Given main.py, complete the Stack class in Stack.py by writing the push() and pop() methods. The stack uses an array of size 5 to store elements. The command Push followed by a positive number pushes the number onto the stack. The command Pop pops the top element from the stack. Entering -1 exits the program. Output "Can't push, stack is full" when push() is called on a full stack. Output "Can't pop, stack is empty" when pop() is called on an empty stack. Ex. If the input is: Push 1 Push 2 Push 3 Push 4 Push 5 Pop -1 Code given main.py(read-only) from Stack import Stack if __name__ == "__main__": stack = Stack(5) input_line = input() while input_line != '-1': split_input = input_line.split(' ') command = split_input[0] if command == 'Push': number_to_push = int(split_input[1]) stack.push(number_to_push) print('Stack contents (top to bottom):') stack.print_stack() elif command == "Pop": stack.pop() print('Stack contents (top to bottom):') stack.print_stack() input_line = input() Stack.py (needs edit) class Stack: def __init__(self, allocation_size): self.array = [None] * allocation_size self.top_index = -1 # TODO: Write a method to push a value into the stack # TODO: Write a method to pop the top value from the stack def print_stack(self): i = self.top_index while i >= 0: print(self.array[i]) i = i - 1 print()
Given main.py, complete the Stack class in Stack.py by writing the push() and pop() methods. The stack uses an array of size 5 to store elements. The command Push followed by a positive number pushes the number onto the stack. The command Pop pops the top element from the stack. Entering -1 exits the program.
Output "Can't push, stack is full" when push() is called on a full stack. Output "Can't pop, stack is empty" when pop() is called on an empty stack.
Ex. If the input is:
Push 1
Push 2
Push 3
Push 4
Push 5
Pop -1
Code given
main.py(read-only)
from Stack import Stack
if __name__ == "__main__":
stack = Stack(5)
input_line = input()
while input_line != '-1':
split_input = input_line.split(' ')
command = split_input[0]
if command == 'Push':
number_to_push = int(split_input[1])
stack.push(number_to_push)
print('Stack contents (top to bottom):')
stack.print_stack()
elif command == "Pop":
stack.pop()
print('Stack contents (top to bottom):')
stack.print_stack()
input_line = input()
Stack.py (needs edit)
class Stack:
def __init__(self, allocation_size):
self.array = [None] * allocation_size
self.top_index = -1
# TODO: Write a method to push a value into the stack
# TODO: Write a method to pop the top value from the stack
def print_stack(self):
i = self.top_index
while i >= 0:
print(self.array[i])
i = i - 1
print()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

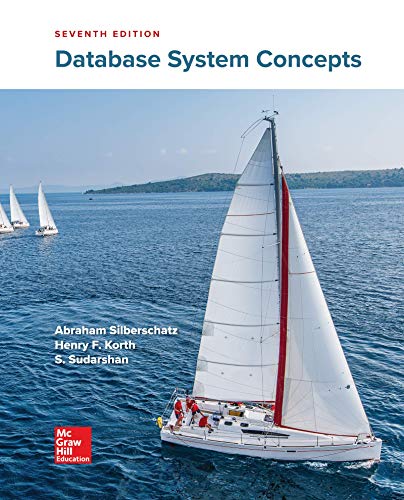
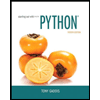
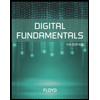
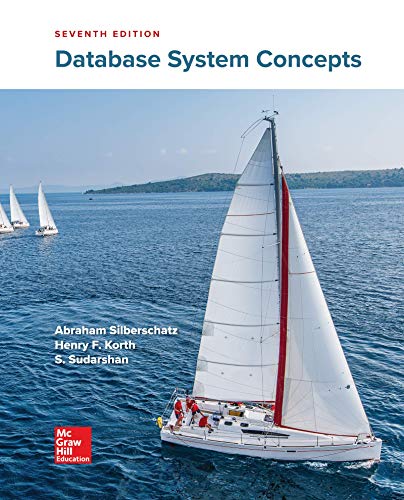
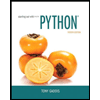
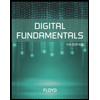
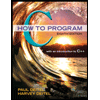
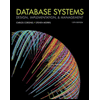
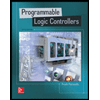