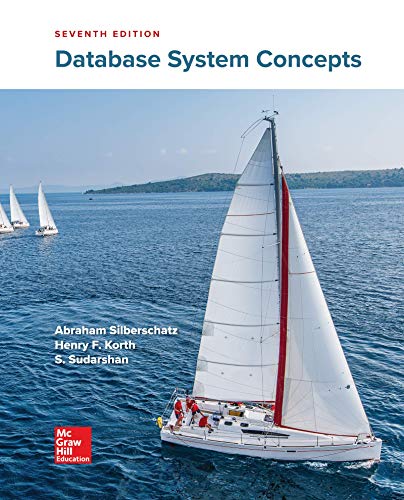
def loopy_madness_with_while_loops(string1: str, string2: str) -> str:
"""
Given two strings <string1> and <string2>, return a new string that
contains letters from these two strings "interwoven" together, starting with
the first character of <string1>. If the two strings are not of equal
length, then start looping "backwards-and-forwards" in the shorter string
until you come to the end of the longer string.
"interwoven" (or "interweaving") means constructing a new string by taking
the first letter from the first string, adding the first letter of the
second string, adding the second letter of the first string,
adding the second letter of the second string, and so on.
"backwards-and-forwards" is a custom looping term. First the loop starts
at position 1 (index 0) and goes until position n (i.e., the end). Once the
loop reaches position n, it goes backwards, starting at position n - 1 and
goes to position 1 (index 0). This repeats until the two strings are
interwoven. For example, the backwards-and-forwards operations of "abc"
would be "abcbabcba..."
>>> loopy_madness_with_while_loops("abcde","12")
'a1b2c1d2e1'
>>> loopy_madness_with_while_loops("ab","123")
'a1b2a3'
>>> loopy_madness_with_while_loops("abcdfe","123")
'a1b2c3d2f1e2'
>>> loopy_madness_with_while_loops("a","b")
'ab'
>>> loopy_madness_with_while_loops("c","ab")
'cacb'
"""
Please do this on python, use while loop and don't use any import, dictionaries, or try-except statement.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Using c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forwardIn the following program skeleton, replace the < insert code here> line with theappropriate code. The resulting program should display the initial list of songs,prompt the user for a string of text to be replaced and then prompt for new text toreplace it. After making the replacement, it should display the updated list of songs.import java.util.Scanner;public class UpdateSongs{public static void main ( String [] args){Scanner stdIn = new Scanner(System.in);String songs =“ 1. Welcome to Your Life – Grouplove\n” +“ 2. Sedona – Houndmouth\n” +“3. Imagine – John Lennon\n” +“4. Bohemian Rhapsody –Queen\n”;String oldText, newText;< insert code here>}}Sample Session:1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Lennon4. Bohemian Rhapsody –QueenEnter text to replace: LennonEnter new text: Dean1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Dean4. Bohemian Rhapsody –Queenarrow_forwarddef findOccurrences(s, ch): lst = [] for i in range(0, len(s)): if a==s[i]: lst.append(i) return lst Use the code above instead of enumerate in the code posted below. n=int(input("Number of rounds of Hangman to be played:")) for i in range(0,n): word = input("welcome!") guesses = '' turns = int(input("Enter the number of failed attempts allowed:")) def hangman(word): secrete_word = "-" * len(word) print(" the secrete word " + secrete_word) user_input = input("Guess a letter: ") if user_input in word: occurences = findOccurrences(word, user_input) for index in occurences: secrete_word = secrete_word[:index] + user_input + secrete_word[index + 1:] print(secrete_word) else: user_input = input("Sorry that letter was not found, please try again: ") def findOccurrences(s, ch): return [i for i, letter in enumerate(s) if letter == ch] *** enumerate not discussed in…arrow_forward
- Given the following code, write a for loop that will replace all potential instances of a, e, and u in the string tester with the letter Q string tester = "Dice Values"; cin >> tester;arrow_forwardWrite a C++ program that stores a list of fruits enumerated in Fruits.txt in a STL list of strings. You need to solve the following problem: · output all the fruits in your STL list · output all fruits that start with letter smaller than ‘m’ · output all fruits that contain less than 6 character symbols · calculate the numeric sum of all letters in each fruit and store your answer in a histogram of length 10 where each element contains a value that is a multiple of 500(e.g. your histogram vector contains 10 values and each value represents the numeric value of each fruit (Note: the grand total of values in you histogram should be equal to the total number of fruits · take all the fruits in Fruits.txt and store the in a STL set<string> container · output all the (unique) fruits in Fruits.txt · output the total number of entries in your set Fruits.txt = {pina, naranja, melon, cereza, uva, ciruela, limon, frutabomba, aguacate, platano, manzana, fresa, lechuga, nuez, sandia, mango,…arrow_forwardWrite a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print:7 9 11 10 10 11 9 7 #include <iostream>using namespace std; int main() { const int NUM_VALS = 4; int courseGrades[NUM_VALS]; int i; for (i = 0; i < NUM_VALS; ++i) { cin >> courseGrades[i]; } /* Your solution goes here */ return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
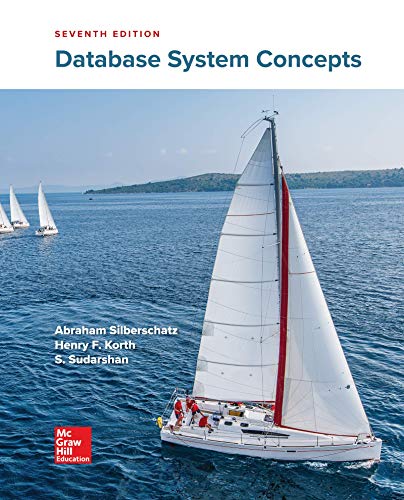
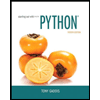
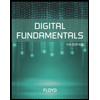
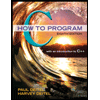
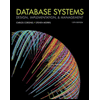
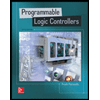