Focus on: Basic list operations, methods, use of functions, and good programming style. Program should be in basic python. Part 1. Write a program that does the following: 1. Create a list of length N where N is a randomly selected integer between 10 and 20 and whose elements are randomly selected integers between 0 and 19. 2. Print out the list. 3. Create a copy of the list. Sort the copy into decreasing order and print it.
Focus on: Basic list operations, methods, use of functions, and good programming style. Program should be in basic python.
Part 1. Write a program that does the following:
1. Create a list of length N where N is a randomly selected integer between 10 and 20 and
whose elements are randomly selected integers between 0 and 19.
2. Print out the list.
3. Create a copy of the list. Sort the copy into decreasing order and print it.
4. Report the distinct elements in the list
Before continuing with the next two parts, add a function user_input(lo, hi) to your
program. It should ask the user for an integer between lo and hi and return the value. Use
appropriate error handling and input validation.
Part 2.
Write a function named how_many(a_list, a_num) that that expects a list of numbers
and a number as arguments. It returns how many times the number is found in the list.
If the number is not in the list, return 0. For example
how_many( [12, 15, 16, 4, 10, 3, 5, 7, 9, 15], 15 )
returns 2
And how_many( [12, 15, 16, 4, 10, 3, 5, 7, 9, 15], 11 )
returns 0
Ask the user for an integer between 0 and 19. If the number is in the list, report how
many times it occurs.
Part 3.
Write a function named find_bigger(a_list, a_num) that expects as arguments a list of
numbers and a number. It should return a new list consisting of all the numbers in the list
that are greater than the number. For example
find_bigger( [12, 15, 16, 4, 10, 3, 5, 7, 9, 15], 11 )
returns [12, 15, 16, 15]
And find_bigger( [12, 15, 16, 4, 10, 3, 5, 7, 9, 15], 15 )
returns [16]
Ask the user for an integer between 0 and 19 and then report how many of the items in the
list are greater than that number.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

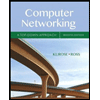
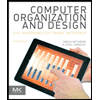
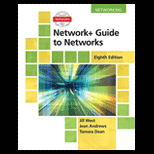
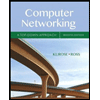
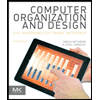
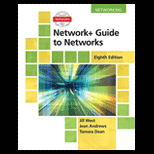
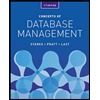
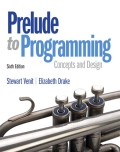
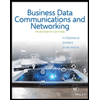