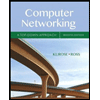
I need help editing this code I keep getting an error
import java.util.Scanner;
public class Factorial
{
public static void main(String[] args)
{
Scanner scan=new Scanner(System.in);
System.out.print("Enter a number to find its factorial value : ");
//read an integer value from keboard
int num=Integer.parseInt(scan.nextLine());
//Calling fact method
System.out.printf("Facorial of %d is %d ", num,fact(num));
}
/*Recursive method, fact
* Method name:fact
* Input arguments:n as integer
* Output arguments: an integer value
* */
public static int fact(int n)
{
//Base case :
//Return 1 if n value is either 0 or 1
if(n==0 || n==1)
return 1;
else
//calling method, fact with n-1
return n*fact(n-1);
}//
}//
![**ASSIGNMENT:**
Replace the sample code with the program shown above that draws two lines shown in Figure A.
Modify the program to add two more lines each connecting to the ends of the two existing lines.
[Diagram Description: Figure A depicts a rhombus shape with two horizontal arrows pointing inward from the left and right sides of the shape.]
Run the program to test.](https://content.bartleby.com/qna-images/question/c43e03e1-b353-4d50-a726-bab1a8232fef/fdafca03-1001-4b86-b94e-c05e69d06e6f/dz1j058_thumbnail.png)

Answer:
Explanation :
your code is running and this is giving correct result but some compiler gives error so we have change public class Factorial replaced with the public class Main in the line number 2 then this code running fine.
Some compiler error gives the due to this above reason
Step by stepSolved in 3 steps with 1 images

- Please create a flowchart for the following program: // Import Scanner classimport java.util.Scanner; // Create a class Trianglepublic class Main{ // Method used to check given three sides form a valid triangle or not public static boolean isTriangle(double a, double b, double c) { // If sum of any two sides is greater than third side if((a+b > c) && (a+c > b) && (b+c > a)) { // Print triangle is valid System.out.println("Three sides form a valid triangle."); // Return true return true; } // If any of the above condition is false else {// Print triangle is invalidSystem.out.println("Three sides form a invalid triangle.\n"); // Return false return false; } } // Method used to compute the area of triangle public static double triArea(double a, double b, double c) { // Compute s double s = (a + b + c) / 2; // Compute the area of triangle double area = Math.sqrt(s*(s - a)*(s - b)*(s - c)); // Return the…arrow_forwardI have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) {…arrow_forwardHi, I am struggling a bit with this problem. (This is in Java by the way.) Provided code: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userText; // Add more variables as needed userText = scnr.nextLine(); // Gets entire line, including spaces. /* Type your code here. */ }}arrow_forward
- JAVA CODE: check outputarrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forwardimport java.util.Scanner; public class DebugSix4 { public static void main(String[] args) { int high, low, count = 0; final int NUM = 5; Scanner input = new Scanner(System.in); System.out.print("This application displays " + NUM + " random numbers" + "\nbetween the low and high values you enter" + "\nEnter low value now... "); low = input.nextInt(); // Inserted the missing semicolon System.out.print("Enter high value... "); high = input.nextInt(); // Added the missing dot to the method call. while (low >= high) { // For proper input validation, changed "" to ">=" System.out.println("The number you entered for a high number, " + high + ", is not more than " + low); System.out.print("Enter a number higher than " + low + "... "); high =…arrow_forward
- How do I code: public static void rotateElements(int[] arr, int rotationCount) public static void reverseArray(int[] arr) In Java?arrow_forwardusing System; class main { publicstaticvoid Main(string[] args) { Random r = new Random(); int randNo= r.Next(1,11); Console.Write("Hi, my name is Stevie. I am thinking of a number from 1 to 10.\nGuess which number I am thinking of? "); int guess = Convert.ToInt32(Console.ReadLine()); if (guess == randNo) { Console.WriteLine("You guessed correctly!"); } else { Console.WriteLine("I was thinking of the number "+randNo+"\nSorry! Play again."); } } } this is a guessing game program for a random number between 1-10 in C#. how can code be added to tell you if your guess is too high or too low from the chosen random number?arrow_forwardI need help editing this code I keep getting an error import java.util.Scanner;public class Factorial { public static void main(String[] args) { Scanner scan=new Scanner(System.in); System.out.print("Enter a number to find its factorial value : "); //read an integer value from keboard int num=Integer.parseInt(scan.nextLine()); //Calling fact method System.out.printf("Facorial of %d is %d ", num,fact(num)); } /*Recursive method, fact * Method name:fact * Input arguments:n as integer * Output arguments: an integer value * */ public static int fact(int n) { //Base case : //Return 1 if n value is either 0 or 1 if(n==0 || n==1) return 1; else //calling method, fact with n-1 return n*fact(n-1); }//}//arrow_forward
- 8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forwardType the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 16, ""); } } Input 0arrow_forwardI have this code: import java.util.Scanner; public class CalcTax { public static void main(String[] args) { Scanner sc = new Scanner(System.in); // Welcome message System.out.println("Welcome to my tax calculation program."); // Declare the variables int i, pinCode, maxRetries = 3; String choice; // Get the PIN code for (i = 0; i < maxRetries; i++) { System.out.print("Please enter the PIN code: "); pinCode = sc.nextInt(); if (pinCode == 5678) { break; } System.out.println("Invalid pin code. Please try again."); } if (i == maxRetries) { System.out.println("Invalid pin code. You have reached the maximum number of retries."); System.exit(0); } // Get the income, interest, deduction, and paid tax amount double income, interest, deduction, paidTax; do { System.out.print("Please…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
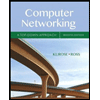
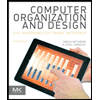
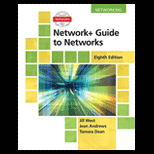
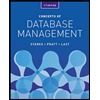
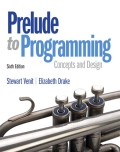
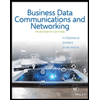