I'm having trouble with this Java coding. There are errors in my code but I don't know how to resolve them. //Import Scanner class import java.util.Scanner; //Import Random class import java.util.Random; //Driver class/program public class ProcessingArrays{ //Main method public static void main(String[] args){ //Create new instance of Scanner object Scanner keyboard = new Scanner(System.in); //Ask user for number of double values System.out.println("How many double numerical entries do you have?\nenter in digits ex: 3"); //Declare variable sizeOfArray of type double //Save user input sizeOfArray int sizeOfArray; sizeOfArray = keyboard.nextInt(); //Create array of size sizeOfArray double[] randomArray; randomArray = new double[sizeOfArray]; //Create new instance of Random object Random rand = new Random(); /*Use for loop to generate and assign random double values to randomArray*/ for(int i = 0; i < sizeOfArray; i++){ randomArray[i] = rand.nextDouble(); } //Invoke sumOfArrayValues method sumOfArrayValues(double[] randomArray); //Invoke aveMaxMin method aveMaxMin(double[] randomArray); } //Sum method with an array as input and a double as output public static void sumOfArrayValues(double[] randomArray){ //Declare variable sum of type double double sum = 0; //Create for loop to sum the values of the array for (int i = 0; i < randomArray.length; i++){ sum += randomArray[i]; } //Print the number of entries System.out.println("The number of entries in the array is: " + randomArray.length); //Print the sum of the entries System.out.println(sum); } //Average, Maximum, Minimum Method public static void aveMaxMin(double[] randomArray){ //Declare variables of type double: sum, average, max, and min double sum = 0; double average = 0; double max = 0; double min = 0; //Use a for loop to calculate the sum of the array values for (int i = 0; i < randomArray.length; i++){ sum += randomArray[i]; //Use if, else-if statements to find min and max values if (randomArray[i + 1] > randomArray[i]){ max = randomArray[i + 1]; } else if (randomArray[i + 1] < randomArray[i]){ min = randomArray[i + 1]; } } //Calculate and print average of array values average = (sum / randomArray.length); System.out.println(average); //Print max and min values System.out.println("The maximum value in the array is: " + max); System.out.println("The minimum value in the array is: " + min); } } The errors are: (See image attached)
I'm having trouble with this Java coding. There are errors in my code but I don't know how to resolve them.
//Import Scanner class
import java.util.Scanner;
//Import Random class
import java.util.Random;
//Driver class/program
public class ProcessingArrays{
//Main method
public static void main(String[] args){
//Create new instance of Scanner object
Scanner keyboard = new Scanner(System.in);
//Ask user for number of double values
System.out.println("How many double numerical entries do you have?\nenter in digits ex: 3");
//Declare variable sizeOfArray of type double
//Save user input sizeOfArray
int sizeOfArray;
sizeOfArray = keyboard.nextInt();
//Create array of size sizeOfArray
double[] randomArray;
randomArray = new double[sizeOfArray];
//Create new instance of Random object
Random rand = new Random();
/*Use for loop to generate and assign random
double values to randomArray*/
for(int i = 0; i < sizeOfArray; i++){
randomArray[i] = rand.nextDouble();
}
//Invoke sumOfArrayValues method
sumOfArrayValues(double[] randomArray);
//Invoke aveMaxMin method
aveMaxMin(double[] randomArray);
}
//Sum method with an array as input and a double as output
public static void sumOfArrayValues(double[] randomArray){
//Declare variable sum of type double
double sum = 0;
//Create for loop to sum the values of the array
for (int i = 0; i < randomArray.length; i++){
sum += randomArray[i];
}
//Print the number of entries
System.out.println("The number of entries in the array is: " + randomArray.length);
//Print the sum of the entries
System.out.println(sum);
}
//Average, Maximum, Minimum Method
public static void aveMaxMin(double[] randomArray){
//Declare variables of type double: sum, average, max, and min
double sum = 0;
double average = 0;
double max = 0;
double min = 0;
//Use a for loop to calculate the sum of the array values
for (int i = 0; i < randomArray.length; i++){
sum += randomArray[i];
//Use if, else-if statements to find min and max values
if (randomArray[i + 1] > randomArray[i]){
max = randomArray[i + 1];
}
else if (randomArray[i + 1] < randomArray[i]){
min = randomArray[i + 1];
}
}
//Calculate and print average of array values
average = (sum / randomArray.length);
System.out.println(average);
//Print max and min values
System.out.println("The maximum value in the array is: " + max);
System.out.println("The minimum value in the array is: " + min);
}
}
The errors are: (See image attached)
![ProcessingArrays.java:43: error: '.class' expected
sumOfArrayValues(double[] randomArray);
ProcessingArrays.java:43: error: ';' expected
sumOfArrayValues(double[] randomArray);
ProcessingArrays.java:46: error: '.class' expected
aveMaxMin(double[] randomArray);
ProcessingArrays.java:46: error: ';' expected
aveMaxMin (double[] randomArray);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faac597e2-e0e7-469d-a457-aa5da7321546%2F07c9c0fa-bda2-4224-a704-ea4f14c54ef0%2F9rcrnkf_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

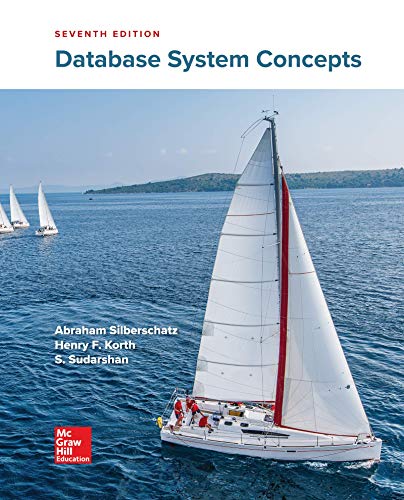
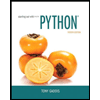
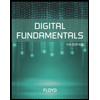
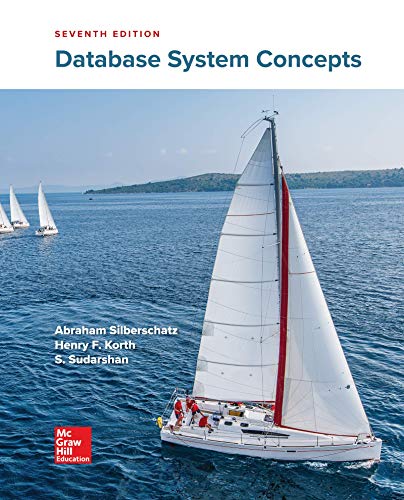
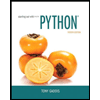
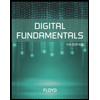
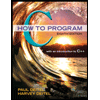
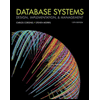
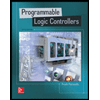