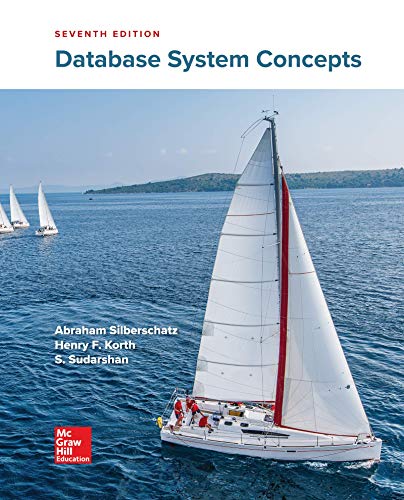
Graphs: Depth First Traversal
Starting with the same graph program as last assignment, implement a depth first traversal method. Test iy on nodes 1, 2, and 3 as start nodes.
Graph program:
#include <iostream>
#include <
#include <string>
using namespace std;
class Edge;
//-------------------------------------------------------------
//
//
class Node
{
public:
Node(string iname)
{
name = iname;
}
string name;
int in_count = 0;
bool visited = false;
vector<Edge *> out_edge_list;
};
//-------------------------------------------------------------
//
//
class Edge
{
public:
Edge(string iname, double iweight, Node *ifrom, Node *ito)
{
name = iname;
weight = iweight;
from = ifrom;
to = ito;
}
string name;
double weight;
Node *from;
Node *to;
bool visited = false;
};
//-------------------------------------------------------------
//
//
class Graph
{
public:
vector<Node *> node_list;
vector<Edge *> edge_list;
//----------------------------------------------------------
//
Node* find_node(string name)
{
for(Node *n : node_list)
if (n->name == name) return n;
return 0;
}
//----------------------------------------------------------
// Add a new edge ( and possibly new nodes) to the graph.
//
void add_edge(string name, double weight, string node_name_from, string node_name_to)
{
Node *node_from, *node_to;
if (!(node_from = find_node(node_name_from)))
node_list.push_back(node_from = new Node(node_name_from));
if (!(node_to = find_node(node_name_to)))
node_list.push_back(node_to = new Node(node_name_to));
Edge *new_edge = new Edge(name, weight, node_from, node_to);
edge_list.push_back(new_edge);
node_from->out_edge_list.push_back(new_edge);
}
void print_nodes()
{
cout << "\nNodes\n=======================\n";
for(Node *n : node_list)
cout << n->name << ' ' << n->in_count << endl;
}
void print_edges()
{
cout << "\nEdges\n=======================\n";
for(Edge *e : edge_list)
cout << e->name << ' ' << e->from->name << ' ' << e->to->name << endl;
}
//----------------------------------------------------------
// Initialize Node in counts.
//
void init_in_counts()
{
}
};
//-------------------------------------------------------------
//
//
int main()
{
Graph g;
g.add_edge("e1", 1.0, "1", "4");
g.add_edge("e2", 2.0, "1", "5");
g.add_edge("e3", 3.0, "2", "3");
g.add_edge("e4", 4.0, "2", "4");
g.add_edge("e5", 5.0, "3", "4");
g.add_edge("e6", 6.0, "3", "6");
g.add_edge("e7", 7.0, "3", "8");
g.add_edge("e1", 8.0, "4", "5");
g.add_edge("e3", 9.0, "5", "7");
g.add_edge("e3", 10.0, "5", "9");
g.add_edge("e3", 11.0, "6", "7");
g.add_edge("e3", 12.0, "7", "9");
g.add_edge("e3", 13.0, "8", "9");
g.print_nodes();
g.print_edges();
return 0;
}
Here is a pseudo-code description:
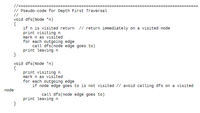

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forward/** * This class will use Nodes to form a linked list. It implements the LIFO * (Last In First Out) methodology to reverse the input string. * **/ public class LLStack { private Node head; // Constructor with no parameters for outer class public LLStack( ) { // to do } // This is an inner class specifically utilized for LLStack class, // thus no setter or getters are needed private class Node { private Object data; private Node next; // Constructor with no parameters for inner class public Node(){ // to do // to do } // Parametrized constructor for inner class public Node (Object newData, Node nextLink) { // to do: Data part of Node is an Object // to do: Link to next node is a type Node } } // Adds a node as the first node element at the start of the list with the specified…arrow_forwardJava (LinkedList) - Grocery Shopping Listarrow_forward
- #include <iostream> usingnamespace std; class Queue { int size; int* queue; public: Queue(){ size = 0; queue = new int[100]; } void add(int data){ queue[size]= data; size++; } void remove(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } else{ for(int i =0; i < size -1; i++){ queue[i]= queue[i +1]; } size--; } } void print(){ if(size ==0){ cout <<"Queue is empty"<<endl; return; } for(int i =0; i < size; i++){ cout<<queue[i]<<" <- "; } cout << endl; } //your code goes here }; int main(){ Queue q1; q1.add(42); q1.add(2); q1.add(8); q1.add(1); Queue q2; q2.add(3); q2.add(66); q2.add(128); q2.add(5); Queue q3 = q1+q2; q3.print();…arrow_forwardJAVA CODE Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics. You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…arrow_forwardAssume a linked list contains following integers: 7, 2, 9, 5, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed: Node<int> *curNode=head; Node<int> *aNode; int s; while(curNode!=NULL){ { aNode=curNode; curNode=curNode->getNext(); } s=aNode->getItem(); A. 15 B. 3 C. 7 D. 2arrow_forward
- 1 Assume some Node class with info & link fields. Complete this method in class List that returns a reference to the node containing the data item in the argument find This, Assume that find this is in the list public class List { protected Node head; protected int size; fublic Public Node find (char find This)arrow_forwardIn java The following is a class definition of a linked list Node:class Node{int info;Node next;}Assume that head references a linked list and stores in order, the int values 5, 7 and 9. Show the instructions needed to delete the Node with 5 so that head would reference the list 7 and 9.arrow_forward#include <iostream> using namespace std; #define SIZE 5 //creating the queue using array int A[SIZE]; int front = -1; int rear = -1; //function to check if the queue is empty bool isempty() { if(front == -1 && rear == -1) return true; else return false; } //function to enter elements in queue void enqueue ( int value ) { //if queue is full if ((rear + 1)%SIZE == front) cout<<"Queue is full \n"; else { //now the first element is inserted if( front == -1) front = 0; //inserting element at rear end rear = (rear+1)%SIZE; A[rear] = value; } } //function to remove elements from queue void dequeue ( ) { if( isempty() ) cout<<"Queue is empty\n"; else //only one element if( front == rear ) front = rear = -1; else front = ( front + 1)%SIZE; } //function to show the element at front void showfront() { if( isempty()) cout<<"Queue is empty\n"; else cout<<"element at front is:"<<A[front]; } //function to display the queue void…arrow_forward
- 1.Assume some Node class with info link fields . Complete this method in class List that returns a reference to the node containing the data item in the argument findThis, Assume that findThis is in the list public class list { protected Node head ; Protected int size ; Public Node find (char find this) { Node curr = head; while(curr != null) { if(curr.info == findThis) return curr; curr = curr.link; } return -1; } }arrow_forwardThe C++ Vertex class represents a vertex in a graph. The class's only data member is _____ Ⓒlabel 1000 O weight O framEdges O toEdges Question 36 The C++ Graph class's fromEdges member is a(n) _________ O string Ⓒdouble O vector O unordered_map Question 37 The C++ Graph class's AddVertex() function's return type is _____ void O Edge+ Overtex) O vectorarrow_forwardGiven the following definition for a LinkedList: // LinkedList.h class LinkedList { public: LinkedList(); // TODO: Implement me void printEveryOther() const; private: struct Node { int data; Node* next; }; Node * head; }; // LinkedList.cpp #include "LinkedList.h" LinkedList::LinkedList() { head = nullptr; } Implement the function printEveryOther, which prints every other data value (i.e. those at the odd indices assuming 0-based indexing).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
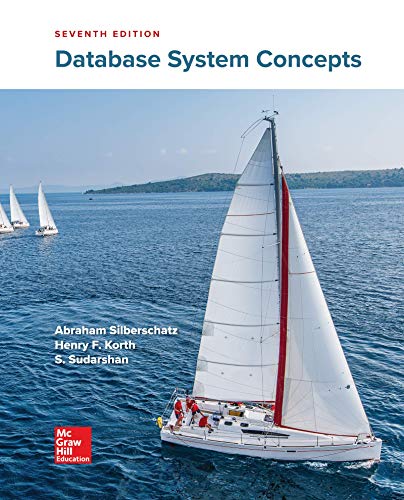
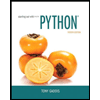
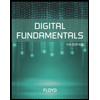
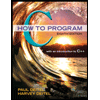
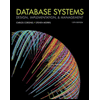
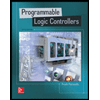