Extend the patient class to include the following data members and methods i.e., INHERITANCE. DEFINE AND IMPLEMENT (write the code for) the extended class. Class will be a name of your choice. Member variable medical record number (i.e., the identifier of the last hospital providing care). Include member method includes, prototypes (declaration) and code (definition): all constructor(s), set medical record number and print to extend the base class patient. Create an object of the inherited class that sets all member variables to values of your choice, i.e., use the extended class as you would in a test/client program. You do not need to write any other code in the test/client
C++ language
patient.h
/*preventing multiple inclusion of header files using
#ifndef and #define directives */
#ifndef PATIENT_H
#define PATIENT_H
#include<iostream>
using namespace std;
class patient
{
//declaring instance variables
private:
string firstName;
string lastName;
int id;
public:
//default constructor declaration
patient();
//parameterized constructor declaration
patient(string fName,string lName,int pId);
//print() function declaration
void print();
//setter functions declaration
void setFirstName(string fName);
void setLastName(string lName);
void setId(int pId);
};
#endif
patient class implementation
patient.cpp
#include "patient.h"
//default constructor implementation
patient::patient()
{
firstName="";
lastName="";
id=0;
}
//parameterized constructor implementation
patient::patient(string fName,string lName,int pId)
{
firstName=fName;
lastName=lName;
id=pId;
}
//print function implementation
void patient:: print()
{
cout<<"First Name: "<<firstName<<endl;
cout<<"Second Name: "<<lastName<<endl;
cout<<"ID: "<<id<<endl;
}
//setter method to set firstName implementation
void patient::setFirstName(string fName)
{
firstName=fName;
}
//setter method to set lastName implementation
void patient::setLastName(string lName)
{
lastName=lName;
}
//setter method to set id implementation
void patient::setId(int pId)
{
id=pId;
}
- Extend the patient class to include the following data members and methods i.e., INHERITANCE. DEFINE AND IMPLEMENT (write the code for) the extended class. Class will be a name of your choice.
- Member variable medical record number (i.e., the identifier of the last hospital providing care).
- Include member method includes, prototypes (declaration) and code (definition): all constructor(s), set medical record number and print to extend the base class patient.
- Create an object of the inherited class that sets all member variables to values of your choice, i.e., use the extended class as you would in a test/client program. You do not need to write any other code in the test/client

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

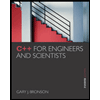
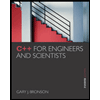