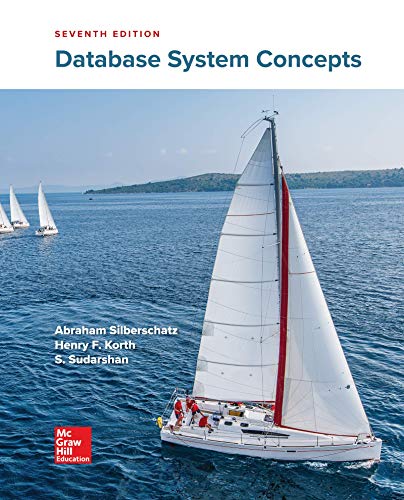
Concept explainers
I wrote three different types of code (gate level modeling, dataflow level modeling and behavioral modeling).
below I have attached the three codes hoping you could write a comment on each line of code describing what each line does and then explain how each code is different.
(Gate level modeling)
module project_gate1 (q, qbar, s, r, clk ); //(EXAMPLE COMMENTS WOULD GO HERE FOR EACH LINE)
/this is to model a SR Flip-flop in gate level modeling
input s, r, clk;
output q, qbar;
wire nand1_out;
wire nand2_out;
nand(nand1_out, s, clk);
nand(nand2_out, r, clk);
nand(q, nand1_out, qbar);
nand(qbar, nand2_out, q);
endmodule
(Data flow modeling)
module srff_dataflow(q, qbar, s, r, clk);
output q, qbar;
input s, r, clk;
wire s_bar, r_bar;
assign s_bar = ~(s & clk & qbar);
assign r_bar = ~(r & clk & q);
assign q = s_bar;
assign qbar = r_bar;
endmodule
(Behavioral level modeling)
module srff_07 (s, r, clk, q, qbar);
input s, r, clk;
output q, qbar;
reg q, qbar;
always@(posedge clk)
begin
if (s == 1 && r == 0)
begin
q <= 1;
qbar <= 0;
end
else if (s == 0 && r == 1)
begin
q <= 0;
qbar <= 1;
end
else if (s==0 && r==0)
begin
q <= q;
qbar <= qbar;
end
end

Gates can be defined in such a way that they are electronic devices or logical components that perform basic logical functions based on Boolean algebra.
Step by stepSolved in 6 steps

- I'm asking for help with different perspectives on purpose. Also, you do not have to complete the code, I’m posting the entire question in order for the question to be clear. Sorry if you see this question ask more than once. Objectives: The main objective of this assignment is to assess the student’s ability to provide a complete Black-Box testing plan for a computer program. Description: you will need to design a complete Black-Box testing plan for the following program. Instead of changing passwords every semester, students will now change passwords once a month. Unfortunately, the school has realized that many students are bad at picking their passwords. Ideally, passwords alternate between letters, numbers, and symbols. However, most students and faculty pick passwords such as “12345password”. To make matters worse, when students and faculty are forced to change their password, usually only a single character is added to the end of their previous password. Due to this…arrow_forwardMatlab coding pleasearrow_forwardOverview In this assignment, you will gain more practice with designing a program. Specifically, you will create pseudocode for a higher/lower game. This will give you practice designing a more complex program and allow you to see more of the benefits that designing before coding can offer. The higher/lower game will combine different programming constructs that you have been learning about, such as input and output, decision branching, and a loop. Higher/Lower Game DescriptionYour friend Maria has come to you and said that she has been playing the higher/lower game with her three-year-old daughter Bella. Maria tells Bella that she is thinking of a number between 1 and 10, and then Bella tries to guess the number. When Bella guesses a number, Maria tells her whether the number she is thinking of is higher or lower or if Bella guessed it. The game continues until Bella guesses the right number. As much as Maria likes playing the game with Bella, Bella is very excited to play the game…arrow_forward
- Language is C++ Lab14A: The Architect. Buildings can be built in many ways. Usually, the architect of the building draws up maps and schematics of a building specifying the building’s characteristics such as how tall it is, how many stories it has etc. Then the actual building itself is built based on the schematics (also known as blueprints). Now it is safe to assume that the actual building is based off the blueprint but is not the blueprint itself and vice versa. The idea of a classes and objects follows a similar ideology. The class file can be considered the blueprint and the object is the building following the analogy mentioned above. The class file contains the details of the object i.e., the object’s attributes (variables) and behavior (methods). Please keep in mind that a class is a template of an eventual object. Although the class has variables, these variables lack an assigned value since each object will have a unique value for that variable. Think of a form that you…arrow_forwardI don't want the code, I only want the flow chart Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Each one of the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Project Specifications Input for this project: Values of the grid (row by row) Output for this project: Whether or not the grid is a magic square Programmer’s full name Project number Project due date Processing Requirements Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number // Function prototypes bool isMagicSquare(int…arrow_forwardDraw the hierarchy chart and design the logic for a program that calculates service charges for Hazel's Housecleaning service. The program contains housekeeping, detail loop, and end-of-job modules. The main program declares any needed global variables and constants and calls the other modules. The housekeeping module displays a prompt for and accepts a customer's last name. While the user does not enter ZZZZ for the name, the detail loop accepts the number of bathrooms and the number of other rooms to be cleaned. The service charge is computed as $40 plus $15 for each bathroom and $10 for each of the other rooms. The detail loop also displays the service charge and then prompts the user for the next customer's name. The end-of-job module, which executes after the user enters the sentinel value for the name, displays a message that indicates the program is complete.arrow_forward
- Use the correct Syntax In python You just started your summer internship with old jalopy auto Rentals based in Lenior. North Carolina. You will be working in a large development team in the process of building the firm's first online reservation system. your first assignment is to build the components that will check the age of the customer and then assign them a car. you will be building the business logic classes, so you do not have to worry about the forms of communicating with the user or data access classes to communicate with the database. To test your classes, you will use them in a test application. The requirements for your classes and the testing application are as follows: -You will design and build 2 components of the online reservation system(ORS). There will be one class to represent customers and another to represent vehicles. -Each class should have at least 2 members and 2 methods -The ORS will need to be able to check to make sure the customer in question is at least…arrow_forwardA manager of a department at Google wants to schedule 15-min coffee chat between two employees every week for his 6 employees. Design and write a program to help this manager. Each employee should have a meeting with a new person. So, no meeting with the same person until they meet all the other colleagues. Use functional programming or object-oriented programing. You can have your own design to cover the requirements but you should consider a design with better time complexity. You can use any data structures (lists, dictionaries, stacks, queues, ...) The number 6 here is an example and imaging there are "n" (an even number) employees in the group (for this example n =6) Every week each employee should have a meeting and just one meeting with one person. Every week they should have a meeting with a new person. Until week 5 ( n-1 weeks) they should have a meeting with the same person. employees = [ employee_1, employee_2, employee_3, employee_4, employee_5, employee_6] It means…arrow_forwardPlease do it Yourself.You are supposed to write an SRS document of the system scenario given in the file named “Smart health system”. In the file the information about the system and its module which are to be made are discussed. You are supposed to make an SRS document of that system by writing only the first three sections of the SRS document. The File: Read it Smart Health Monitoring SystemAs the name states Smart Health Monitoring System is a smart AI that calculates almost everything you need to take care of your health. The System helps with-BMI Level,-Diet Monitoring,-Diet Suggestions,-Footstep monitoring,-Sleep Suggestions,-Water Alarm. So the user has to just register into the system by giving his personal details and physical attributes and the rest, the system takes care of everything. The Food items with its fats, carbs and proteins level are already stored in the database and the user can also add new food, which will help the system to monitor the users diet and…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
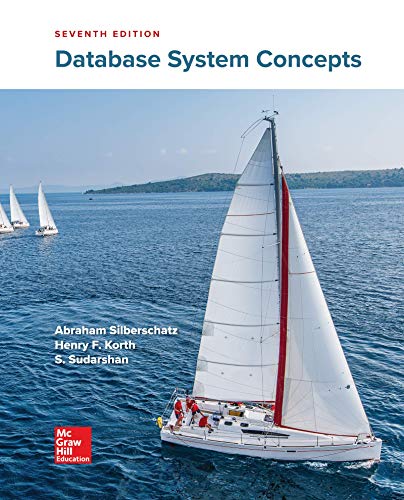
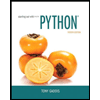
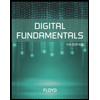
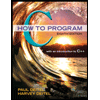
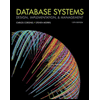
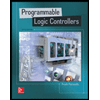