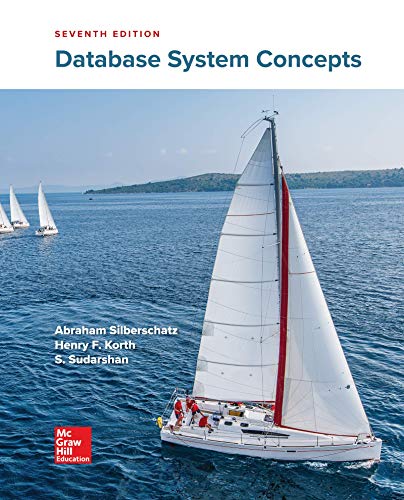
Given an array A[0 : n−1] of n integers, compute the maximum sum of non-empty consecutive subsequence present in the array.
For example, if the input is A = [3, 2, 5, 1, 6], then the output max sum is 17 (the corresponding maximum sum consecutive subsequence is [3, 2, 5, 1, 6]. If the input is A = [−2, 11, −4, 13, −5, −2], then the output max sum is 20 (the corresponding maximum sum consecutive subsequence is [11, −4, 13].
(a) Given input A[0 : n − 1] and n, design a divide-and-conquer
(b) Let T(n) be the runtime of your algorithm when the input size is n. Establish the recurrence relation of T(n) for your algorithm, and then solve the recurrence relation to provide the big-O runtime of your algorithm. [You can use
any method to solve the recurrence relation.]

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- You're given an array arr. Apply the following algorithm to it: find intervals of consecutive prime numbers and consecutive non-prime numbers; replace each such interval with the sum of numbers in it; if the resulting array is different from the initial one, return to step 1, otherwise return the result. Input A non-empty integer array such that: -10000 ≤ arr[i] ≤ 10000 1 ≤ arr.length ≤ 1000 Output An integer array. Examples For arr = [1, 2, 3, 5, 6, 4, 2, 3] the result should be [21, 5]: [1, 2, 3, 5, 6, 4, 2, 3] --> [(1), (2 + 3 + 5), (6 + 4), (2 + 3)] --> [1, 10, 10, 5] [1, 10, 10, 5] --> [(1 + 10 + 10), (5)] --> [21, 5] For arr = [-3, 4, 5, 2, 0, -10] the result should be [1, 7, -10]: [-3, 4, 5, 2, 0, -10] --> [(-3 + 4), (5 + 2), (0 + -10)] --> [1, 7, -10] Solve it in C# pleasearrow_forwardin java ecplise . Implement a program that randomly generates 10 integers from -100 to 100,stores them in a 1D array, and finds their maximum value. Calculate theexecution time of finding a maximum. Repeat the test for 10,000 and10,000,000 numbers. Provide your results in the form of a table below andprovide a small summary. Implement a Java program that will populate a 5x5 matrix with randomlygenerated integers from 0 to 100.(1) print your matrix in a table form.(2) modify your code to multiply all even numbers by 10 and print the matrix.Your output of questions (1) and (2) should match the format of the followingsample outputs:arrow_forward1. Asymptotics. Given an array A of n integers, you'd like to output a two-dimensional n x n array B in which B[i, j] = max {A[i], A[i + 1],..., A[j]} for each i < j. For i j the value of B[i, j] can be left as is. for i = 1,2, η for j = i + 1, n " Compute the maximum of the entries A[i], A[i + 1], , A[j]. Store the maximum value in B[i, j]. (a) Find a function of such that the running time of the algorithm is O(f(n)), and clearly explain why. (b) For the same function f argue that the running time of the algorithm is also (f(n)). (This establishes an asymptotically tight bound (f(n)).) (c) Design and analyze a faster algorithm for this problem. You should give an algorithm with running O(g(n)), where lim→∞ g(n)/f(n) = 0.arrow_forward
- The algorithm below searches for the maximum in an input array A. Assume A is a random sequence containing n distinguishable real numbers, what is the probability for line #4 to be executed exactly once? (The phrase "random sequence" here means the n numbers can appear in any order with equal chance.) mymax(A, n) { 1: max = A[1]; 2: for i = 2:n 3: if A[i] > max 4: max = A[i); 5: end; 6: end; 7: return max; }arrow_forwardA majority element is an element that makes up more than half of the items inan array. Given a positive integers array, find the majority element. If there is no majority element,return -1. Do this in O(N) time and 0(1) space.Input: 1 2 5 9 5 9 5 5 5Output: 5arrow_forward15. Consider the problem of finding the first position in which an array b occurs as a subsequence of an array a. Write two nested loops: let result undefined for (let i = 0; i < a.length - b.length; i++) { for (let j = 0; j < b.length; j++) { if (a[i+j] = b[j]) . . . } } Complete with labeled break and continue statements.arrow_forward
- an array of integers nums sorted in ascending order, find the startingand ending position of a given target value. If the target is not found in thearray, return [-1, -1]. For example:Input: nums = [5,7,7,8,8,8,10], target = 8Output: [3,5]Input: nums = [5,7,7,8,8,8,10], target = 11Output: [-1,-1].arrow_forwardLet M(n) be the minimum number of comparisons needed to sort an array A with exactly n ele- ments. For example, M(1) = 0, M(2) = 1, and M(4) = 4. If n is an even number, clearly explain why M(n) = 2M(n/2) + n/2.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
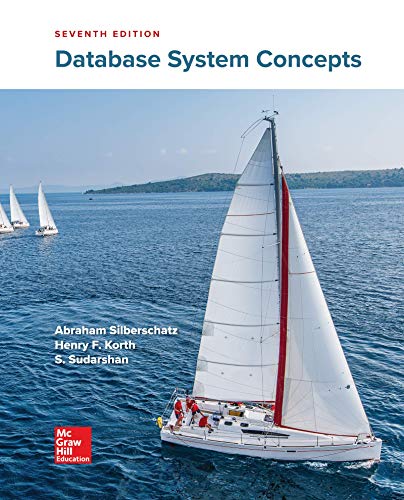
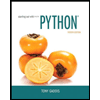
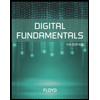
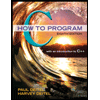
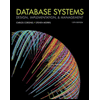
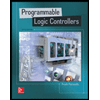