import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner console = new Scanner(System.in); programIntro(); double ExamScore1 = getExamScores(console, 1); double GPAScore1 = getGPAInformation(console); double ExamScore2 = getExamScores(console, 2); double GPAScore2 = getGPAInformation(console); double candidate1 = finalScore(ExamScore1, GPAScore1); double candidate2 = finalScore(ExamScore2, GPAScore2); System.out.println("First applicant overall score = " + String.format("%.14f", candidate1)); System.out.println("Second applicant overall score = " + String.format("%.14f", candidate2)); compareApplicants(candidate1, candidate2); } public static void programIntro() { System.out.println("This program compares two applicants to determine which one seems like the stronger applicant."); System.out.println("For each candidate I will need either SAT or ACT scores plus a weighted GPA."); } public static double getExamScores(Scanner console, int applicantNo) { String appNo = applicantNo == 1 ? "first" : "second"; System.out.println("Information for the " + appNo + " applicant:"); System.out.printf("do you have 1) SAT scores or 2) ACT scores? \033[0;4m"); int option = console.nextInt(); System.out.print("\033[0;0m"); double score; if (option == 1) { score = getSATInformation(console); } else { score = getACTInformation(console); } return score; } public static double getSATInformation(Scanner console) { System.out.printf("SAT math? \033[0;4m"); double score = console.nextInt(); System.out.print("\033[0;0m"); System.out.print("SAT verbal? \033[0;4m"); score += console.nextInt() * 2; System.out.print("\033[0;0m"); return score / 24.0; } public static double getACTInformation(Scanner console) { System.out.print("ACT English? " + "\033[0;4m"); double score = console.nextInt(); System.out.print("\033[0;0m"); System.out.print("ACT math? \033[0;4m"); score += console.nextInt(); System.out.print("\033[0;0m"); System.out.print("ACT reading? \033[0;4m"); score += console.nextInt()* 2; System.out.print("\033[0;0m"); System.out.print("ACT science? \033[0;4m"); score += console.nextInt(); System.out.print("\033[0;0m"); return score / 1.8; } public static double getGPAInformation(Scanner console) { System.out.print("overall GPA? \033[0;4m"); double GPA = console.nextDouble(); System.out.print("\033[0;0m"); System.out.print("max GPA? \033[0;4m"); double MaxGPA = console.nextDouble(); System.out.print("\033[0;0m"); double GPAScore = (GPA / MaxGPA) * 100; return GPAScore; } public static double finalScore(double ExamScore, double GPAScore) { return ExamScore + GPAScore; } public static void compareApplicants(double candidate1, double candidate2) { if (candidate1 > candidate2) { System.out.println("The first applicant seems to be better"); } else if (candidate2 > candidate1) { System.out.println("The second applicant seems to be better"); } else { System.out.println("The two applicants seem to be equal"); } } public static double roundNumber(double number) { return Math.round(number * 100.0) / 100.0; } }
Please help explain how this Java code is working
Code:
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
programIntro();
double ExamScore1 = getExamScores(console, 1);
double GPAScore1 = getGPAInformation(console);
double ExamScore2 = getExamScores(console, 2);
double GPAScore2 = getGPAInformation(console);
double candidate1 = finalScore(ExamScore1, GPAScore1);
double candidate2 = finalScore(ExamScore2, GPAScore2);
System.out.println("First applicant overall score = " + String.format("%.14f", candidate1));
System.out.println("Second applicant overall score = " + String.format("%.14f", candidate2));
compareApplicants(candidate1, candidate2);
}
public static void programIntro() {
System.out.println("This program compares two applicants to determine which one seems like the stronger applicant.");
System.out.println("For each candidate I will need either SAT or ACT scores plus a weighted GPA.");
}
public static double getExamScores(Scanner console, int applicantNo) {
String appNo = applicantNo == 1 ? "first" : "second";
System.out.println("Information for the " + appNo + " applicant:");
System.out.printf("do you have 1) SAT scores or 2) ACT scores? \033[0;4m");
int option = console.nextInt();
System.out.print("\033[0;0m");
double score;
if (option == 1) {
score = getSATInformation(console);
} else {
score = getACTInformation(console);
}
return score;
}
public static double getSATInformation(Scanner console) {
System.out.printf("SAT math? \033[0;4m");
double score = console.nextInt();
System.out.print("\033[0;0m");
System.out.print("SAT verbal? \033[0;4m");
score += console.nextInt() * 2;
System.out.print("\033[0;0m");
return score / 24.0;
}
public static double getACTInformation(Scanner console) {
System.out.print("ACT English? " + "\033[0;4m");
double score = console.nextInt();
System.out.print("\033[0;0m");
System.out.print("ACT math? \033[0;4m");
score += console.nextInt();
System.out.print("\033[0;0m");
System.out.print("ACT reading? \033[0;4m");
score += console.nextInt()* 2;
System.out.print("\033[0;0m");
System.out.print("ACT science? \033[0;4m");
score += console.nextInt();
System.out.print("\033[0;0m");
return score / 1.8;
}
public static double getGPAInformation(Scanner console) {
System.out.print("overall GPA? \033[0;4m");
double GPA = console.nextDouble();
System.out.print("\033[0;0m");
System.out.print("max GPA? \033[0;4m");
double MaxGPA = console.nextDouble();
System.out.print("\033[0;0m");
double GPAScore = (GPA / MaxGPA) * 100;
return GPAScore;
}
public static double finalScore(double ExamScore, double GPAScore) {
return ExamScore + GPAScore;
}
public static void compareApplicants(double candidate1, double candidate2) {
if (candidate1 > candidate2) {
System.out.println("The first applicant seems to be better");
} else if (candidate2 > candidate1) {
System.out.println("The second applicant seems to be better");
} else {
System.out.println("The two applicants seem to be equal");
}
}
public static double roundNumber(double number) {
return Math.round(number * 100.0) / 100.0;
}
}

Step by step
Solved in 3 steps with 4 images

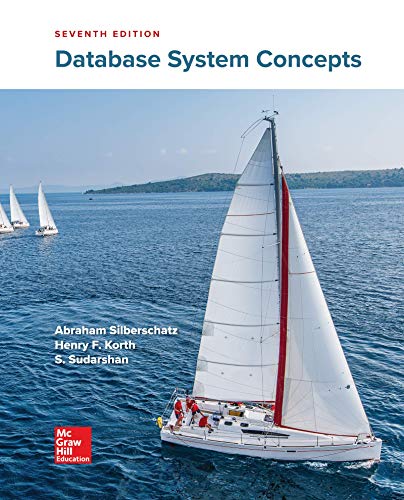
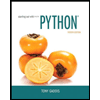
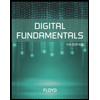
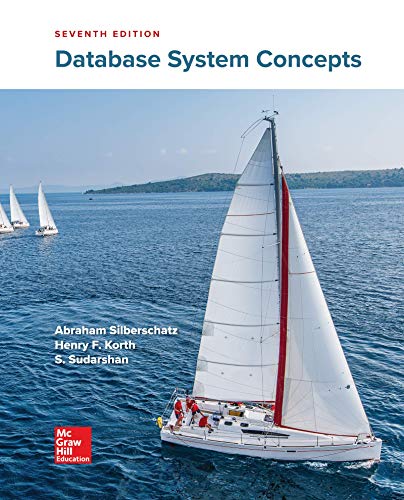
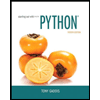
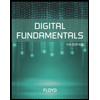
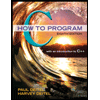
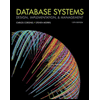
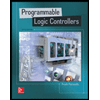