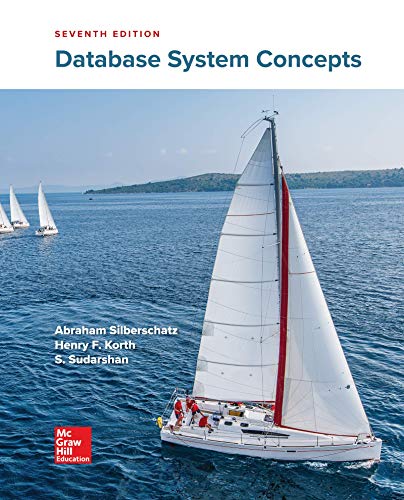
*JAVA*
complete method
Delete the largest value
removeMax();
Delete the smallest value
removeMin();
class BinarHeap<T> {
int root;
static int[] arr;
static int size;
public BinarHeap() {
arr = new int[50];
size = 0;
}
public void insert(int val) {
arr[++size] = val;
bubbleUP(size);
}
public void bubbleUP(int i) {
int parent = (i) / 2;
while (i > 1 && arr[parent] > arr[i]) {
int temp = arr[parent];
arr[parent] = arr[i];
arr[i] = temp;
i = parent;
}
}
public int retMin() {
return arr[1];
}
public void removeMin() {
}
public void removeMax() {
}
public void print() {
for (int i = 0; i <= size; i++) {
System.out.print( arr[i] + " ");
}
}
}
public class BinarH {
public static void main(String[] args) {
BinarHeap Heap1 = new BinarHeap();
Heap1.insert(12);
Heap1.insert(9);
Heap1.insert(24);
Heap1.insert(4);
Heap1.insert(71);
// print inorder traversal of the BH
System.out.printf("My Binar Heap:%n");
Heap1.print();
System.out.printf("%n%nCall Min:%n[%d]", Heap1.retMin());
System.out.printf("%n%nAfter remove Min:%n");
Heap1.removeMin();
Heap1.print();
System.out.printf("%n%nAfter remove Max:%n");
Heap1.removeMax();
Heap1.print();
}
}

Step by stepSolved in 3 steps with 5 images

- 1. Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: capacity and size are 0, array pointer is nullptr user constructor: create a dynamic array of the specified size c. overloaded operators: subscript operator: return an element or exits if illegal index d. "the big three": copy constructor: construct an IntArr object using deep copy assignment overload operator: deep copy from one object to another destructor: destroys an object without creating a memory leak e. grow function: "grow" the array to twice its capacity f. push_back function: add a new integer to the end of the array g. pop_back function: remove the last element in the array h. getSize function: return the current size of the array (not capacity) i. Use the provided main function (next page) and output example Notes a. grow…arrow_forwardpublic class arrayOutput { public static void main (String [] args) { final int NUM_ELEMENTS = 3; int[] userVals = new int [NUM_ELEMENTS]; int i; int sumVal; userVals [0] = 3; userVals [1] = 5; userVals [2] = 8; Type the program's output sumVal = 0; for (i = 0; i < userVals.length; ++i) { sumVal= sumVal + userVals [i]; System.out.println (sumVal); } ? ? ??arrow_forwardclass temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; I need help writing the definition of the member function set so the instance varialbes are set according to the parameters. I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.arrow_forward
- using System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } This code is supposed to count from 1-88 and then select three random numbers from the list but instead it generates an infinite loop of random numers between 1-88. how can it be fixed?arrow_forwardC PROGRAMMING LANGUAGEarrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forward
- #include <iostream>using namespace std;class st{private:int arr[100];int top;public:st(){top=-1;}void push(int ItEM) {top++;arr[top]=ItEM; }bool ise() {return top<0;} int pop(){int Pop;if (ise()) cout<<"Stack is emptye ";else{Pop=arr[top];top--;return Pop;}}int Top(){int TOP;if (ise()) cout<<" empty ";else {TOP=arr[top];return TOP;}}void screen(){ for (int i = 0; i <top+1 ; ++i) {cout<<arr[i];} }};int main() {st c;c.push(1);c.push(2);c.push(3);c.push(4);c.pop();c.push(5);c.screen();return 0;} alternative to this code??arrow_forwardAjva.arrow_forwardDon't delete the code. Fix the error and make sure it runs. #include using namespace std; class Puzzle { public: char arr[4][4] // constructor Puzzle() { char arr[4][2] = {'f', 'k', 's','s'} {'b', 'j', 'o','u'} {'b', 'o', 'b', 'n'} {'x', 'y', 'w', 'y'} } // destructor ~Puzzle() { } // print method void print() { for (int i=0; i> word; if (p.search(word)) cout << "The word is found in the puzzle." << endl; else cout << "The word is not found in the puzzle." << endl; return 0; }arrow_forward
- The following program asks the user to enter two numbers. What is the output of the program if the user enters 12 and 14? #include <iostream> using namespace std; void func1(int &, int &);void func2(int &, int &, int &); void func3(int, int, int); int main() { int x = 0, y = 0, z = 0; cout << x << " " << y << " " << z << endl; func1(x, y);cout << x << " " << y << " " << z << endl; func2(x, y, z); cout << x << " " << y << " " << z << endl; func3(x, y, z);cout << x << " " << y <<" " << z << endl; return 0; } void func1(int &a, int &b) { cout << "Enter two numbers: "; cin >> a >> b; } void func2(int &a, int &b, int &c) { b++;c−−;a = b + c; } void func3(int a, int b, int c) { a = b − c; }arrow_forwardclass TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forward#include <stdio.h> int arrC[10] = {0}; int bSearch(int arr[], int l, int h, int key); int *joinArray(int arrA[], int arrB[]) { int j = 0; if ((arrB[0] + arrB[4]) % 5 == 0) { arrB[0] = 0; arrB[4] = 0; } for (int i = 0; i < 5; i++) { arrC[j++] = arrA[i]; if (arrB[i] == 0 || (bSearch(arrA, 0, 5, arrB[i]) != -1)) { continue; } else arrC[j++] = arrB[i]; } for (int i = 0; i < j; i++) { int temp; for (int k = i + 1; k < j; k++) { if (arrC[i] > arrC[k]) { temp = arrC[i]; arrC[i] = arrC[k]; arrC[k] = temp; } } } for (int i = 0; i < j; i++) { printf("%d ", arrC[i]); } return arrC; } int bSearch(int arr[], int l, int h, int key) { if (h >= l) { int mid = l + (h - l) / 2; if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
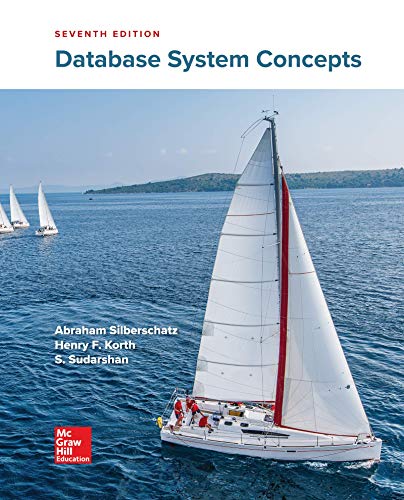
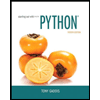
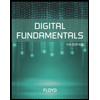
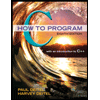
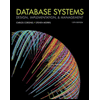
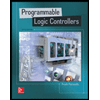