1. Modify your program from 04 Hands-on Activity 1 to apply encapsulation. Make bloodType and rhFactor non-static and private. Remove the constructor with two (2) parameters. 2. The names of the public setter and getter methods should be: • setBlood Type() • setRhFactor() • getBloodType() getRhFactor() 3. Use the setter methods to accept user input. 4. Display the values by calling the getter methods. ple Output:
import java.util.Scanner;
class BloodData {
private static String bloodType;
private static String rhFactor;
public BloodData() {
bloodType="O";
rhFactor="+";
}
public BloodData(String bt, String rh) {
bloodType = bt;
rhFactor = rh;
}
public void display() {
System.out.println(bloodType+rhFactor+" is added to the blood bank."); //prints message
}
}
public class Main {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in); //create Scanner instance
System.out.print("Enter blood type of patient: ");
String input1=sc.nextLine(); //accept input from user
System.out.print("Enter the Rhesus factor (+ or -): ");
String input2=sc.nextLine(); //accept input from user
BloodData bd; //create instance
if("".equals(input1) || "".equals(input2)) //if any of inputs is blank
bd=new BloodData(); //allocates memory using default constructor
else //if valid inputs
bd=new BloodData(input1,input2); //allocates memory using parameterized constructor
bd.display(); //invokes display method
}
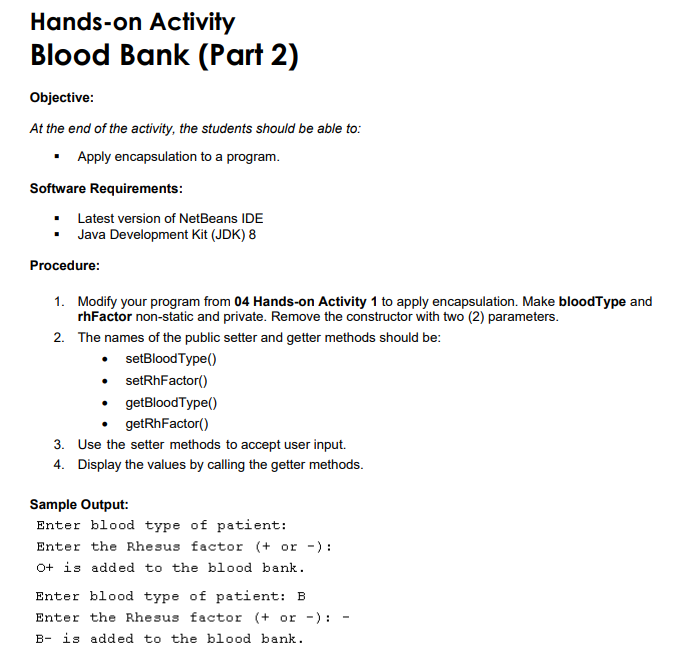

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

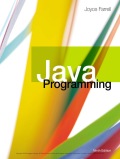
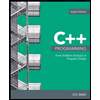
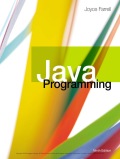
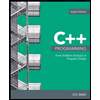