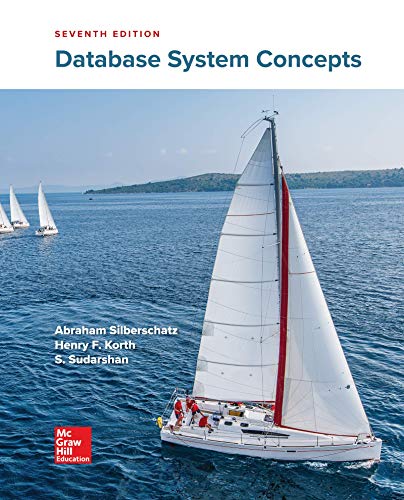
Implement in C Programming
9.5.1: LAB: Parsing dates
Complete main() to read dates from input, one date per line. Each date's format must be as follows: March 1, 1990. Any date not following that format is incorrect and should be ignored. Use the substring() function to parse the string and extract the date. The input ends with -1 on a line alone. Output each correct date as: 3-1-1990.
Ex: If the input is:
March 1, 1990 April 2 1995 7/15/20 December 13, 2003 -1
then the output is:
3-1-1990 12-13-2003
Use the provided GetMonthAsInt() function to convert a month string to an integer. If the month string is valid, an integer in the range 1 to 12 inclusive is returned, otherwise 0 is returned. Ex: GetMonthAsInt("February") returns 2 and GetMonthAsInt("7/15/20") returns 0.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- In pythonarrow_forward1 - Write a Python function that will do the following: 1. Ask the user to input an integer that will be appended to a list that was originally empty. This will be done 5 times, meaning that when the input is complete, the list will have five elements (all integers). a. Determine whether each element is an even number or an odd number b. Return a list of five string elements "odd" and "even" that map the indexes of the elements of the input list, according to whether these elements are even or odd numbers.For example: if the input sequence is 12, 13, 21, 51, and 30, the function will return the list ["even", "odd", "odd", "odd", "even"].arrow_forwardpython: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter, Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "Luna Lovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger, Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "James Potter, Gryffindor"])['Hermione Granger, Gryffindor',…arrow_forward
- Write a function that will accept a list of integers and return the sum of the contents of the list(……if the list contains 1,2,3 it will return 6…..)arrow_forwardpython: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "LunaLovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger,Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "JamesPotter, Gryffindor"])['Hermione Granger, Gryffindor', 'James…arrow_forwardplease solve in python and give code. thankzzarrow_forward
- Given a file of unsorted words with mixed case: read the entries in the file and sort those words lexicographically. The program (in c++) should then prompt the user for an index, and display the word at that index. Since you must store the entire list in an array, you will need to know the length. The "List of 1000 Mixed Case Words" contains 1000 words. You are guaranteed that the words in the array are unique, so you don't have to worry about the order of, say, "bat" and "Bat."arrow_forwardC++arrow_forwardThe function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forward
- In c++ and without the use of vectors, please. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3…arrow_forwardIn c++ and please without the use of vectors. Thanks very much! A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use linear search. Modify the algorithm to output the count of how many comparisons were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Joe 123-5432 Linda 983-4123…arrow_forwardWrite a function count_evens(nums) that takes a list of ints called nums and returns the number of even ints in the given list. Note: the % "mod" operator computes the remainder, e.g. 5 % 2 is 1.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
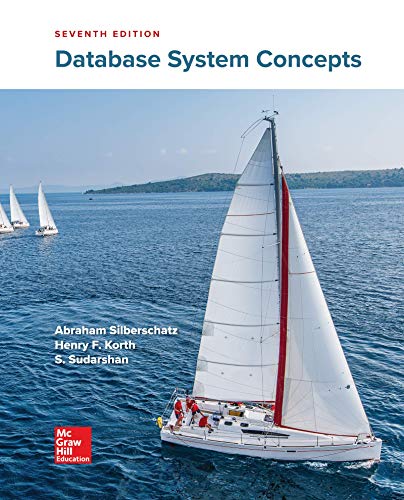
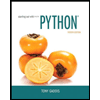
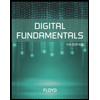
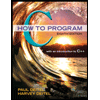
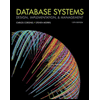
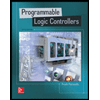