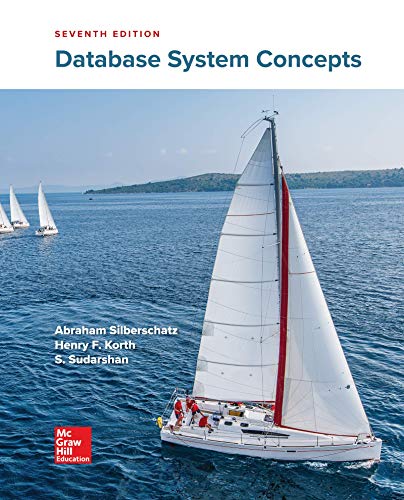
Here's my C++ programming coding assignment for 4-11:
Instructions
Write a program that implements
the
(Chapter 1), which determines the
monthly wages of a salesperson.
The instructions for Example 1 - 3
have been posted below for your
convenience.
Example 1 - 3
Every salesperson has a base
salary. The salesperson also
receives a bonus at the end of each
month, based on the following
criteria: If the salesperson has been
with the store for five years or less,
the bonus is $10 for each year that
he or she has worked there. If the
salesperson has been with the
store for more than five years, the
bonus is $20 for each year that he
or she has worked there. The
salesperson can earn an additional
bonus as follows: If the total sales
made by the salesperson for the
month are at least $5,000 but less
than $10,000, he or she receives a
3% commission on the sale. If the
total sales made by the salesperson
for the month are at least $10,000,
he or she receives a 6%
commission on the sale.
To calculate a salesperson’s
monthly paycheck, you need to
know the base salary, the number
of years that the salesperson has
been with the company, and the
total sales made by the salesperson
for that month. Suppose
baseSalary denotes the base
salary,noOfServiceYears denotes
the number of years that the
salesperson has been with the
store,bonus denotes the bonus,
totalSales denotes the total sales
made by the salesperson for the
month, and additionalBonus
denotes the additional bonus.
Since your program handles
currency, make sure to use a data
type that can store decimals with a
precision to 2 decimals
Here's what I have for my program:
#include <iostream>
#include <iomanip>
#include <string>
using namespace std;
const
const
main ()
{
//Declare variables
int baseSalary;
int noOfServiceYears;
double bonus;
double totalSales;
double additionalSales;
double additionalBonus;
double payCheck;
cout << fixed << showpoint << setprecision(2);
//Get the employee's Base Salary
cout << "Please enter the employee's Base Salary: ";
cin >> baseSalary << endl;
//Get the employee's number of years of service with the company
cout << "Please enter the employee's number of years of service: ";
cin >> noOfServiceYears << endl;
//Calculate the employee's bonus using the following formula
if (noOfServiceYears <= 5)
bonus = 10 * noOfServiceYears;
else
bonus = 20 * noOfServiceYears;
//Get the employee's total number of sales
cout << "Please enter the employee's recordered number of sales: ";
cin >> totalSales << endl;
//Calculate the employee's additional bonus using the following formula
if (totalSales < 5000)
additionalBonus = 0;
else if (totalSales <= 5000 && totalSales < 10000)
additionalBonus = totalSales * 0.03;
else
additionalBonus = totalSales * 0.06;
//Calculate the employee's paycheck using the following equation
payCheck = baseSalary + bonus + additionalBonus;
cout << "The employee's gross-adjusted monthly salary is: " << payCheck << endl;
cin >> payCheck
return 0;
}
I know that my programed parameters is correct. My problem with my program is that I'm missing two constant variables for the bonus calculations of 10 and 20 * noOfServiceYears. Also, I need to input a constant variable for the additionalBonus calculations of 0.03 and 0.06 to complete my program, but I have no idea how to write them within the context of my program. Everytime I think I got it right, my program goes out-of-whack. Can someone help me? I don't want to copy anyone's work. I will only use your ideas as a reference point to complete my code, ok? I believe in doing my own work.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- Python elif ifarrow_forwardMathematical functions introduced in chapter 4 can be used in the mathematical calculations and simulations of the engineering and scientific problems. In these cases, we formulate the mathematical problem and then use the computer for calculations and simulations. Can a computer code solve the math problem and come up with mathematical formulas, i.e. can a computer program do what a mathematician does?arrow_forwardWrite a console in c#arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
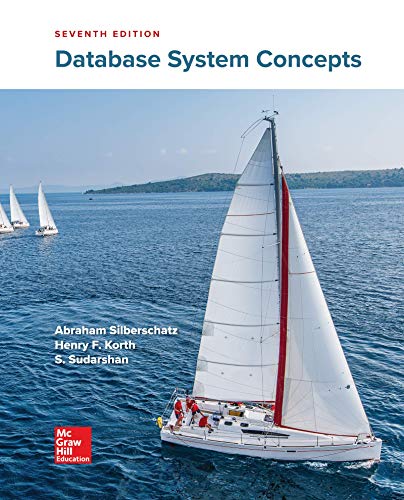
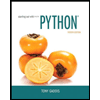
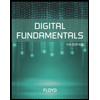
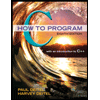
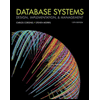
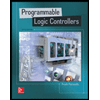