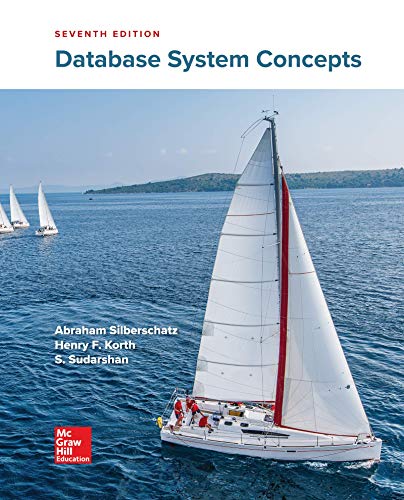
Implement in C
9.10.1: LAB: Movie show time display
Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements:
- Each row contains the title, rating, and all showtimes of a unique movie.
- A space is placed before and after each vertical separator ('|') in each row.
- Column 1 displays the movie titles and is left justified with a minimum of 44 characters.
- If the movie title has more than 44 characters, output the first 44 characters only.
- Column 2 displays the movie ratings and is right justified with a minimum of 5 characters.
- Column 3 displays all the showtimes of the same movie, separated by a space.
Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows.
Hints: Use the fgets() function to read each line of the input text file. When extracting texts between the commas, copy the texts character-by-character until a comma is reached. A string always ends with a null character ('\0').
Ex: If the input of the program is:
movies.csv
and the contents of movies.csv are:
16:40,Wonders of the World,G 20:00,Wonders of the World,G 19:00,Journey to Space ,PG-13 12:45,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 15:00,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 19:30,Buffalo Bill And The Indians or Sitting Bull's History Lesson,PG 10:00,Adventures of Lewis and Clark,PG-13 14:30,Adventures of Lewis and Clark,PG-13 19:00,Halloween,R
the output of the program is:
Wonders of the World | G | 16:40 20:00 Journey to Space | PG-13 | 19:00 Buffalo Bill And The Indians or Sitting Bull | PG | 12:45 15:00 19:30 Adventures of Lewis and Clark | PG-13 | 10:00 14:30 Halloween | R | 19:00
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
int main(void) {
const int MAX_TITLE_CHARS = 44; // Maximum length of movie titles
const int LINE_LIMIT = 100; // Maximum length of each line in the text file
char line[LINE_LIMIT];
char inputFileName[25];
/* Type your code here. */
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardWhat are the values of the variable number and the vector review after the following code segment is executed? vector<int> review(10,-1); int number; number = review[0]; review[1] = 150; review[2] = 350; review[3] = 450; review.push_back(550); review.push_back(650); number += review[review.size()-1]; review.pop_back();arrow_forwardCreate a structure called applianceTvpe with the following members. Use the proper variable TYPES for the members: name; SUpplierlD: modelNo: cost; Create an array variable of type applianceType that can hold 50 components. Write the code segment to read from a file into the structure array. Declare an input file stream identifier for this purpose. Open the file and read until end of file. Keep a count of how many rows actually read. The data file contains the following data: RefrigeratorABC 80 4657 521.62 ToasterOven 100 3245 245.96 MicroWave 95 7878 345.67 Using a looping construct, output to the console (cout) the data read into the structure array. Make sure you do not go outside the bounds of the array or beyond the number of rows actually read. You do not need to write any #include statements, using statements and you do not have to write code to open the file. Assume the file is open. You do need to declare variables needed for the program segment.arrow_forward
- First programming homework Create a class of records for a gradebook called Rec. The data should be private, and should include a firstname, lastname, array of three grades, and a field for average grade. Build two constructors: a default constructor and a constructor that takes the first and last name. Build a function to read the data, either from a file or from cin. The read function reads the two names and three grades, but does not read the average grade. Build a write function that writes the data either to a file or to cout. The write function prints all of the data on one line with spaces between fields. Build a function to calculate the average grade field. Build an overloaded operator == to compare two records. This should be implemented as a friend function. Declare the constructors and functions in the body of the class, but implement them outside the class. I will provide you with a driver called hw1.cpp in ~cthorpe/public/142 and with a test file HW1.txt in the same…arrow_forwardIn Python Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program is: movies.csv and the contents…arrow_forwardC++ Add a search command that asks the user for a string and finds the first line that contains that string. The search starts at the first line currently displayed and stops at the end of the file. If the string is found, the line that contains it is displayed at the top. In case the user enters a string X that does not occur in the file, the program should print the error message: ERROR: string "X" was not found. Attached is the first lines of the .txt filearrow_forward
- C++ Add a search command that asks the user for a string and finds the first line that contains that string. The search starts at the first line currently displayed and stops at the end of the file. If the string is found, the line that contains it is displayed at the top. In case the user enters a string X that does not occur in the file, the program should print the error message: ERROR: string "X" was not found.arrow_forwardComputer Science csv file "/dsa/data/all_datasets/texas.csv" Task 6: Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols). Hint: function(); $county ==; plot(); add=TRUE.arrow_forwardDescriptionWrite a program to compute average grades for a course. The course records are in a single file and are organized according to the following format: each line contains a student's first name, then one space, then the student's last name, then one space, then some number of quiz scores that, if they exist, are separated by one space. Each student will have zero to ten scores, and each score is an integer not greater than 100. Your program will read data from this file and write its output to a second file. The date in the output file will be nearly the same as the data in the input file except that you will print the names as last-name, first-name; each quiz score, and there will be one additional number at the end of each line:the average of the student's ten quiz scores.Both files are parameters. You can access the name of the input file with argv[1]. and the name of the output file with argv[2].The output file must be formatted as described below: 1. First and last names…arrow_forward
- CODE SHOULD BE PYTHON:arrow_forwardJAVA 8.13 LAB: Movie show time display Write a program that reads movie data from a csv (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the csv file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator (|) in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the csv file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Ex: If the input of the program…arrow_forwardC++ beginners.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
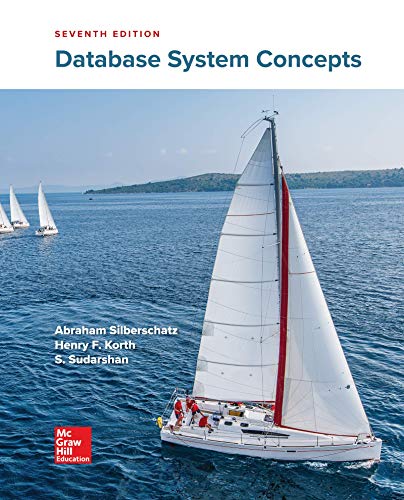
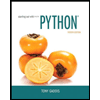
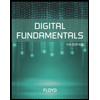
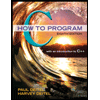
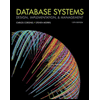
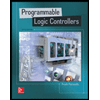