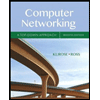
How do I make my code print out possitive odd numbers?
example:
Input: 5
Output: 1,3,5
Input: 8
Output: 1,3,5,7
import java.lang.Math;
import java.util.Scanner;
public class MidtermProblems
{
public static String odds(int a)
{
int odds;
odds = a;
String s;
if(odds > 0)
{
//s = odds;
return "" + odds;
}
else if(odds < 0)
{
s = "None";
}
else
{
s = "";
}
return s;
}
public static void main(String[] args) {
Scanner in = new Scanner(System.in);
int a=0;
System.out.println("Enter a: ");
a = in.nextInt();
String result = odds(a);
result = MidtermProblems.odds(a);
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- import java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forwardpublic class Course{String name;String dept;int number;public Course (String n, String d, int n){name = n;dept = d;number = n;}public String toString(){return "Course " + name + " in " + dept + " #: " + number;}public static void main (String [] args){Scanner in = new Scanner(System.in);add 10 lines using the scanner and saving them as strings The input for each line will be entered a String, a space, another String, and an int. Parse the input string into those three items and make an instance of Course. Add the new Course instance to a generic ArrayList of Course. print out each Course in the ArrayList.arrow_forwardQuestion #4: Re-write the program of Question #3 using data files. The input data numbers.txt contains (positive) integer numbers. Each time the program reads a number, check whether it is a prime and stores the result in the output file Results.txt. 373 373 is Prime!! 11 11 is Prime!! 2552 is NOT prime!! 5 is Prime!! 3561 is NOT prime!! 2552 3561 numbers.txt Results.txtarrow_forward
- import java.util.Scanner;public class MP2{public static void main (String[] args){//initialization and declarationScanner input = new Scanner (System.in);String message;int Option1;int Option2;int Quantity;int TotalBill;int Cash;int Change;final int SPECIALS = 1;final int BREAKFAST = 2;final int LUNCH = 3;final int SANDWICHES = 4;final int DRINKS = 5;final int DESSERTS = 6;//For the Special, Breakfast, Lunch Optionsfinal int Meals1 = 11;final int Meals2 = 12;final int Meals3 = 13;final int Meals4 = 14;//First menu and First OptionSystem.out.println("\t Welcome to My Kitchen ");System.out.println("\t\t SPECIALS");System.out.println("\t\t [2] BREAKFAST MEALS");System.out.println("\t\t [3] LUNCH MEALS");System.out.println("\t\t [4] SANDWICHES");System.out.println("\t\t [5] DRINKS");System.out.println("\t\t [6] DESSERTS");System.out.println("\t\t [0] EXIT");System.out.print("Choose an option ");Option1 = input.nextInt();//first switch case statementsswitch(Option1){case (SPECIALS):message…arrow_forwardConsider the following piece of code. (The numbers on the left margin denote line numbers.) line#01 public class funcRef {02 private int n;03 public funcRef (int m) {n = m;}04 public static void func (funcRef t) {t.n++;}05 public static void main (String [] args) {06 funcRef t1, t2;07 t1 = new funcRef(20);08 t2 = t1;09 System.out.print(t2.n);10 func(t1);11 System.out.print(t2.n);12 func(t1);13 System.out.print(t1.n);14 func(t2);15 System.out.print(t1.n);16 func(t2);17 System.out.print(t1.n);18 System.out.print(t1.n);19 return;20 }21 } Out of the following, tick the true statements and leave the false statements unticked.(More than one of the statements could be true. You get full marks only for marking all the statements correctly. There are no partial marks.) A. The output of the program will be 202022222222. B. When func(t1) is called in line…arrow_forwardJava Questions - (Has 2 Parts). Based on each code, which answer out of the choices "A, B, C, D, E" is correct. Each question has one correct answer. Thank you. Part 1 - Given the following code, the output is __. class Sample{ public static void main (String[] args){ try{ System.out.println(5/0);}catch(ArithmeticException e){ System.out.print("Divide by zero????");}finally{ System.out.println("5/0"); } }} A. Divide by zero????B. 5/0C. Divide by zero????5/0D. 5/0Divide by zero????E. "5/0" Part 2 - Given the following code, the output is __. try{ Integer number = new Integer("1"); System.out.println("An Integer instance.");}catch (Exception e){ System.out.println("An Exception.");} A. An Integer instance.B. An Exception.C. 1D. Error: ExceptionE. None of the optionsarrow_forward
- Type the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 16, ""); } } Input 0arrow_forwarduse the JCreator program for code in picture and show the outputarrow_forwardInput class Output { public static void main(String args[]) { int x , y = 1; x = 10; if (x != 10 && x / 0 == 0) System.out.println(y); else System.out.println(++y); } } Find outputarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
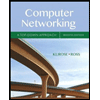
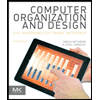
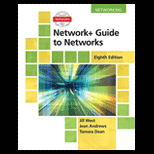
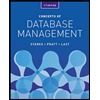
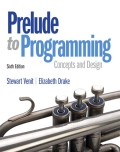
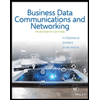