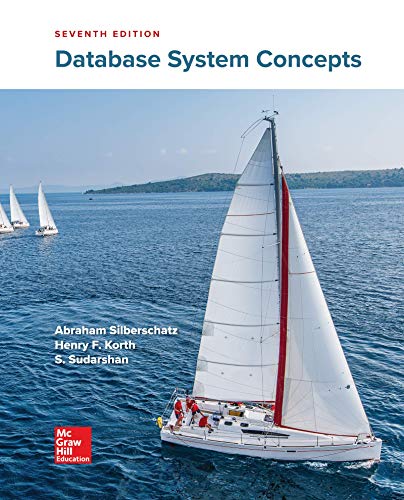
Fill the insertCommas method to recursively construct and return a string representation of the parameter num, including commas for every third decimal place. Ex. 157992546 >>>>> 157,992,546
import java.util.*;
public class NumbersWithCommas{
public static String insertCommas(int num){
}
public static void main(String[] args){
int numTests =(int)(Math.random() * 6) + 5;
for (int i = 0; i < numTests; i++){
int num = (int)(Math.random() * Integer.MAX_VALUE); System.out.println(num + " >>>>> " + insertCommas(num));
}
System.out.println(1000000 + " >>>>> " + insertCommas(1000000)); System.out.println(1001020 + " >>>>> " + insertCommas(1001020)); System.out.println(561010002 + " >>>>> " + insertCommas(561010002));
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- public class Main { static int findPosSum(int A[], int N) { if (N <= 0) return 0; { if(A[N-1]>0) return (findPosSum(A, N - 1) + A[N - 1]); else return findPosSum(A, N - 1); } } public static void main(String[] args) { int demo[] = { 11, -22, 33, -4, 25,12 }; System.out.println(findPosSum(demo, demo.length)); } } Consider the recursive function you wrote in the previous problem. Suppose the initial call to the function has an array of N elements, how many recursive calls will be made? How many statements are executed in each call? What is the total number of statements executed for all recursive calls? What is the big O for this function?arrow_forwardThe following recursive method called z is created. This method accepts two parameters: A string s, and an integer index The code in the method is: if (index == s.length()) return ""; <------ base case if(index % 2 == 0) return ""+ s.charAt(index) + z(s,index+1); <---- recursive call else return z(s,index+1); <----- recursive call What would be the output with the call: z("javajavajava",0);arrow_forwardCorrect my codes in java // Arraysimport java.util.Scanner;public class Assignment1 {public static void main (String args[]){Scanner sc=new Scanner (System.in);System.out.println("Enter mark of student");int n=sc.nextInt();int a[]=new int [n];int i;for(i=0;i<n;i++){System.out.println("Total marks of student in smster");a[i]=sc.nextInt();}int sum=0;for(i=0;i<n;i++){sum=sum+a[i];}System.out.println("Total marks is :");for (i=0;i<n;i++);{System.out.println(a[i]);}System.out.println();}}arrow_forward
- preLabC.java 1 import java.util.Random; 2 import java.util.StringJoiner; 3 4- public class preLabC { 5 6- 7 8 9 10 11 12 13 14 15 16 17 18 19 20 } public static String myMethod(MathVector inputVector) { String vectorStringValue = new String(); // empty String object System.out.println("here is the contents object, inputVector: + inputVector); // get a String out of that MathVector object. Do it here. On the next line. // return the double value here. return vectorStringValue; "1 ▸ Compilation Description A MathVector object will be passed to your method. Return its contents as a String. If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector object as a String. This makes it useful for displaying to the user. /** * Returns a String representation of this vector. The String should be in the format "[1, 2, 3]" * * @return a String representation of this vector * @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method. */…arrow_forwardpublic class arrayOutput ( public static void main (String [] args) { final int NUM ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; } Type the program's output userVals [0] = 2; userVals [1] = 6; userVals [2] = 8; for (i = userVals.length - 1; i >= 0; −−1) { System.out.println(userVals [1]); } C.C. ? ? ??arrow_forwardin java Describe the definition of recursive function. oBase case(s)oRecursive case(s)2. Write the code part of code below public class ReverseArray { public static void main(String[] args) { // TODO Auto-generated method stub public static void reverseArray(int[] data,int low, int high ) { if(low < high) { int temp = data[low]; data[low] = data[high]; data[high] = temp; reverseArray(data, low + 1, high -1); } }arrow_forward
- Write a statement that calls the recursive method backwardsAlphabet() with parameter starting Letter. 789GHA 10 11 12 13 } TENN 14 15 16 17 18 19 20 21 22 } 23 } } else { } System.out.print(currLetter + "); backwardsAlphabet((char) (currLetter - 1)); public static void main (String[] args) { Scanner scnr = new Scanner(System.in); char startingLetter; startingLetter = scnr.next().charAt(0); /* Your solution goes here */ ▶arrow_forwardimport java.util.* ; public class Averager { public static void main(String[] args) { //a two dimensional array int[][] a = {{5, 9, 3, 2, 14}, {77, 44, 22, 15, 99}, {14, 2, 3, 9, 5}, {88, 15, 17, 121, 33}} ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average = 29.85") ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; System.out.println("EXPECTED:") ; System.out.println("Average of evens = 26.57") ; Random random = new Random(1) ; a = new int[100][1] ; for (int i = 0 ; i < 100 ; i++) a[i][0] = random.nextInt(1000) ; System.out.println("For an array of random values in range 0 to 999:") ; System.out.printf("Average = %.2f\n", average(a)) ; System.out.printf("Average of evens = %.2f\n", averageEvens(a)) ; } /** Find the average of all elements of a two-dimensional array @param aa the two…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
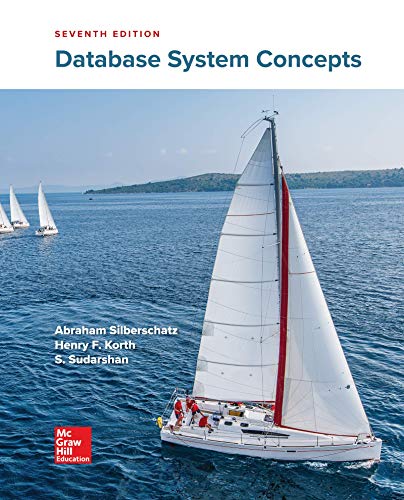
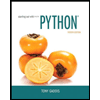
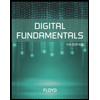
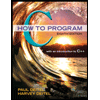
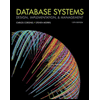
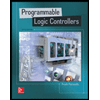