ex2_main.cpp #include #include #include "lab2_ex2.h" #define FILENAME "statement11.txt" int main() { // 1. Acquire the text from file char *text = read_text_file(FILENAME); // Note: Caller should free the memory allocated printf("%s\n", text); // 2. Compute the readability and display the grade level float readability = get_readability(text); printf("Readability index = %f\n", readability); display_level(readability); // 3. Free resources, e.g. allocated memory by read_text_file() function free(text); return 0; } ... lab2_ex2.cpp /* EDIT THIS FILE TO SOLVE THE LABORATORY EXERCISES */ #include #include #include #include #include #include "lab2_ex2.h" /* Assume that a letter is any lowercase character from a to z or any uppercase character from A to Z */ int alpanumeric_count(const char *text) { int count = 0; /* YOUR CODE HERE!!! */ return count; } /* Assume that any sequence of characters separated by spaces or new line or tab , etc. should count as a word. ' ' space '\t' horizontal tab '\n' newline '\r' carriage return */ int word_count(const char *text) { int count = 0; /* YOUR CODE HERE!!! */ return count; } /* Assume that any occurrence of a period, exclamation point, or question mark indicates the end of a sentence. */ int sentence_count(const char *text) { int count = 0; /* YOUR CODE HERE!!! */ return count; } /* This function computes the readability according to the formula: Coleman–Liau Index Formula CLI = 0.0588 * L - 0.296 * S - 15.8 https://en.wikipedia.org/wiki/Coleman%E2%80%93Liau_index The Coleman–Liau index is a readability test designed by Meri Coleman and T. L. Liau to gauge the understandability of a text. Like the Flesch–Kincaid Grade Level, Gunning fog index, SMOG index, and Automated Readability Index, its output approximates the U.S. grade level thought necessary to comprehend the text. Like the ARI but unlike most of the other indices, Coleman–Liau relies on characters instead of syllables per word. Although opinion varies on its accuracy as compared to the syllable/word and complex word indices, characters are more readily and accurately counted by computer programs than are syllables. The Coleman–Liau index was designed to be easily calculated mechanically from samples of hard-copy text. Unlike syllable-based readability indices, it does not require that the character content of words be analyzed, only their length in characters. Therefore, it could be used in conjunction with theoretically simple mechanical scanners that would only need to recognize character, word, and sentence boundaries, */ float get_readability(const char *text) { /* YOUR CODE HERE!!! */ return 0.0f; }
...
ex2_main.cpp
#include <stdio.h>
#include <stdlib.h>
#include "lab2_ex2.h"
#define FILENAME "statement11.txt"
int main()
{
// 1. Acquire the text from file
char *text = read_text_file(FILENAME); // Note: Caller should free the memory allocated
printf("%s\n", text);
// 2. Compute the readability and display the grade level
float readability = get_readability(text);
printf("Readability index = %f\n", readability);
display_level(readability);
// 3. Free resources, e.g. allocated memory by read_text_file() function
free(text);
return 0;
}
...
lab2_ex2.cpp
/*
EDIT THIS FILE TO SOLVE THE LABORATORY EXERCISES
*/
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#include <math.h>
#include <stdlib.h>
#include "lab2_ex2.h"
/*
Assume that a letter is any lowercase character from a to z
or any uppercase character from A to Z
*/
int alpanumeric_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
Assume that any sequence of characters separated by spaces or new line or tab , etc. should count as a word.
' ' space
'\t' horizontal tab
'\n' newline
'\r' carriage return
*/
int word_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
Assume that any occurrence of a period, exclamation point, or
question mark indicates the end of a sentence.
*/
int sentence_count(const char *text)
{
int count = 0;
/* YOUR CODE HERE!!! */
return count;
}
/*
This function computes the readability according to the formula:
Coleman–Liau Index Formula
CLI = 0.0588 * L - 0.296 * S - 15.8
https://en.wikipedia.org/wiki/Coleman%E2%80%93Liau_index
The Coleman–Liau index is a readability test designed by Meri Coleman and T. L. Liau to
gauge the understandability of a text. Like the Flesch–Kincaid Grade Level, Gunning fog index,
SMOG index, and Automated Readability Index, its output approximates the U.S. grade level thought
necessary to comprehend the text.
Like the ARI but unlike most of the other indices, Coleman–Liau relies on characters instead of
syllables per word. Although opinion varies on its accuracy as compared to the syllable/word and
complex word indices, characters are more readily and accurately counted by computer programs than
are syllables.
The Coleman–Liau index was designed to be easily calculated mechanically from samples of hard-copy text.
Unlike syllable-based readability indices, it does not require that the character content of words be
analyzed, only their length in characters. Therefore, it could be used in conjunction with theoretically
simple
*/
float get_readability(const char *text)
{
/* YOUR CODE HERE!!! */
return 0.0f;
}
/*
This function accepts readability and displays the readability grade level for the text
*/
void display_level(float readability)
{
int level = round(readability);
if (level < 1)
{
printf("Before Grade level 1\n");
}
else if (level > 16)
{
printf("Grade level 16+\n");
}
else
{
printf("Grade level %i\n", level);
}
}
/*
This function reads in a text file given the filename
WARN: The caller needs to free the memory to avoid leaks
*/
char *read_text_file(const char *filename)
{
FILE *fp = fopen(filename, "r");
if (fp == NULL)
{
printf("Error: could not open file %s", filename);
return NULL;
}
fseek(fp, 0, SEEK_END);
long length = ftell(fp);
fseek(fp, 0, SEEK_SET);
char *buffer = (char *)malloc(length + 1);
buffer[length] = '\0';
fread(buffer, 1, length, fp);
fclose(fp);
return buffer;
}
...
lab2_ex2.h
#ifndef lab2_ex2_h
#define lab2_ex2_h
int sentence_count(const char *);
int alpanumeric_count(const char *);
int word_count(const char *);
float get_readability(const char *);
void display_level(float);
char *read_text_file(const char *);
...
VERY IMPORTANT NOTE: Modify/edit/fix only lab2_ex1.cpp to make the program work.
i'm using VS Code on Windows

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

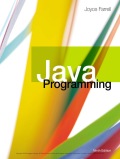
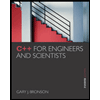
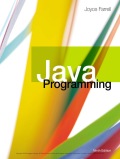
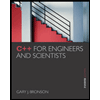
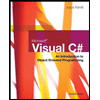