How can I ensure the Capital letters are in the right place?"** Explanation 1) Below is program that define function which accepts a text string and returns a new string constructed by finding all its vowels and reversing their order while keeping all other characters in same order It defines a function reverse_vowels returns new string with vowels reversed Create a list to store vowels in text Loop through all characters in text append the vowels in list Create a new text string to store vowels in reverse Loop through all characters in text if character is vowel pop one vowels from end and update new_text else keep the other text same return new text It has a test code which calls function reverse_vowels with multiple inputs and display new returned text 2) Save program in python file and run Program """Program that define function which accepts a text string and returns a new string constructed by finding all its vowels and reversing their order while keeping all other characters in same order""" #function returns new string with vowels reversed def reverse_vowels(text): #Create a list to store vowels in text vowels = [] #Loop through all characters in text for ch in text: if ch in "aeiouAEIOU": #append the vowels in list vowels.append(ch) #Create a new text string to store vowels in reverse new_text = "" #Loop through all characters in text for ch in text: if ch in "aeiouAEIOU": #pop one vowels from end and update new_text new_text += vowels[-1] vowels = vowels[:-1] else: #keep the other text same new_text += ch #return new text return new_text #Test Code #Call function reverse_vowels with multiple inputs and display new returned text print(reverse_vowels("Revorse the vewels")) print(reverse_vowels("Bengt Hilgursson")) print(reverse_vowels("Why do you laugh? I chose the death")) print(reverse_vowels("These are people you protect with your pain!")) print(reverse_vowels("Who's the leader of the club that's made for you and me? \ T-R-C-K-Y M-O-U-S-E! Tricky Mouse! TRICKY MOUSE! Tricky Mouse! TRICKY MOUSE!\ Forever let us hold our Hammers high! High! High! High!"))
1) Below is program that define function which accepts a text string and returns a new string constructed by finding all its vowels and reversing their order while keeping all other characters in same order
- It defines a function reverse_vowels returns new string with vowels reversed
- Create a list to store vowels in text
- Loop through all characters in text
- append the vowels in list
- Create a new text string to store vowels in reverse
- Loop through all characters in text
- if character is vowel pop one vowels from end and update new_text
- else keep the other text same
- return new text
- It has a test code which calls function reverse_vowels with multiple inputs and display new returned text
2) Save program in python file and run
"""Program that define function which accepts a text string and returns a new string
constructed by finding all its vowels and reversing their order while keeping all
other characters in same order"""
#function returns new string with vowels reversed
def reverse_vowels(text):
#Create a list to store vowels in text
vowels = []
#Loop through all characters in text
for ch in text:
if ch in "aeiouAEIOU":
#append the vowels in list
vowels.append(ch)
#Create a new text string to store vowels in reverse
new_text = ""
#Loop through all characters in text
for ch in text:
if ch in "aeiouAEIOU":
#pop one vowels from end and update new_text
new_text += vowels[-1]
vowels = vowels[:-1]
else:
#keep the other text same
new_text += ch
#return new text
return new_text
#Test Code
#Call function reverse_vowels with multiple inputs and display new returned text
print(reverse_vowels("Revorse the vewels"))
print(reverse_vowels("Bengt Hilgursson"))
print(reverse_vowels("Why do you laugh? I chose the death"))
print(reverse_vowels("These are people you protect with your pain!"))
print(reverse_vowels("Who's the leader of the club that's made for you and me? \
T-R-C-K-Y M-O-U-S-E! Tricky Mouse! TRICKY MOUSE! Tricky Mouse! TRICKY MOUSE!\
Forever let us hold our Hammers high! High! High! High!"))
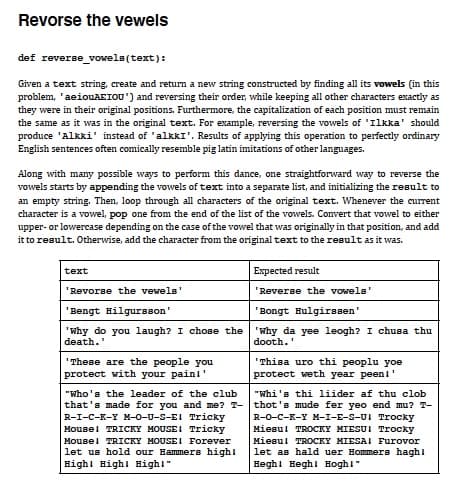

Step by step
Solved in 2 steps with 1 images

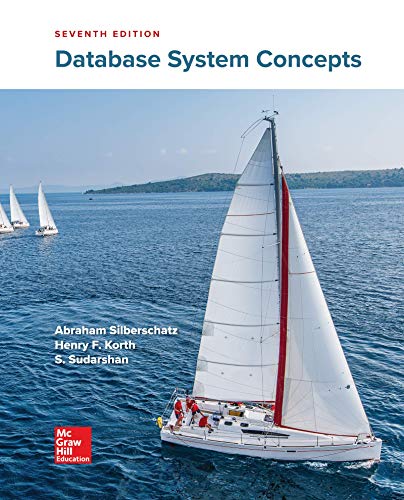
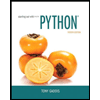
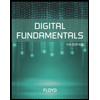
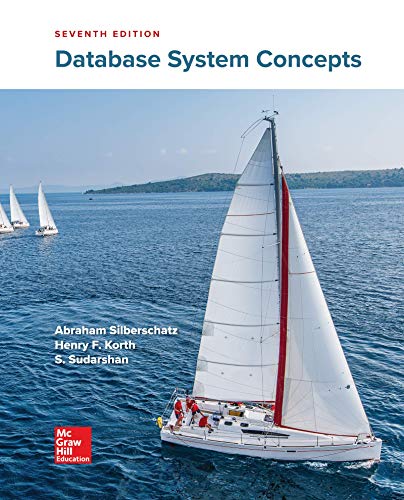
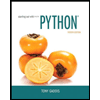
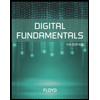
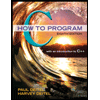
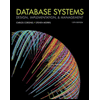
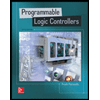