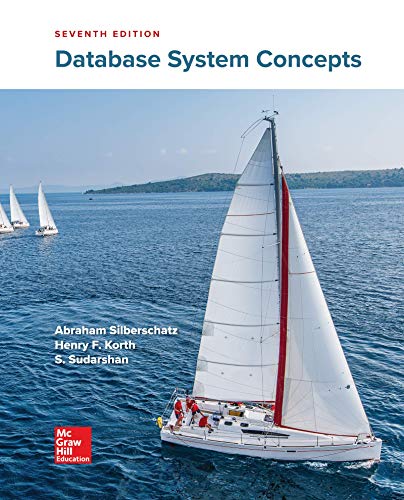
- For simple usage of GUIs, why would the source codes (shown below) not work?
- The input/output objects have not been properly handled.
- The data type of the methods has been defined in a wrong way.
- The necessary parameters in the methods have not been assigned properly.
- The flow of execution has been applied in a wrong way.
import javax.swing.JOptionPane;
public class Palindrome
{
public static void main(String[] args) //main method
{
long num1, num2; //variables to store the numbers
String inputStr, outputStr; // strings to receive from the user
// and display
inputStr=JOptionPane.showInputDialog("Enter the number: ");
num1 = Long.parseLong(inputStr); // change the string to
num2 = num1; // numbers
if (num1 <= 0)
{
num1 = - num1; //if the numbers are negative, change the sign
inputStr = inputStr.valueOf(num1);//change the inputStr value
}
if (isPalindrome(inputStr))
outputStr = num2 + " is a palindrome";
else
outputStr = num2 + " is not a palindrome";
JOptionPane.showMessageDialog(null, outputStr,"Palindrome Program",JOptionPane.INFORMATION_MESSAGE);
System.exit(0);
}
public static void isPalindrome(String str) //call this method
{
int len = str.length();
int i, j;
j = len - 1;
for (i = 0; i <= (len - 1) / 2; i++)
{
if (str.charAt(i) != str.charAt(j))
return 0;
j--;
}
return 1;
}
}
- Which of following is not true about using Java applets?
- A Java applet is a program that is intended to be transported over the Web and executed using a web browser.
- While a Java application is a stand-alone program with a main method, an Java applet doesn't have a main method.
- The paint method is manually executed regardless of the Java applet’s contents.
- The paint method also accepts a parameter that is an object of the Graphics class.
- To draw an oval object like this (shown below), how should we specify the method parameters (like coordinates and sizes)?
11
- drawOval (50, 80, 175, 20);
- drawOval (20, 175, 80, 50);
- drawOval (175, 20, 50, 80);
- drawOval (175, 50, 80, 20);
- Which of the following is not true about GUI containers?
- A GUI container is a component that is used to hold and organize other components.
- A frame is a container displayed as a separate window with a title bar.
- A panel is a container that cannot be displayed on its own but is used to organize other components.
- A frame cannot be repositioned and resized on the screen as needed.
- Which of the following is not true about GUI events?
- An event in Java is an object that represents some activity to which we may want to respond.
- Even a movement of a mouse can be considered as an event.
- Since Java does not strictly define classes that represent typical events, users should define their own events.
- We set up a listener object to respond to an event when it occurs.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Please help me with this. I am really struggling Please help me modify my java matching with the image provide below or at least fix the errors import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Game extends JFrame implements ActionListener { int hallo[][] = {{4,6,2}, {1,4,3}, {5,5,1}, {2,3,6}}; int rows = 4; int cols = 3; JButton pics[] = new JButton[rows*cols]; public Game() { setSize(600,600); JPanel grid = new JPanel(new GridLayout(rows,cols)); int m = 0; for(int i = 0; iarrow_forwardFor simple usage of GUIs, why would the source codes (shown below) not work?(a) The input/output objects have not been properly handled.(b) The data type of the methods has been defined in a wrong way.(c) The necessary parameters in the methods have not been assigned properly.(d) The flow of execution has been applied in a wrong way. import javax.swing.JOptionPane;public class Palindrome{public static void main(String[] args) //main method{long num1, num2; //variables to store the numbersString inputStr, outputStr; // strings to receive from the user// and displayinputStr=JOptionPane.showInputDialog("Enter the number: ");num1 = Long.parseLong(inputStr); // change the string tonum2 = num1; // numbersif (num1 <= 0){num1 = - num1; //if the numbers are negative, change the sign inputStr = inputStr.valueOf(num1);//change the inputStr value } if (isPalindrome(inputStr))outputStr = num2 + " is a palindrome";elseoutputStr = num2 + " is not a palindrome";JOptionPane.showMessageDialog(null,…arrow_forwardIn JavaScript, how are an object and an interface related to each other? a. The object’s interface consists of the code and data needed for that object. b. An object’s interface is analogous to a pocket calculator’s inner workings. c. Built-in JavaScript objects do not require an interface, but custom objects do. d. An interface allows another program to access an object’s properties and methods.arrow_forward
- Hi I want to create a JAVA program that will allow me to create a canvas (specifically in eclipse). Can someone straighten my code import java.awt.*;import javax.swing.*;class Main extends JFrame { // constructor Main() { super("canvas"); // create a empty canvas Main c = new Main() { // paint the canvas public void paint(Graphics g) { // set color to red g.setColor(Color.red); // set Font g.setFont(new Font("Bold", 1, 20)); // draw a string g.drawString("This is a canvas", 100, 100); } }; // set background c.setBackground(Color.black); add(c); setSize(400, 300); show(); } // Main Method public static void main(String args[]) { Main c = new Main(); }} ReplyForwardarrow_forwardPlease type and execute this java program and also need an output for this java program as soon as possible.arrow_forwardWrite in Java! Write the Widget class. a. A widget has a name and price. b. The default for name should be “widget” c. Write a constructor (not the no-args), all getters and setters. d. Add a toString method. e. Create an object of type Widget.arrow_forward
- Implement the following in the .NET Console App. Write the Bus class. A Bus has a length, a color, and a number of wheels. a. Implement data fields using auto-implemented Properies b. Include 3 constructors: default, the one that receives the Bus length, color and a number of wheels as input (utilize the Properties) and the Constructor that takes the Bus number (an integer) as input.arrow_forwardPlease code in java and follow the instructions as it is.. Dont use very advanced methods. refer the images provided Hired by the popular nightclub, 'Club B-day' to automate the job of their bouncers. ClubB-day has a novel criterion for entering, where it must be that person's birthday. Additionallystate law stipulates that a patron must also be aged 21 or over to enter the premises since alcoholis served. Your robot must determine whether a person can enter the club given the followingconditions:● Is today their birthday?● Are they 21 years or older?InputYour solution must take in a total of six numerical inputs with each input separated by a space.The first three inputs represent the current month, current day, current year as integers. Theother three inputs represent the birth month, birthday, birth year as integers.OutputThe output should display as a boolean result whether the patron can enter or not.arrow_forwardPlease help me with this using java. A starters code is provided (I created a starters code). For more info refer to the image provided. Please ensure tge code works Starter code:import javax.swing.*; import java.applet.*; import java.awt.event.*; import java.awt.*;public class halloween extends Applet implements ActionListener{ int hallow[] [] = ? int rows = 4; int cols = 3; JButton pics[] = new JButton [rows * cols]; public void init () { Panel grid = new Panel (new GridLayout (rows, cols)); int m = 0; for (int i = 0 ; i < rows ; i++) { for (int j = 0 ; j < cols ; j++) { pics [m] = new JButton (createImageIcon ("back.png")); pics [m].addActionListener (this); pics [m].setActionCommand (m + ""); pics [m].setPreferredSize (new Dimension (128, 128)); grid.add (pics [m]); } }m++; }}add (grid); }public void actionPerformed (ActionEvent…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
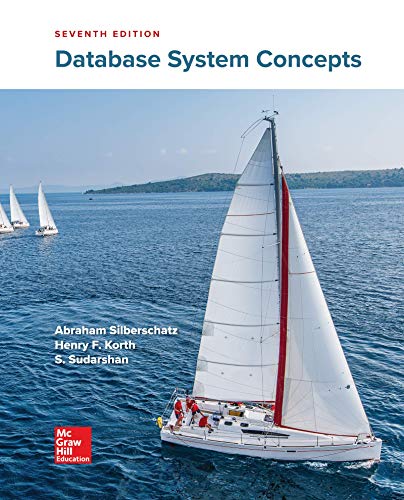
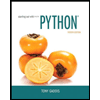
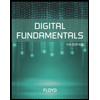
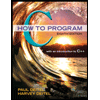
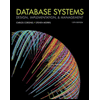
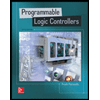