Hello! I could really use help for these two problems. Program 1 Show how well dressed you and your date are. Your program will prompt for two inputs, the style of you and your date. Input for each should be a integer on a scale of 1-10, with 10 being the best. You can be seated immediately if either of you are 'stylin' (8 or higher) . However, if you or your date have a style of 2 or less, then you won't be seated, even if one of you is a 8 or more. Otherwise, you'll have to wait for a table. Based on the inputs, the program should indicate if you can be seated right away, if you have to wait, or if there are no tables available. Sample output shown below: Rate how dressed up you are, with 10 being the highest ==> 8 How about your date? Same scale ==> 9 Your table is ready! Rate how dressed up you are, with 10 being the highest ==> 9 How about your date? Same scale ==> 2 Sorry, No table available Rate how dressed up you are, with 10 being the highest ==> 5 How about your date? Same scale ==> 5 Please wait for a table. My Code so far for program 1: import java.util.*; public class DateNight { public static void main(String args[]) { Scanner Sc = new Scanner(System.in); int input1; // Print a message asking for first persons level of dress. System.out.print("Welcome to our restaurant! Please enter your level of dress, with 10 as the highest : "); // Ask for the dates level of dress. int input2; System.out.print("Now, please enter your dates level of dress, with 10 as the highest : "); if(input1 + input2 >= 8) { System.out.println("You and your date can be seated right away!"); } else if(input1 <= 7 && >= 3) && (input2 <=7 & >= 3){ System.out.println("Please wait for a table."); } Program 2 Calculate a electric bill. Prompt for the meter reading as a integer with the folowing calculations: if the meter reading is less than or equal to 50 units then the rate is $ 0.50 per unit if the meter reading is greater than 50 and less than 100 units, then the rate is $0.75 per unit if the meter reading is greater or equal to 100, but less than 250, then the rate is $1.20 per unit Anything 250 and above is 1.50 per unit The electric use = meter reading x rate. An additional surcharge of 20% should be added to the bill. Else-if statements should be used to determine the rate. Print the electric use rate, the surcharge, and the total amount due. Formatting the numbers to currency format is not necessary. Sample Output- Enter the meter reading:125 Electric Use: 150.0 Surcharge is: 30.0 Your energy bill is: $180.0
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Hello! I could really use help for these two problems.
Show how well dressed you and your date are. Your program will prompt for two inputs, the style of you and your date. Input for each should be a integer on a scale of 1-10, with 10 being the best. You can be seated immediately if either of you are 'stylin' (8 or higher) . However, if you or your date have a style of 2 or less, then you won't be seated, even if one of you is a 8 or more. Otherwise, you'll have to wait for a table.
Based on the inputs, the program should indicate if you can be seated right away, if you have to wait, or if there are no tables available.
Sample output shown below:
Rate how dressed up you are, with 10 being the highest ==> 8
How about your date? Same scale ==> 9
Your table is ready!
Rate how dressed up you are, with 10 being the highest ==> 9
How about your date? Same scale ==> 2
Sorry, No table available
Rate how dressed up you are, with 10 being the highest ==> 5
How about your date? Same scale ==> 5
Please wait for a table.
My Code so far for program 1:
import java.util.*;
public class DateNight {
public static void main(String args[]) {
Scanner Sc = new Scanner(System.in);
int input1;
// Print a message asking for first persons level of dress.
System.out.print("Welcome to our restaurant! Please enter your level of dress, with 10 as the highest : ");
// Ask for the dates level of dress.
int input2;
System.out.print("Now, please enter your dates level of dress, with 10 as the highest : ");
if(input1 + input2 >= 8) {
System.out.println("You and your date can be seated right away!");
}
else if(input1 <= 7 && >= 3) && (input2 <=7 & >= 3){
System.out.println("Please wait for a table.");
}
Program 2
Calculate a electric bill. Prompt for the meter reading as a integer with the folowing calculations:
if the meter reading is less than or equal to 50 units then the rate is $ 0.50 per unit
if the meter reading is greater than 50 and less than 100 units, then the rate is $0.75 per unit
if the meter reading is greater or equal to 100, but less than 250, then the rate is $1.20 per unit
Anything 250 and above is 1.50 per unit
The electric use = meter reading x rate.
An additional surcharge of 20% should be added to the bill.
Else-if statements should be used to determine the rate. Print the electric use rate, the surcharge, and the total amount due. Formatting the numbers to currency format is not necessary.
Sample Output-
Enter the meter reading:125
Electric Use: 150.0
Surcharge is: 30.0
Your energy bill is: $180.0
I would really appriacte any help, with steps on how to solve this if possible. Thanks in advance!

Step by step
Solved in 7 steps with 2 images

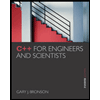
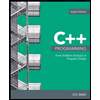
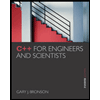
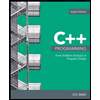