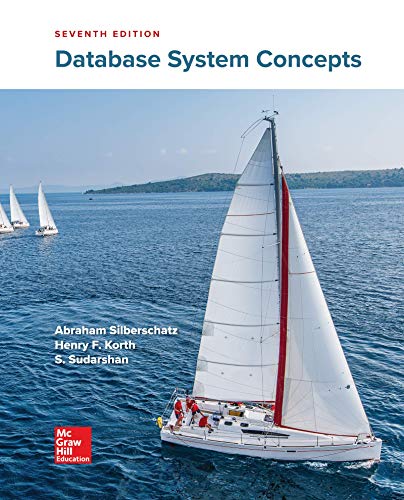
I need help with my java compiler program by
Generating intermediate code from the AST, such as three-address code or bytecode
import java.util.*;
public class SimpleCalculator {
private final String input;
private int position;
private boolean hasDivByZero = false;
private Map<String, String> symbolTable;
public SimpleCalculator(String input) {
this.input = input;
this.position = 0;
this.symbolTable = new HashMap<>();
}
public static void main(String[] args) {
SimpleCalculator calculator = new SimpleCalculator("3 + 5 * (2 - 1)");
int result = calculator.parseExpression();
if (calculator.hasDivByZero) {
System.out.println("Error: division by zero");
} else {
System.out.println("Result: " + result);
}
}
public int parseExpression() {
int value = parseTerm();
while (true) {
if (consume('+')) {
value += parseTerm();
} else if (consume('-')) {
value -= parseTerm();
} else {
break;
}
}
return value;
}
public int parseTerm() {
int value = parseFactor();
while (true) {
if (consume('*')) {
value *= parseFactor();
} else if (consume('/')) {
int divisor = parseFactor();
if (divisor == 0) {
hasDivByZero = true;
break;
}
value /= divisor;
} else {
break;
}
}
return value;
}
public int parseFactor() {
if (consume('(')) {
int value = parseExpression();
consume(')');
return value;
}
StringBuilder sb = new StringBuilder();
while (Character.isDigit(peek())) {
sb.append(consume());
}
String variable = sb.toString();
if (symbolTable.containsKey(variable)) {
String type = symbolTable.get(variable);
if (!type.equals("int")) {
System.out.println("Error: " + variable + " is not an integer");
}
} else {
System.out.println("Error: " + variable + " is not defined");
}
return Integer.parseInt(variable);
}
private char consume() {
return input.charAt(position++);
}
private boolean consume(char c) {
if (peek() == c) {
position++;
return true;
}
return false;
}
private char peek() {
return position < input.length() ? input.charAt(position) : '\0';
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Change Java code below so that it outputs a 9x9 board and not a 3x3 board. import java.util.*; public class Tic_Tac_Toe{ static String[] board;static String turn;static String checkWinner(){for (int a = 0; a < 8; a++) {String line = null; switch (a) {case 0:line = board[0] + board[1] + board[2];break;case 1:line = board[3] + board[4] + board[5];break;case 2:line = board[6] + board[7] + board[8];break;case 3:line = board[0] + board[3] + board[6];break;case 4:line = board[1] + board[4] + board[7];break;case 5:line = board[2] + board[5] + board[8];break;case 6:line = board[0] + board[4] + board[8];break;case 7:line = board[2] + board[4] + board[6];break;}//For X winnerif (line.equals("XXX")) {return "X";} // For O winnerelse if (line.equals("OOO")) {return "O";}} for (int a = 0; a < 9; a++) {if (Arrays.asList(board).contains(String.valueOf(a + 1))) {break;}else if (a == 8) {return "draw";}} // To enter the X Or O at the exact place on board.System.out.println(turn + "'s turn; enter…arrow_forwardjavascript Need help defining a function frequencyAnalysis that accepts a string of lower-case letters as a parameter. frequencyAnalysis should return an object containing the amount of times each letter appeared in the string. example frequencyAnalysis('abca'); // => {a: 2, b: 1, c: 1}arrow_forwardimport java.util.Scanner;public class MP2{public static void main (String[] args){//initialization and declarationScanner input = new Scanner (System.in);String message;int Option1;int Option2;int Quantity;int TotalBill;int Cash;int Change;final int SPECIALS = 1;final int BREAKFAST = 2;final int LUNCH = 3;final int SANDWICHES = 4;final int DRINKS = 5;final int DESSERTS = 6;//For the Special, Breakfast, Lunch Optionsfinal int Meals1 = 11;final int Meals2 = 12;final int Meals3 = 13;final int Meals4 = 14;//First menu and First OptionSystem.out.println("\t Welcome to My Kitchen ");System.out.println("\t\t SPECIALS");System.out.println("\t\t [2] BREAKFAST MEALS");System.out.println("\t\t [3] LUNCH MEALS");System.out.println("\t\t [4] SANDWICHES");System.out.println("\t\t [5] DRINKS");System.out.println("\t\t [6] DESSERTS");System.out.println("\t\t [0] EXIT");System.out.print("Choose an option ");Option1 = input.nextInt();//first switch case statementsswitch(Option1){case (SPECIALS):message…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
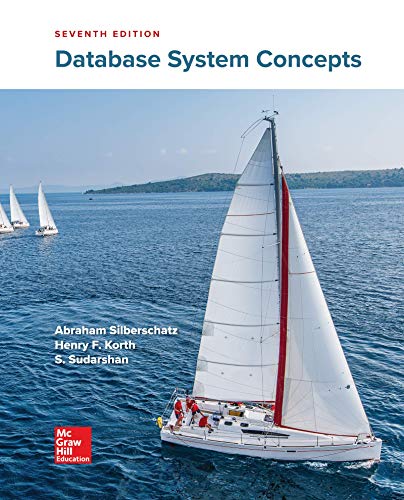
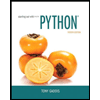
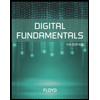
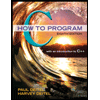
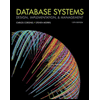
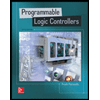