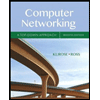
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
WILL GIVE THUMBS UP IF CORRECT AND WITH EXPLANATION.
WILL GIVE THUMBS DOWN IF INCORRECT OR INSUFFICIENT EXPLANATION.
Given the code snippet below which returns a list of all the permutations of a string "inp" with length x. Find the following:
- The recurrence relation of the running time of func(inp)? (Assume that appending to a list and string concatenation both run in constant time or O(1). Hint: Determine the number of returned elements first)
- The worst case time complexity of func(inp). (Be as precise as possible with factorials. In other words, between any factorials such as n! (n+1)! and (n-1)!, only the most precise answer will be acepted)
![def func(inp):
if len(inp) < 1:
return [""]
list = []
for x in range(len(inp)):
for y in func(inp[:x] + inp[x+1:]):
list.append (inp[x] + y)
return list
print(func (input()))](https://content.bartleby.com/qna-images/question/50530471-3d1d-446c-af31-a941cdfd5635/87432179-9fe0-4a2b-80b4-2ad583d3f35a/xmp838a_thumbnail.png)
Transcribed Image Text:def func(inp):
if len(inp) < 1:
return [""]
list = []
for x in range(len(inp)):
for y in func(inp[:x] + inp[x+1:]):
list.append (inp[x] + y)
return list
print(func (input()))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Similar questions
- Write a function longest_streak(string, char) that receives as parameters a strings and a character (which is a string of length 1); the function must return the longest streak of that character in the string (i.e. what is the longest sequence of that character contained in the string). solve in pyhtonarrow_forwardThe goal is to rewrite the function, below, such that passes in a different list of parameters, particularly eliminating the need to pass low and high for each recursive call to binary_search. defbinary_search(nums,low,high,item): mid=(low+high)//2iflow>high:returnFalse #The item doesn't exist in the list!elifnums[mid]==item:returnTrue# The item exists in the list!elifitem<nums[mid]:returnbinary_search(nums,low,mid-1,item)else:returnbinary_search(nums,mid+1,high,item) The new function should be prototyped below. The number of changes between the given version, and the one requested is not significant. defbinary_search(nums,item):pass# Remove this and fill in with your code Tip: If you consider that high and low are used to create a smaller version of our problem to be processed recursively, the version requested will do the same thing, just through a different, more Pythonic technique.arrow_forwardC++ only Example Input14 31 1 1 24 14 23 2Example Output432arrow_forward
- Complete the functional program given below to return the longest palindromic substring of the string passed as a parameter. class Solution { public: string longestpalindrome(string s) { //write your code here } //write code here for any extra functions needed }; Allowed Time Complexity: O(n^2) Use constant space. Language: C++arrow_forwardRemains code. Implement regular expression matching with support for '.' and '*'. '.' Matches any single character.'*' Matches zero or more of the preceding element. The matching should cover the entire input string (not partial). The function prototype should be:bool is_match(const char *s, const char *p) Some examples:is_match("aa","a") → falseis_match("aa","aa") → trueis_match("aaa","aa") → falseis_match("aa", "a*") → trueis_match("aa", ".*") → trueis_match("ab", ".*") → trueis_match("aab", "c*a*b") → true""" def is_match(str_a, str_b): """Finds if `str_a` matches `str_b` Keyword arguments: str_a -- string str_b -- string """ len_a, len_b = len(str_a) + 1, len(str_b) + 1 matches = [[False] * len_b for _ in range(len_a)] # Match empty string with empty pattern matches[0][0] = True # Match empty string with .* for i, element in enumerate(str_b[1:], 2): matches[0][i] = matches[0][i - 2] and element == '*' for i, char_a in…arrow_forward‘Write a function nesting(), which takes an arbitrary number of parameters, and returns a list containing the arguments. Write another function unnesting(), which takes a list L as the parameter and retums a 1D list. Notice that, you should be able to use loops to solve thisproblem and should not use recursive functions (which we will not cover in this course). ‘Write assertions to test the two functions.For example nesting(1, nesting(2, 3, nesting(4, 5, [6])), [7, 8], 9)unnesting([1, [2, 3, [4, 5, [6]]], [7. 8], 9]) (1, [2, 3, [4, 5, [6]]], [7, 8], 9][1, 2,3, 4, 5, 6, 7, 8 9]arrow_forward
- Can someone help me with this question in C++ and not any other programming language No library functions that leverage hash and maps can be used except to make it all lowercase List sentence(s) with the maximum no. of occurrences of the word "the" in the entire file and also list the corresponding frequency. List sentence(s) with the maximum no. of occurrences of the word "of" in the entire file and also list the corresponding frequency. List sentence(s) with the maximum no. of occurrences of the word "was" in the entire file and also list the corresponding frequency. here is a link too the file its called tiny1 https://drive.google.com/file/d/1ariNN_rW9c3vijK1hvjObcQJafYQ2gzm/view?usp=sharingarrow_forwardIn Kotlin, Write a recursive function called myZip, with an expression body. MyZip takes two Lists and returns a List of Pairs in which each value may be any type (I suggest your start by thinking out how to represent this data type). The pairs consist of corresponding elements in the two lists (the first element of the first list and the first element of the second list, etc). The base case should be that either (or both) of the original lists has length 1, so that, if the lists have different length, the zipping stops when the first list runs out of values.arrow_forwardQ3. Let E = {a, b}. Let BDFA = {(M)| M is a DFA that accepts some string containing an equal number of as and bs). Show that BDFA is decidable. (Hint: remember the different results/theorems about CFLs, provide a semi-formal discussion/argument)arrow_forward
- Complete the follow Kotlin program by defining “positives” and “negatives” values for a collection of numbers using the filter higher ordered function.The Filter takes lambda expression as their input. For the “negatives” value, use the it shorter notation. fun main() {val numbers = listOf(1, -2, 3, -4, 5, -6) println("Numbers: $numbers") println("Positive Numbers: $positives") println("Negative Numbers: $negatives") }arrow_forwardWrite a function filter_codes(list_codes) that receives a list of codes and returns another list containing those codes that: Only have numeric characters; and Are at least 2 characters long; and At most 6 characters long Hint: remember that Python has a built-in function for determining if a string only contains numeric characters. solve using pythonarrow_forwardI need correct answer unique answer for this. Don't copy from anywhere otherwise I'll report your account for sure. Last time I received wrong answer for this. This time i need right answer. Do not send wrong and plagiarism responsearrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
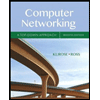
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
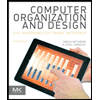
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
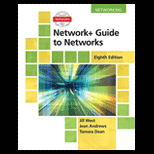
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
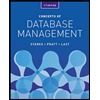
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
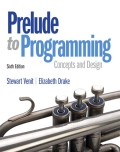
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
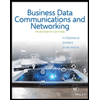
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY