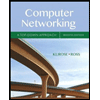
How to create an application class called ImageProcessorApplicationMT and an image processor class called ImageProcessorMT according to below?
Exercise 1: The Thread Pool
For this exercise you should create your own implementation of a thread pool. In preparation for this exercise, you should change the ImageProcessorMT class so that it implements the Runnable interface. Your thread pool will manage threads that run your new ImageProcessorMTs. The thread pool class itself should also implement Runnable.
The thread pool should offer a constructor that receives the size of the thread pool as a parameter.
The thread pool has relatively simple functionality. It should be possible to submit a task
(i.e. an ImageProcessorMT) to the thread pool. This does not mean that the task will actually run yet, just that the thread pool is aware of it and keeps it in a waiting list. The number of ImageProcessorMTs that are added can exceed the number of threads available in the thread pool.
It should also be possible to start the thread pool. This means that the thread pool will then run as many ImageProcessorMTs as it can up to its size. For example, if the thread pool size is 5, but 10 ImageProcessorMTs have been added, it can only run 5.
When any ImageProcessorMT has terminated, a new one from the waiting list should be started by the thread pool (if one exists). The thread pool should continue to do this until the waiting list is empty, when it should itself terminate.
The thread pool should offer a join() method that causes the calling thread to wait until the thread pool has terminated.
Note that when you change ImageProcessorMT to implement Runnable, its run() method works the same way as it does in ImageProcessorST: it processes the image and then exits (terminating the thread). You might wish to add a boolean and a getter to ImageProcessorMT that signals that the run method has terminated, hence the result is available, and the thread pool can now run another thread (if the waiting list was not empty).
Exercise 2: Parallelising ImageProcessorMT
For this exercise you will take the existing ImageProcessorMT class and parallelise the filtering and greyscale operations. Remember, the ImageProcessorMT class itself should already now implement Runnable as a result of Exercise 1.
Parallelising these operations means simply ‘slicing’ the image data and giving each slice to a thread to work on. If there are two threads, for example, each would perform the filtering or greyscale operation on half of the image data. This means that the pixel data produced by all of the threads must be ‘reassembled’ at the end to create one array of pixel data as the result.
There are some practical limits to this approach. For example, if an image has dimensions 256x256 then, at
5
most, you can set 256 threads working on the image - one row of pixels each5. However, this might negate the benefits of parallelism due to the overhead introduced by the parallelisation. You should parametrise the number of threads created and experiment with the effect of changing the number of threads. To get your code working, make sure at first that it works with one thread before adding more.

Step by stepSolved in 3 steps with 1 images

- Can you help me with this code because i don't know what to do with this code, this code has to be in C. question that I need help with:You need to use the pthread for matrix multiplication. Each threadfrom the threadpool should be responsible for computing only a partof the multiplication (partial product as shown in the above picture –all Ti(S) are called a partical product). Your main thread should splitthe matrices accordingly and create the partial data arrays that areneeded to compute each Ti. You must create a unique task with thedata and submit it to the job queue. You can compute the partialproducts concurrently as long as you have threads available in thethreadpool. You have to remove the task the from queue and submitto a thread in the threadpool. You should define the number ofthreads to be 5 and keep it dynamic so that we can test the samecode with a higher or lower number of threads as needed. When allthe partial products are computed all the threads in the…arrow_forwardPlease answer the follwoing regarding the java code: When looking at the code below, assume that the MyThread and MyRunnable classes are correctly implemented in other files. How many threads are there in the program shown below? (Be careful to consider ALL the threads!)arrow_forwardFill in the blanks:5. The ( 6 ) is used to implement mutual exclusion where it can be decremented by aprocess and incremented by another, but the value must either be 0 or 1.6. If deadlock prevention approach is used to deal with deadlocks in a system, the ( 7 )condition can be prevented using the direct method.7. Two threads may share the memory space, but they cannot share the same ( 8 )8. Consider round-robin (RR) scheduling algorithm is implemented with 2 seconds timeslice and it is now selecting a new process; if we have 3 blocked processes (A, B, and C),and A has been waiting the longest, then A would need to wait a period of ( 9 ) secondsto be selected.9. In real-time systems, if a task appears at random times, then it is considered ( 10 ).arrow_forward
- Write java code to create a thread by (extending), theprogram create 3 thread that displaying “fatmah” and thenumber of thread that is running.Rewrite the above program by implementing the RunnableInterfacearrow_forwardModify this threading example to use, exclusively, multiprocessing, instead of threading. import threadingimport time class BankAccount(): def __init__(self, name, balance): self.name = name self.balance = balance def __str__(self): return self.name # These accounts are our shared resourcesaccount1 = BankAccount("account1", 100)account2 = BankAccount("account2", 0) class BankTransferThread(threading.Thread): def __init__(self, sender, receiver, amount): threading.Thread.__init__(self) self.sender = sender self.receiver = receiver self.amount = amount def run(self): sender_initial_balance = self.sender.balance sender_initial_balance -= self.amount # Inserting delay to allow switch between threads time.sleep(0.001) self.sender.balance = sender_initial_balance receiver_initial_balance = self.receiver.balance receiver_initial_balance += self.amount # Inserting delay to allow switch between threads time.sleep(0.001)…arrow_forwardMulti-tasking can not be achieved with a single processor machine. True False 2. Async/Await is best for network bound operations while multi-threading and parallel programming is best for CPU-bound operations. True False 3. The following is a characteristic of an async method:The name of an async method, by convention, ends with an "Async" suffix. True False 4. Using asynchronous code for network bound operations can speed up the time needed to contact the server and get the data back. True False 5. Asynchronous programming has been there for a long time but has tremendously been improved by the simplified approach of async programming in C# 5 through the introduction of: The Task class True Falsearrow_forward
- Exercise 1: Write a thread class TabPrinter that prints the elements of an array of integers (in one line) every 2 seconds 5 times. Use the way of extending the class thread. Write the main method which creates and starts three threads Printer which will print different arrays of integers. After that it prints "Main won’t wait. Main exits". Modify the above thread program so that you implement the interface Runnable. Make the main thread waiting till all other threads finish execution. ____________________________________________________________________________________________ Exercise 2: Write a thread class TextThread that prints a text every 1 second 10 times. Read the following main class. Try to guess what will be its output. class Test { public static void main(String s[]) throws Exception { TextThread x = new TextThread ("I am thread x"); TextThread y= new TextThread ("I am thread y"); System.out.println("I am Main thread"); } } Write it and execute it. Is the…arrow_forwardCould you kindly utilize solely my code as I contributed to its development? I emphasize, please utilize only my code.I attached my code below label my code. The question that I need help with: You need to use the pthread for matrix multiplication. Each threadfrom the threadpool should be responsible for computing only a partof the multiplication (partial product as shown in the above picture –all Ti(S) are called a partical product). Your main thread should splitthe matrices accordingly and create the partial data arrays that areneeded to compute each Ti. You must create a unique task with thedata and submit it to the job queue. You can compute the partialproducts concurrently as long as you have threads available in thethreadpool. You have to remove the task the from queue and submitto a thread in the threadpool. You should define the number ofthreads to be 5 and keep it dynamic so that we can test the samecode with a higher or lower number of threads as needed. When allthe…arrow_forwardSteps to be followed: Create a class called MyThread that extends the Thread class. Declare an instance variable int limitToStop in the MyThread class. Create a parameterized constructor in the MyThread class that takes an int as a parameter to initialize a limitToStop instance variable. Override the run() method in the MyThread class. In the run() method, put a for-loop that goes from 0 to limitToStop. The block associated with the for-loop should print out only the odd numbers. Call Thread.sleep(1000) after every number you print out in the for-loop you created in the previous step. The Thread.sleep(1000) method throws an InterruptedException , which is a checked exception. Therefore, we need to enclose the Thread.sleep(1000) call in a try block. Also, add a catch block associated with the try that catches the InterruptedException and prints out the exception information using the printStackTrace() method. Create a MyThread variable using the new keyword in the main()…arrow_forward
- Subject Name: Advanced Object-Oriented Programming 1. Complete the below given code (1) class NyThread Thread t: Runnable ( MyThread (String n, int pl t (2) (3) : / creates teh Thread t with nane n : // changen the priority to p Syster.out.printin ("Thread "+n+* created with priority "+p): t. (4) : // exexcute the thread t. I // The only abatarct aethed in Runnable public for (int 1=10: 1>=1: 1--) { Syaten.out.prántin ("Thread *t.getName ()+"\e i - "a) : (5) try { (6) :/ ma ke the Thead t eleep to 2 seconds } catch (Exception e) Syater.out.printin ("Exception in Child"): System.out.printin ("Thread "+t. getName ()+" 1s Exiting"): public statie void main (Stringt) arga) { MyThread t1, 12; ti - new MyThread ("R1", 7): t2 = new MyThread ("R1", 3): try ( :// 2 atatements to aake the Threads ti a v2 exit at same time (8) ) catoh (Exception e) ( Syster.out.printin("Exception in main"): System.out.printin ("Thread Main is Exiting"): 1. 2. 3. 4. 6. 7. 8. 5.arrow_forwardEach group represents one OOP principle.Create a new thread and for each grouping, identify the OOP principle that is best represented by the images i the group. Explain what made you think the grouping represents the OOP principle you chose. Use acronym APIE to recall what the principles are - Abstraction, Polymorphism, Inheritance, Encapsulation. Grouping 1 caring1 caring 2 caring3 caring5 Grouping 2 brown Blue House Key family Grouping 3 package1 package2 package3 package4 Grouping 4 painting thoughts car leafarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
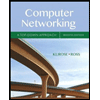
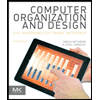
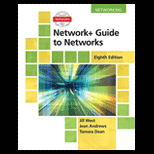
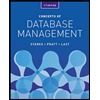
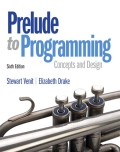
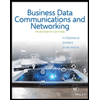