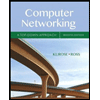
Please use Python for this question. Make sure the code is formatted properly.
Write a function orderPizza that allows the user input to build a pizza. It then prints a thank you message, the cost of the pizza and then returns the Pizza that was built.
Output for this question:
>>> orderPizza()==Pizza('M',{'garlic', 'onion', 'mushroom'})
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $14.299999999999999
True
>>> orderPizza()==Pizza('L',{'calamari', 'garlic'})
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $16.65
True
>>> orderPizza()==Pizza('S',set())
Welcome to Python Pizza!
What size pizza would you like (S,M,L): Type topping to add (or Enter to quit): Thanks for ordering!
Your pizza costs $6.25
True
#stdin back #
put stdin back to original, again, shouldnt cause error
>>> sys.stdin = si # return stdin

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- i am after a python Graphical User Interface (GUI) for the question shown in the image: the GUI must implement the following code: #creating function def account(): # taking input for amount deposited 'p' p = int(input('Enter number of deposited amount:$')) # taking input for stated interest rate 'r' r = float(input(' Enter stated interest rate: ')) # taking input for coumpounding interest 'm' m = int(input(' Enter compounding interest: ')) # Now calculating account balance account_balance = p*(1+(r/m))**m print(account_balance) # Now calculating Annual percentage yield (APY) APY = (1+(r/m)**m)-1 # if in case account balance comes greater than 1000$ than it will calculate APY if account_balance > 1000: print(APY) account() as well as it must have all these: Use the Grid element• Add Label elements throughout your GUI.• Use the Background Colour property.• Allow a user to select choices from an either list box, drop-down menu, or scroll-down menuelement. Allow a user to input…arrow_forwardHow would I fill in the blank for this question in pythonarrow_forwardVoid functions do not return any value when they are called.True or falsearrow_forward
- Write a function named product that takes two int parameters and returns the product of those two integers. Here is an example: int n = product(7, 5); // n is 35 We are providing a test framework that, if you wish, you may use with a compiler to test your function. What you will submit as your answer to this problem is only the product function. Do not submit things like the #include lines or a main routine. If we take the code you write in the space below and insert it into the program, the resulting program must build without compilation errors (mere warnings are allowed). You do not need to write comments in the code; we will not look at any comments. Here is the test framework that you may copy and paste into a C++ source file. For your own testing purposes, you might add additional tests in the main routine, although you won't be turning them in, of course. #include <iostream> #include <cassert> using namespace std; // SUBMIT AS YOUR ANSWER ONLY THE CODE BETWEEN THIS…arrow_forwardin pythonarrow_forwardPython (this is not graded this is practice work that is not graded) write and test a function which takes two dates (month, day) list arguments.When the function is called with the two arguments, it returns the number of days betweenthose two dates. Print the returned value. It is assumed that the first date occurs first. Forinstance, if the users calls the function with FUNCTION([12, 10], [12, 20]) as arguments, thereturned value is 10 days. However, if FUNCTION([12, 20], [12, 10]) is called, the returnedvalue is -10 days.Assume February has 28 days.Test data: [10, 30], [5, 20] [1, 30], [5, 25] [5, 25], [1, 30] [1, 1], [12, 31]arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
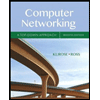
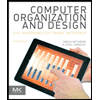
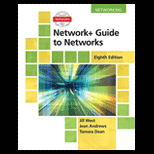
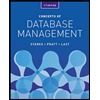
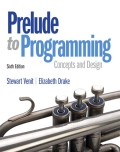
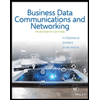