Identify the errors in the following code segment * provide the correction for each error. Import Java.util.scanner; public class Readable { public Static void main(String[] args) { Scanner KB ; int shares; double averagePrice = 14.67; Shares = KB.nextDouble(); System.out.println("There were " , shares + " shares sold at $" + averagePrice + " per share."); } }
Identify the errors in the following code segment
* provide the correction for each error.
Import Java.util.scanner;
public class Readable
{
public Static void main(String[] args)
{
Scanner KB ;
int shares;
double averagePrice = 14.67;
Shares = KB.nextDouble();
System.out.println("There were " , shares + " shares sold at $" + averagePrice + " per share.");
}
}

There are a few errors in the given code segment:
-
The package name is not written correctly. It should be
java.util.Scanner
, with a lowercase "s" in "Scanner". -
The variable
KB
is declared but not initialized. We need to create a new instance ofScanner
to read input from the user. -
The variable
Shares
is declared with an uppercase "S" which is not the same as the lowercase "shares" variable used later in the program. Java is case-sensitive, so these two variables are different and cannot be used interchangeably. -
The
println
statement has a syntax error. It should use the+
operator to concatenate the strings and variables together.
Here's the corrected code:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

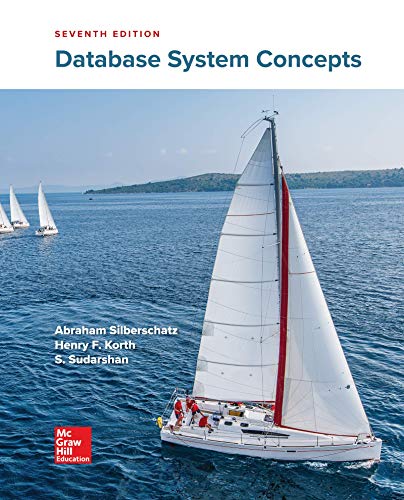
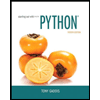
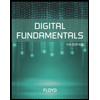
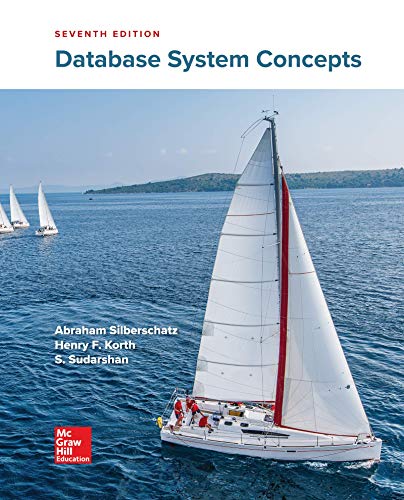
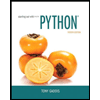
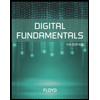
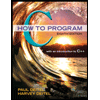
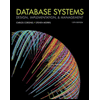
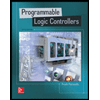