lab by one dimensional array(not vector) Write a C++ program that: part a) for 3 students find the average of a student by asking him how many grades do you have. To answer to this question, determine the size of the array(number of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Part b) Find the maximum and minimum grades for each student. Part c) Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98
Step by stepSolved in 4 steps with 2 images

Do the following lab by one dimensional array(not vector) Write a C++ program that: part a) for 3 students find the average of a student by asking him how many grades do you have. To answer to this question, determine the size of the array(number of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Part b) Find the maximum and minimum grades for each student. Part c) Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98
Do the following lab by one dimensional array(not vector) Write a C++ program that: part a) for 3 students find the average of a student by asking him how many grades do you have. To answer to this question, determine the size of the array(number of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Part b) Find the maximum and minimum grades for each student. Part c) Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98
- code for this in C: Create an array of 15 integers. Add all elements located at ODD index positions and sum also all numbers located at EVEN(Assume zero index is even) index positions in the linear array. The user will be the one to input each item in the array of 15 elements. Display also the inputted integers in the array. Given the sample layout w/ test data below:arrow_forwardWrite the following function that tests whether the array has four consecutive numbers with the same value. bool isConsecutiveFour(const int values[], int size) Write a test program that prompts the user to enter a series of integers and displays if the series contains four consecutive numbers with the same value. Your program should first prompt the user to enter the input size—i.e., the number of values in the series. Assume the maximum number of values is 80. Here are sample runs: Enter the number of values: 8 Enter the values: 3 4 5 5 5 5 4 5 The list has consecutive fours Enter the number of values: 9 Enter the values: 3 4 5 5 6 5 5 4 5 The list has no consecutive foursarrow_forward► Write a C++ program to record sales of products sold at various stores across the country. Your program must cater for seven (7) products and five (5) stores/locations in a two-dimensional array. Your program must be: 1) Allow data entry. 2) Find the product that has best sales in each store. 3) Find the product that has worst sales in each store. 4) Determine total sales for each product in all the stores. 5) Determine total sales for each store. 6) Determine the best performing store. 7) Determine the best performing product and say which product it is and in which store did it make historic sales.arrow_forward
- Display Array values Write a program that lets the user enter ten values into an array. Then pass the array, the size of the array, and the number, num to a function called displayArrayValues(). The function should then display all the numbers in the array larger than n. Write a program using C++.arrow_forwardCorrect my mistake in my C++ code please! Here is the question and my code is below as well. (Duplicate Elimination with array) Use a one-dimensional array to solve the following problem. Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, validate it and store it in the array only if it isn't a duplicate of a number already read. After reading all the values, display only the unique values that the user entered. Provide for the 'worst case' in which all 20 numbers are different. Use the smallest possible array to solve this problem. Code; #include <iostream> using namespace std; int main(){ int arr[100]={0};int temp,i;for( i=0;i<20;i++){ cout<<"Enter an Integer : "; cin>>temp; arr[temp]++;}for( i=0;i<100;i++) if(arr[i]==1) cout<<i<<" "; return 0;}arrow_forwardUSING C lang: Create a simple Tic Tac Toe game designed for one player (against computer). you will need to create arrays and functions to complete this program. The first screen should ask the user for his name, print a welcome message, and display an empty 3x3 Tic Tac Toe board. Then, the program should ask the user to put the coordinates of his move. once the user enters the coordinates, the program should respond accordingly with a print of the user's move and the computer's move. this should go on until a winning pattern is found. Key steps: Game board is designed, user inputs taken and marked on the board, validate the inputs that no two inputs at the same place and out of range, check the winner/loser and complete the game.arrow_forward
- C languagearrow_forwardC++ Do the following lab by an array(not vector) for 3 students find the average of a student by asking him how many grades do you have. The answer to this question, determine the size of the array(list of grades) and then ask the user to enter grades(the number of the grades is equal to the size of the array) then calculate the average for each student. Hence, you will calculate 3 averages. to calculate the average, use the array accumulator. Also when you get each grade validate it. validation for grade means that you check whether a grade is between 0 and 100. if the grade is negative or a grade is more than 100 then it is not valid and you have to ask the user to enter a grade again. Find the maximum and minimum grades for each student. Print the sorted list of the grades for each student. example: if grades user entered are: 60 78 98 23 45 then sorted list is:23 45 60 78 98arrow_forwardprogramming used: javaarrow_forward
- Summary In this lab, you use what you have learned about searching an array to find an exact match to complete a partially prewritten C++ program. The program uses an array that contains valid names for 10 cities in Michigan. You ask the user to enter a city name; your program then searches the array for that city name. If it is not found, the program should print a message that informs the user the city name is not found in the list of valid cities in Michigan. The file provided for this lab includes the input statements and the necessary variable declarations. You need to use a loop to examine all the items in the array and test for a match. You also need to set a flag if there is a match and then test the flag variable to determine if you should print the the Not a city in Michigan. message. Comments in the code tell you where to write your statements. You can use the previous Mail Order program as a guide. Instructions Ensure the provided code file named MichiganCities.cpp is…arrow_forwardWrite a C++ program that uses a 1D array to store 25 students' computer programming grades (total grade 100). The program should not accept incorrect or invalid grades, and the user should be able to enter marks one at a time. Once the user has completed the entry, the program should output the following. a) Maximum grade b) The average grade (sum of all grades/total number of students) c) Minimum grade Write the C++ code in the space provided (Full marks are awarded for a complete solution.)arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
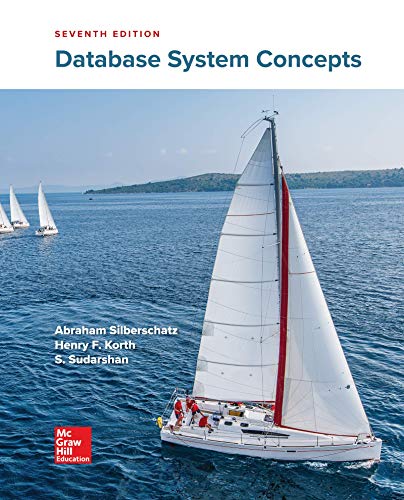
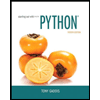
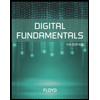
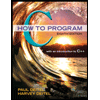
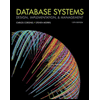
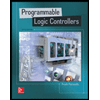