help me solve this in the Java language. The Game class is part 1, but I need you guys to help me with part 2 only. I've already know part 1. Here is my Game Class of part 1. Based on that help me with part 2. public class Test1 { class Game { private String gameName; private String releaseDate; private double cost; private boolean isMultiplayer; public Game () { cost = 0.0; isMultiplayer = false; gameName = "";
*** Please help me solve this in the Java language. The Game class is part 1, but I need you guys to help me with part 2 only. I've already know part 1. Here is my Game Class of part 1. Based on that help me with part 2.
public class Test1 {
class Game {
private String gameName;
private String releaseDate;
private double cost;
private boolean isMultiplayer;
public Game () {
cost = 0.0;
isMultiplayer = false;
gameName = "";
releaseDate = "";
}
public Game (double cost, boolean isMultiplayer, String gameName, String releaseDate) {
if (cost < 0) {
this.cost = 0.0;
}
else {
this.cost = cost;
this.isMultiplayer = isMultiplayer;
this.gameName = gameName;
this.releaseDate = releaseDate;
}
}
public void setgameName (String gameName) {
this.gameName = gameName;
}
public void setreleaseDate (String releaseDate) {
this.releaseDate = releaseDate;
}
public void setisMultiplayer (boolean isMultiplayer){
this.isMultiplayer = isMultiplayer;
}
public void setCost (double cost) {
if (cost < 0) {
this.cost = 0.0;
}
else {
this.cost = cost;
}
}
String getgameName() {
return this.gameName;
}
String getreleaseDate() {
return this.releaseDate;
}
boolean getisMultiplayer() {
return this.isMultiplayer;
}
// Method to calculate the cost
public double CalculateCost (double taxPercentage, double discountPercentage) {
if (cost > 0) {
return (cost + (cost * taxPercentage / 100) - (cost * discountPercentage / 100));
}
else
return 0;
}
}
}
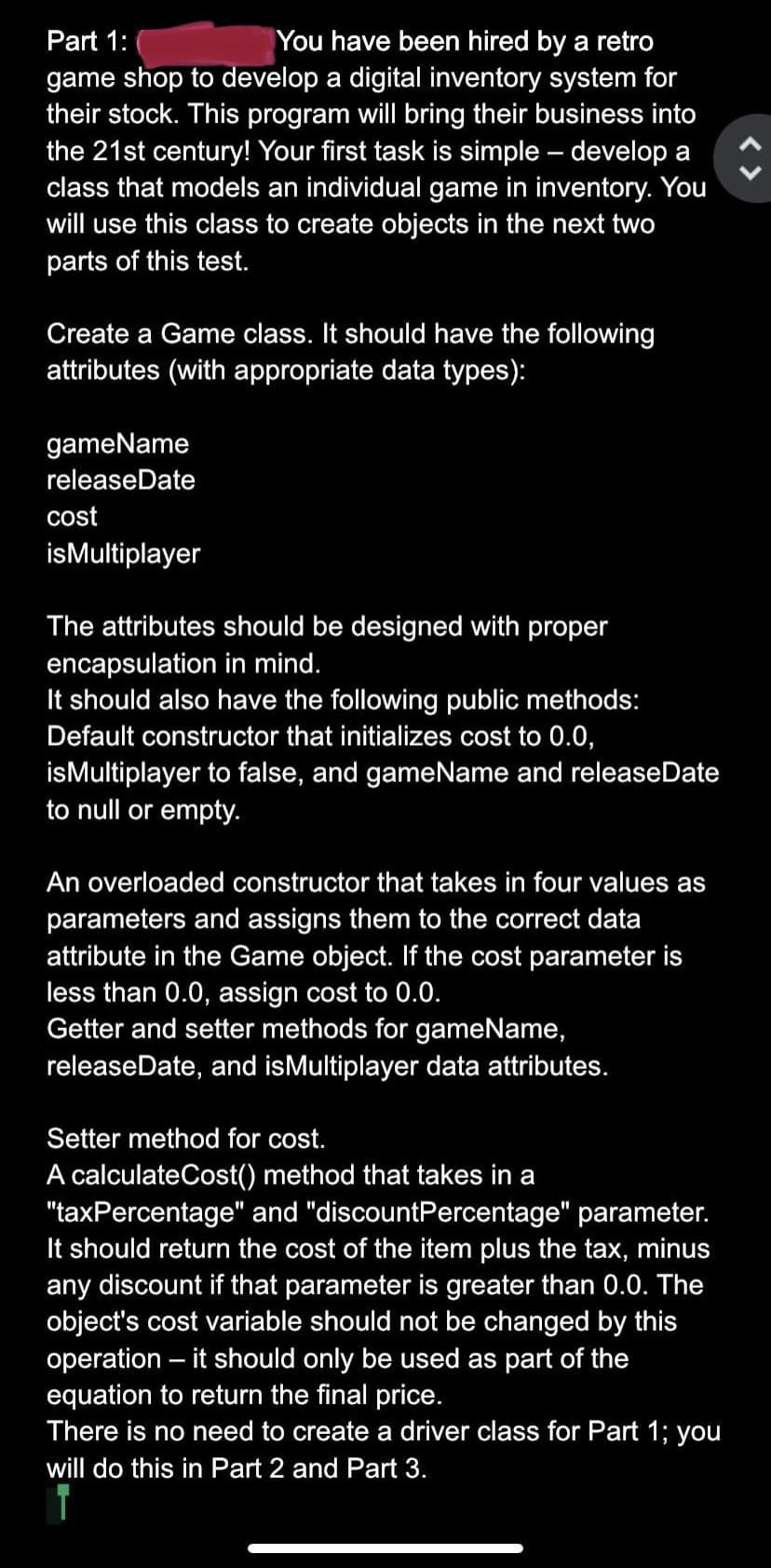
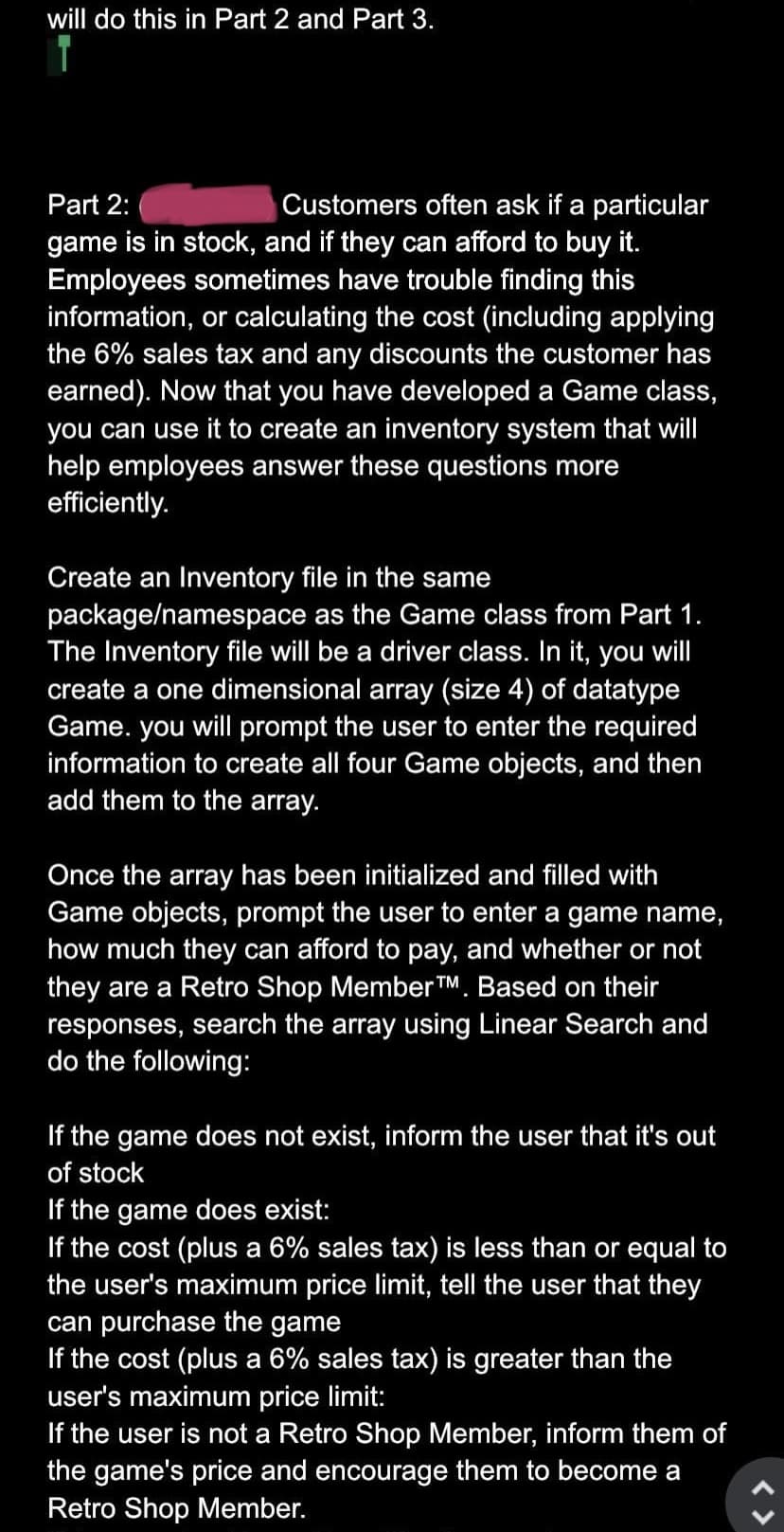

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

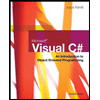
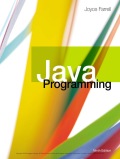
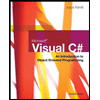
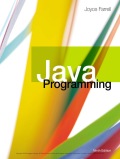