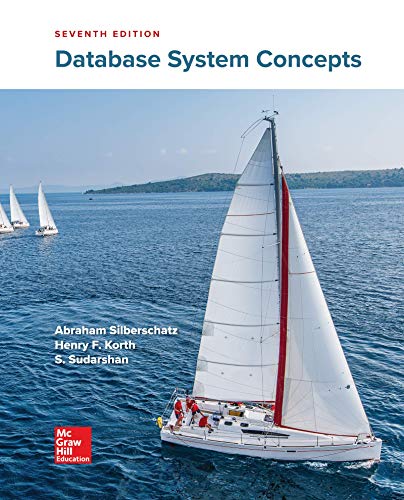
Hello! I am not sure how I would write in Python code that can generate random numbers without using the built-in import random function. Is this possible?
So far, my code is:
import random
# Define 20 random numbers between 1-100
numbers_random = [random.randint(1, 100) for _ in range(20)]
# Find the lowest number of the random numbers
numbers_lowest = min(numbers_random)
# Find the highest number of the random numbers
numbers_highest = max(numbers_random)
# Define the total of all numbers
numbers_total = sum(numbers_random)
# Define the average of all numbers
numbers_average = total / len(numbers_random)
# Show all data
print("Twenty random numbers:", numbers_random)
print("Lowest number:", numbers_lowest)
print("Highest number:", numbers_highest)
print("Total of the numbers:", numbers_total)
print("Average of the numbers:", numbers_average)
What I am doing with this Python code is creating 20 random numbers that are between 1-100 and showing the lowest number, highest number, the total and the averages whilst using the built-in list functions. I just want to challenge myself by not using the import random function at the top. Is there a way to do that?

In the provided scenario,The challenge is to generate random numbers in Python without using the built-in random
module. Instead, we'll create a custom pseudo-random number generator to mimic the functionality of the random
module. This involves designing a basic algorithm for generating random-like numbers. Keep in mind that these numbers won't be truly random, but they can simulate randomness effectively for educational purposes. The custom generator will be used to create 20 pseudo-random numbers within a specified range. The code will then calculate statistics such as the lowest number, highest number, total, and average of the generated values, much like what the random
module enables. This exercise offers insight into the underlying mechanisms of random number generation and provides a valuable learning experience.
Step by stepSolved in 4 steps with 1 images

- Write a Python program that will generate 1000 random numbers between 1 and 100 inclusive from the random generator. For each value returned from the random generator, keep a count of the number of even numbers generated and the number of odd numbers generated. Use the following functions within this program: - getRandom(): this function will call the random generator and return the generated integer value. - isOdd(): this function will return true or false depending on whether the number generated is odd or even. - update(): this function will update the counters for odd or even. - display(): once the program has completed 1000 random numbers, the counters for the number of odd and even will be displayed. REQUIREMENTS: - Add a beginning statement when the program starts to execute. - Add an ending statement when the program is complete. - Call the random generator 1000 times for a randomly generated number between…arrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardWrite a program in C as follows:- Create an array of integers named “toy” that has 120 rows and 4 columns.- The program should repeatedly display the following menu:A or a to add a toy to the bagV or v to calculate and display the total value of the toysW or w to calculate and display the total weight of the toysD or d to delete a toy from the arrayM or m to calculate and display the number of small toysN or n to calculate and display the number of medium toysL or l to calculate and display the number of large toysX or x to start filling a new bagP or p to exit program - Santa’s bag can hold 30 large toys or 60 medium toys or 120 small toys or any combination ofsizes that satisfy this requirement (ex: 29 large + 1 medium + 2 small would be max capacity).Also, the total weight of toys cannot exceed 620 Kgs. All values are entered in centimeters andgrams. (Hint: the return values of the size function should help you in calculating the bagcapacity.)The…arrow_forward
- I wrote this code in c programming. what this code should do is ask how many times its going to run and run that many times(this works), ask how many books there are in a place(this works), then ask the max number of pages you want to read(this works), then asks how many pages there are in a book and put that into an array(works), then sorts the array of pages(works), and then adds the number in the array until you cant which is less than or equal to the max number of pages you want to read, and puts how many books you read(does not work), and then prints out how many books you read. a sample input is 35 206 12 3 10 25 2112 3 6 10 210 319 6 6 3 8 2 12 15 13 7 sample out put should be 345 #include <stdio.h> #include <stdlib.h> void MergeSort(int values[], int start, int end); void Merge(int values[], int start, int middle, int end); void add(int pages[], int count[], long long maxPages, long long total); void Print_Array(int count[], int cases); int main(void) {…arrow_forwardYou have been hired by a local organic sod company to write coverage calculator for their website. Organic sod is cut into rectangles 9 square foot in area for lawn installation. Your program will prompt the customer for the length and the width of a rectangular area being covered with sod. Implement the following "pseudocode" to obtain the number of pieces to order. • Compute the area of the rectangle to be covered • Divide the area by 9 • Convert the result to an integer and then add 1 Below is a sample run with Python output in blue and user input in black. Enter length:200 Enter width:40 You should order 889 pieces.arrow_forward. Initialize please.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
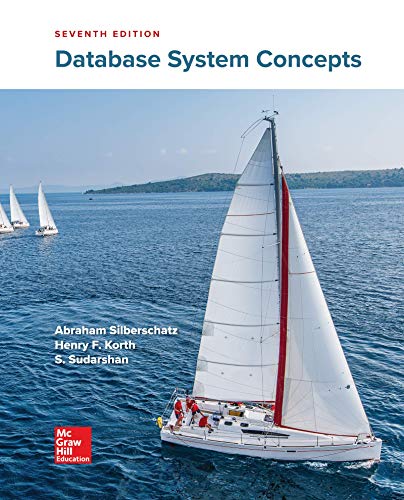
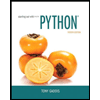
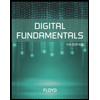
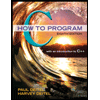
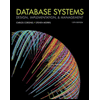
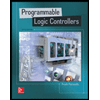