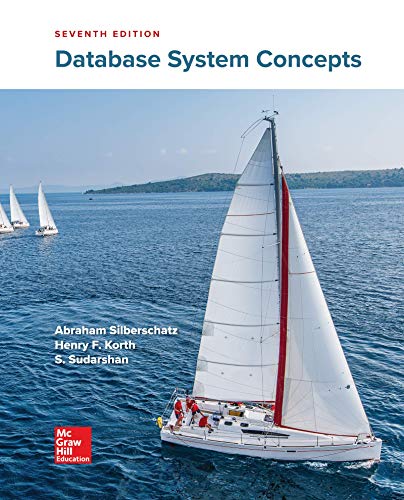
Concept explainers
How do I fix the code?
Code:
import java.util.Scanner;
public class Grades {
static int count = 0;
public static int readGrades() {
int grade,count = 0;
Scanner input = new Scanner(System.in);
grade = input.nextInt();
return grade;
}
public static int sum(int[] arr) {
int sum = 0;
for (int i = 0; i < arr.length; i++)
sum = sum + arr[i];
return sum;
}
public static int sum(int[] arr, int firstIndex, int lastIndex) {
if (firstIndex < 0 || lastIndex >= arr.length || lastIndex >= firstIndex || firstIndex >= arr.length || lastIndex < 0 || firstIndex < lastIndex) {
return -666;
}
int sum = 0;
for (int i = firstIndex; i <= lastIndex; i++)
sum = sum + arr[i];
return sum;
}
public static double average(int[] arr) {
double sum = sum(arr);
return ((float) sum / (float) (arr.length));
}
public static int maxValue(int[] arr) {
int max = 0;
for (int i = 0; i < arr.length; i++) {
if (max < arr[i]) {
max = arr[i];
}
}
return max;
}
public static int maxValue(int[] arr, int firstIndex, int lastIndex) {
if (firstIndex < 0 || lastIndex >= arr.length || lastIndex >= firstIndex || firstIndex >= arr.length || lastIndex < 0 || firstIndex < lastIndex) {
return -666;
}
int max = 0;
for (int i = firstIndex; i <= lastIndex; i++) {
if (max < arr[i]) {
max = arr[i];
}
}
return max;
}
public static int indexOfFirstMaxValue(int[] arr) {
int max = 0;
for (int i = 0; i < arr.length; i++) {
if (maxValue(arr) == arr[i]) {
max = i;
}
}
return max;
}
public static int minValue(int[] arr) {
int min = arr[0];
for (int i = 1; i < arr.length; i++) {
if (min > arr[i]) {
min = arr[i];
}
}
return min;
}
public static int minValue(int[] arr, int firstIndex, int lastIndex) {
if (firstIndex < 0 || lastIndex >= arr.length || lastIndex >= firstIndex || firstIndex >= arr.length || lastIndex < 0 || firstIndex < lastIndex) {
return -666;
}
int min = arr[0];
for (int i = firstIndex; i <= lastIndex; i++) {
if (min > arr[i]) {
min = arr[i];
}
}
return min;
}
public static int indexOfFirstMinValue(int[] arr) {
int min = 0;
for (int i = 0; i < arr.length; i++) {
if (minValue(arr) == arr[i]) {
min = i;
}
}
return min;
}
public static int numberOfBelowAverageElements(int[] arr) {
int count = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] < average(arr)) {
count++;
}
}
return count;
}
public static int numberOfAboveAverageElements(int[] arr) {
int count = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] > average(arr)) {
count++;
}
}
return count;
}
public static void rotateElements(int[] arr) {
int i, temp;
temp = arr[0];
for (i = 0; i < arr.length - 1; i++) {
arr[i] = arr[i + 1];
}
arr[arr.length - 1] = temp;
System.out.print("\nRotated array is: \n");
for (int k = 0; k < arr.length; k++) {
System.out.print(arr[k] + " ");
}
}
public static void rotateElements(int[] arr, int rotationCount) {
for (int i = 0; i < rotationCount; i++)
rotateElements(arr);
System.out.print("\nRotated array is: \n");
for (int k = 0; k < arr.length; k++) {
System.out.print(arr[k] + " ");
}
}
public static void reverseArray(int[] arr) {
int n = arr.length;
int[] b = new int[n];
int j = n;
for (int i = 0; i < n; i++) {
b[j - 1] = arr[i];
j = j - 1;
}
System.out.print("\nReversed array is: \n");
for (int k = 0; k < n; k++)
System.out.print(b[k] + " ");
}
public static void main(String[] args) {
int grade[] = new int[5];
int i = 0;
System.out.println("Enter a grade : ");
while ( i < 5) {
grade[i] = readGrades();
if (grade[i] == -1)
{
break;
}
else {
i++;
count++;
}
}
System.out.println("Number of grades: " + count);
//take care of the firstIndex and lastIndex parameters [0, count-1]
System.out.println("The maximum grades is: " + maxValue(grade, 0, count-1));
System.out.println("The minimum grades is: " + minValue(grade, 0, count -1));
}
}
![**Testing `Grades.sum(int[] arr, int firstIndex, int lastIndex)` with `firstIndex < 0`:**
- **Your output:**
Incorrect indexes entered.
- **Test feedback:**
The method `Grades.sum(int[] arr, int firstIndex, int lastIndex)` is not properly checking `firstIndex < 0`.](https://content.bartleby.com/qna-images/question/ecfe1b28-5736-4fb7-bb45-1ff6972df94e/25e0b59a-acc9-401c-a6fe-3cd48cca2443/pas94fs_thumbnail.png)
![**Testing Scenario**
This test evaluates the `Grades.sum(int[] arr, int firstIndex, int lastIndex)` method by checking the condition where `firstIndex >= arr.length`.
**Expected Output:**
- The method should handle incorrect index entries properly.
**Your Output:**
- Message: "Incorrect indexes entered."
**Test Feedback:**
- Error Message: "***** Error ***** Grades.sum(int[] arr, int firstIndex, int lastIndex) invoked with invalid indexes."
**Explanation:**
The test checks if the method correctly identifies and handles cases where the starting index (`firstIndex`) is beyond or equal to the length of the array, which is an invalid scenario for array operations. The feedback suggests that there was an error due to invalid index parameters used in the method call.](https://content.bartleby.com/qna-images/question/ecfe1b28-5736-4fb7-bb45-1ff6972df94e/25e0b59a-acc9-401c-a6fe-3cd48cca2443/2kpnbd5_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 12 images

- program that takes a first name as the input, and outputs a welcome message to that name. Ex: If the input is: Chrystal the output is: Hey Chrystal! Welcome to zyBooks!arrow_forwardConsider the class below. public class Addition { private int sum = 0; public static void add (int x, int sum) { sum += x; }//end method add public static void main (String[] args) { int sum = 0; add (2, sum); add (3, sum); add (4, sum); "1 System.out.println("The total is: + sum); } //end main method }//end class Addition The programmer who wrote the code above and expected the program's output to say: The total is: 9 That is, the programmer wanted the add method to add values to the sum class variable. Fix the code so that it works properly by making edits such as changing names, crossing out code, adding code, etc.arrow_forwardFind the errors in this code: public class TstArray { public static void main(String args[]){ int a[] = {5, 4, 9}; int avg; int total; for(int i=0; i< a.length; i++){ total = 0; total += a[i]; } avg = total/a.length; } }arrow_forward
- Type the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 8, ""); } } Input 5arrow_forwardFix all logic and syntax errors. Results Circle statistics area is 498.75924968391547 diameter is 25.2 // Some circle statistics public class DebugFour2 { public static void main(String args[]) { double radius = 12.6; System.out.println("Circle statistics"); double area = java.Math.PI * radius * radius; System.out.println("area is " + area); double diameter = 2 - radius; System.out.println("diameter is + diameter); } }arrow_forwardpublic static int countIt (int n) { int count = 0; for (int i = n; i > 0; i /= 2) for (int j = 0; j < 10; j++) count += 1; return count; } Big-O notation: Explanation:arrow_forward
- import java.util.Scanner; public class MorgansBonuses { public static void main(String[] args) { int WeeksWorked; int Reviews; int x, y; final int Quit = 99; double[][] bonuses = { { 5, 9, 16, 22, 30 }, { 10, 12, 18, 24, 36 }, { 20, 25, 32, 42, 55, 68 }, { 46, 54, 65, 77, 90 }, { 60, 72, 84, 96, 120 }, { 85, 100, 120, 140, 175 } }; Scanner ID = new Scanner(System.in); System.out.println("Please enter the number of weeks the employee has worked or " + Quit + " to quit.>>"); WeeksWorked = ID.nextInt(); while (WeeksWorked != Quit) { System.out.println("Please enter the number of positive reviews the employee has recieved or " + Quit +" to end program.>>"); Reviews = ID.nextInt(); if (WeeksWorked >= bonuses.length) { WeeksWorked = bonuses.length - 1; } if (Reviews >= bonuses[0].length) { Reviews =…arrow_forwardCorrect Code for Java: Enter an integer >> 11 Enter another integer >> 23 The sum is 34 The difference is -12 The product is 253 import java.util.Scanner; public class DebugTwo2 { public static void main(String args[]) { int a, b; Scanner input = new Scanner(System.in); System.out.print("Enter an integer >> "); a = nextInt (); System.out.print("Enter b = nextInt (); System.out.println("The System.out.println("The System.out.println("The } } another integer >> "); sum is " + (a + b)); difference is " + (a - b)); product is + (a*b));arrow_forwardint stop = 6; int num =6; int count=0; for(int i = stop; i >0; i-=2) { num += i; count++; } System.out.println("num = "+ num); System.out.println("count = "+ count); } }arrow_forward
- Fix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forwardHow do I fix the errors? Code: import java.util.Scanner;public class ReceiptMaker { public static final String SENTINEL = "checkout";public final int MAX_NUM_ITEMS;public final double TAX_RATE;private String[] itemNames;private double[] itemPrices;private int numItemsPurchased; public ReceiptMaker() {MAX_NUM_ITEMS = 10;TAX_RATE = 0.0875;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;}public ReceiptMaker(int maxNumItems, double taxRate) {MAX_NUM_ITEMS = maxNumItems;TAX_RATE = taxRate;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;} public void greetUser() {System.out.println("Welcome to the " + MAX_NUM_ITEMS + " items or less checkout line");}public void promptUserForProductEntry() {System.out.println("Enter item #" + (numItemsPurchased + 1) + "'s name and price separated by a space, or enter \"checkout\" to end transaction early");}public void addNextPurchaseItemFromUser(String…arrow_forwardclass isinfinite_output { public static void main(String args[]) { Double d = new Double(1 / 0.); boolean x = d.isInfinite(); System.out.print(x); } } What will the given code prints on the output screen.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
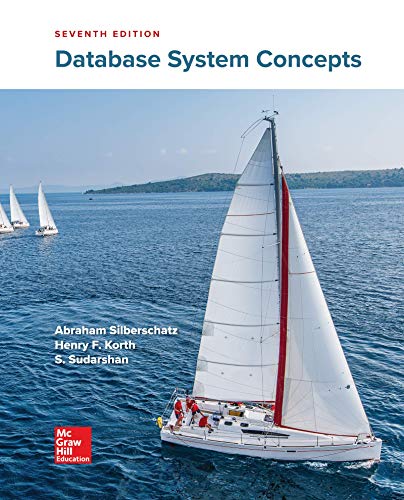
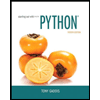
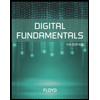
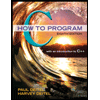
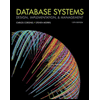
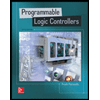