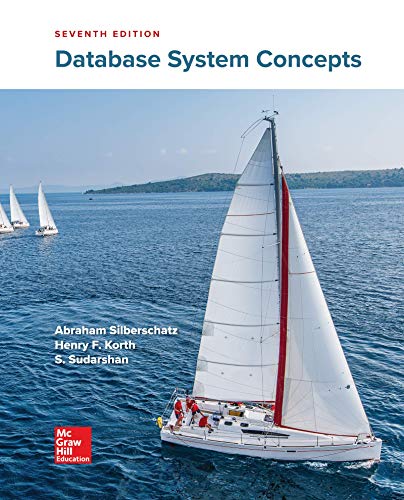
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
import java.util.Scanner;
public class MakeChange {
public static void main(String[] args) {
// Create a Scanner object for user input
Scanner scanner = new Scanner(System.in);
// Ask the user for the amount of change in dollars
System.out.print("Enter the amount of change: ");
double dollarAmount = scanner.nextDouble();
// Convert the dollar amount to cents
int cents = (int) (dollarAmount * 100);
// Calculate the total number of coins
int quarters = cents / 25;
cents %= 25;
int dimes = cents / 10;
cents %= 10;
int nickels = cents / 5;
cents %= 5;
int pennies = cents / 1;
cents %= 1;
// Calculate the total number of coins
int totalCoins = quarters + dimes + nickels + pennies;
System.out.println("Change due is: $"+ dollarAmount);
// Output the total number of coins
System.out.println("This is following "+ totalCoins+" coins: ");
// Output the individual coin counts
System.out.println("Quarters: " + quarters);
System.out.println("Dimes: " + dimes);
System.out.println("Nickels: " + nickels);
System.out.println("Pennies: " + pennies);
// Close the scanner
scanner.close();
}
}
when i run the program it doesn't count 4 pennies when i enter 0.29, why is that?
Expert Solution

arrow_forward
Step 1: Introduction to the cause of the error
The issue you're encountering in your program is related to floating-point precision when converting dollar amounts to cents. Floating-point numbers like double
in Java can sometimes lead to small precision errors, which can affect the results of calculations. When you enter 0.29 dollars, the conversion to cents may not yield exactly 29 cents due to these precision issues.
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- public class Side Plot { * public static String PLOT_CHAR = "*"; public static void main(String[] args) { plotxSquared (-6,6); * plotNegXSquaredPlus20 (-5,5); plotAbsXplus1 (-4,4); plotSinWave (-9,9); public static void plotXSquared (int minX, int maxx) { System.out.println("Sideways Plot"); System.out.println("y = x*x where + minX + "= minX; row--) { for (int col = 0; col row * row; col++) { System.out.print (PLOT_CHAR); if (row== 0){ } System.out.print(" "); } for(int i = 0; i <= maxx* maxX; i++) { System.out.print("-"); } System.out.println(); Sideways PHOT y = x*x where -6<=x<=6 Sideways Plot y = x*x where -6<=x<=6 How do I fix my code to get a plot like 3rd picture?arrow_forwardGiven an integer, return the sum of all the odd numbers starting from 1. Assume the input will always be greater than 2. Ex: If the input is: 20 the output is 1+3+5+7+9+11+13+15+17+19: 100 449182.3196742.qx3zqy7 LAB ACTIVITY 1 import java.util.Scanner; 3 public class LabProgram { 4 5 6 7 8 9 10 13.3.1: CodingBird (Loop): Sum Even public static void main(String[] args) { /* Type your code here. */ } LabProgram.java 0/10 Load default template...arrow_forwardimport java.util.Scanner;/** This program calculates the geometric and harmonic progression for a number entered by the user.*/public class Progression{ public static void main(String[] args) { Scanner keyboard = new Scanner (System.in); System.out.println("This program will calculate " + "the geometric and harmonic " + "progression for the number " + "you enter."); System.out.print("Enter an integer that is " + "greater than or equal to 1: "); int input = keyboard.nextInt(); // Match the method calls with the methods you write int geomAnswer = geometricRecursive(input); double harmAnswer = harmonicRecursive(input); System.out.println("Using recursion:"); System.out.println("The geometric progression of " + input + " is " + geomAnswer); System.out.println("The harmonic progression of " +…arrow_forward
- public class Animal { public static int population; private int age; } public Animal (int age) { this.age = age; population++; } + msg); public void say (String msg) { System.out.print("Saying: " System.out.print(" for "); System.out.print(getHuman Years()); System.out.println(" years."); } public int getHuman Years() { return age; } public class Mammal extends Animal { private String species; public Mammal(String species, int age) { super (age); this. species species; } } } = public int getHuman Years() { if (species.equals("dog")) 1 else return super.getHumanYears() * 7; return super.getHumanYears(); €arrow_forwardimport java.util.Scanner;public class MP2{public static void main (String[] args){//initialization and declarationScanner input = new Scanner (System.in);String message;int Option1;int Option2;int Quantity;int TotalBill;int Cash;int Change;final int SPECIALS = 1;final int BREAKFAST = 2;final int LUNCH = 3;final int SANDWICHES = 4;final int DRINKS = 5;final int DESSERTS = 6;//For the Special, Breakfast, Lunch Optionsfinal int Meals1 = 11;final int Meals2 = 12;final int Meals3 = 13;final int Meals4 = 14;//First menu and First OptionSystem.out.println("\t Welcome to My Kitchen ");System.out.println("\t\t SPECIALS");System.out.println("\t\t [2] BREAKFAST MEALS");System.out.println("\t\t [3] LUNCH MEALS");System.out.println("\t\t [4] SANDWICHES");System.out.println("\t\t [5] DRINKS");System.out.println("\t\t [6] DESSERTS");System.out.println("\t\t [0] EXIT");System.out.print("Choose an option ");Option1 = input.nextInt();//first switch case statementsswitch(Option1){case (SPECIALS):message…arrow_forward8) Now use the Rectangle class to complete the following tasks: - Create another object of the Rectangle class named box2 with a width of 100 and height of 50. Note that we are not specifying the x and y position for this Rectangle object. Hint: look at the different constructors) Display the properties of box2 (same as step 7 above). - Call the proper method to move box1 to a new location with x of 20, and y of 20. Call the proper method to change box2's dimension to have a width of 50 and a height of 30. Display the properties of box1 and box2. - Call the proper method to find the smallest intersection of box1 and box2 and store it in reference variable box3. - Calculate and display the area of box3. Hint: call proper methods to get the values of width and height of box3 before calculating the area. Display the properties of box3. 9) Sample output of the program is as follow: Output - 1213 Module2 (run) x run: box1: java.awt. Rectangle [x=10, y=10,width=40,height=30] box2: java.awt.…arrow_forward
- BAGEL FILES import javax.swing.JFrame; public class Bagel{//-----------------------------------------------------------------// Creates and displays the controls for a bagel shop.//-----------------------------------------------------------------public static void main (String[] args){JFrame frame = new JFrame ("Bagel Shop");frame.setDefaultCloseOperation (JFrame.EXIT_ON_CLOSE); frame.getContentPane().add(new BagelControls()); frame.pack();frame.setVisible(true);}} import java.awt.*;import java.awt.event.*; import javax.swing.*; public class BagelControls extends JPanel{private JComboBox bagelCombo;private JButton calcButton;private JLabel cost;private double bagelCost;public BagelControls(){String[] types = {"Make A Selection...", "Plain","Asiago Cheese", "Cranberry"};bagelCombo = new JComboBox(types);calcButton = new JButton("Calc");cost = new JLabel("Cost = " + bagelCost);setPreferredSize (new Dimension (400,…arrow_forwardTASK 3. Console Input and Output. Review Scanner class and its methods, Implement the following code, make sure it runs without errors. 1 package 1lessons; 3 import java.util.Scanner; 4 5 public class ReportSum3 { public static void main(String args[]) { Scanner sc = new Scanner(System. in); 60 7 8 // Prompt the user to enter the first integer System.out.println("Enter the value for 'a' and press Enter: "); int a = sc.nextInt(); // Prompt the user to enter the second integer System.out.println("Enter the value for 'b' and press Enter: "); int b = sc.nextInt(); 10 11 12 13 14 15 16 17 18 19 20 21 22 } //Close the Scanner. It's a good practice to release the resource that was acquired sc.close(); // Display the result System.out.printf( "Provided Integers: %4d and %4d, the total is %5d\n", a, b, (a + b)); } To simplify the task, we came out with the idea to use Methods. Implement a method to add two integers.arrow_forwardpublic class Test { } public static void main(String[] args) { int a = 5; a += 5; } switch(a) { case 5: } System.out.print("5"); break; case 10: System.out.print("10"); System.out.print("0"); default:arrow_forward
- Type the program's output import java.util.Scanner; public class NumberSearch { public static void findNumber(int number, int lowVal, int highVal, String indentAmt) { int midVal; midVal = (highVal + lowVal) / 2; System.out.print(indentAmt); System.out.print(midVal); if (number == midVal) { System.out.println(" a"); } else { if (number < midVal) { System.out.println(" b"); findNumber(number, lowVal, midVal, indentAmt + " "); } else { System.out.println(" c"); findNumber(number, midVal + 1, highVal, indentAmt + " "); } } System.out.println(indentAmt + "d"); } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); int number; number = scnr.nextInt(); findNumber(number, 0, 16, ""); } } Input 0arrow_forwarduse the JCreator program for code in picture and show the outputarrow_forwardInheritanceDemo.java: // StudentName Today's Date public class InheritanceDemo { public static void main(String[] args) { KleenexBox kb1 = new KleenexBox(); KleenexBox kb2 = new KleenexBox(100); Box justABox = new Box(); System.out.println("justABox has area " + BoxArea(justABox)); System.out.println("kb1 has area " + BoxArea(kb1)); System.out.println("kb2 has this many square inches of tissue: " + KleenexArea(kb2)); } public static double BoxArea (Box b) { return b.width * b.length; } public static double KleenexArea (KleenexBox a) { return a.width * a.length * a.numTissues; } } Box.java: // Student Name Today's Date public class Box { public double width; public double length; public String label; } KleenexBox.java: // Student's Name Today's Date public class KleenexBox extends Box { public int numTissues; // A new Kleenex box public KleenexBox() { numTissues = 50; length = 8.0; width = 5.0; } // A new Kleenex box with a specific number of tissues public…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
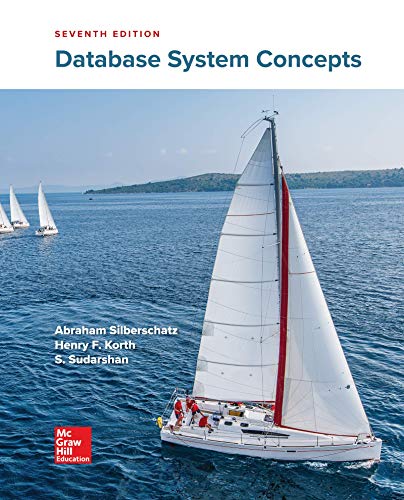
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
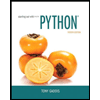
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
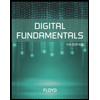
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
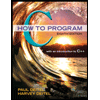
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
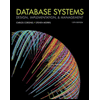
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
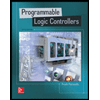
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education