import javax.swing.JOptionPane; public class MultipleChoiceQuestion { static int correct = 0; static int incorrect = 0; String question; String correctAnswer; MultipleChoiceQuestion(String query, String a, String b, String c, String d, String e, String Answer) { question = query+"\n"; question = "A. "+a+"\n"; question = "B. "+b+"\n"; question = "C. "+c+"\n"; question = "D. "+d+"\n"; question = "E. "+e+"\n"; correctAnswer = Answer.toUpperCase(); } String ask() { while (true) { String answer = JOptionPane.showInputDialog(question); answer =answer.toUpperCase(); } String ask() { while (true) { String answer = JOptionPane.showInputDialog(question); answer=answer.toUpperCase(); boolean valid = (answer.equals("A")||answer.equals("B")|| answer.equals("C")|| answer.equals("D")||answer.equals("E")); If(valid) return answer; JOptionPane.showMessageDialog(null,"Invalid answer. Please enter" A, B, C, D, or E."); } } void check() { nQuestions++; String answer = ask(); if (answer.equals(correctAnswer)) { JOptionPane.showMessageDialog(null,"Correct!"); int nCorrect = 0; nCorrect++; }else { JOptionPane.showMessageDialog(null,"Incorrect. The correct answer is + "+correctAnswer+"."); } } static void showResults() { Object nCorrect = null; Object nQuestions = null; JOptionPane.showMessageDialog(null,nCorrect +"correct out of "+ nQuestions +" questions"); } } public class Quiz { public static void main(String[] args) { MultipleChoiceQuestion question = new MultipleChoiceQuestion("What is a quiz?", "a test of knowledge, especially a brief informal test given to students", "42", "a duck", "to get to the other side", "To be or not to be, that is the question.", question.check(); question.showResults(); MultipleChoiceQuestion question1 = new MultipleChoiceQuestion("Who was the first president of the United States?", "Washington", "Jefferson", "Lincoln", "Clinton", "a"); question1.check(); question1.showResults(); MultipleChoiceQuestion question2 = new MultipleChoiceQuestion("The World Wide Web is also known?", "Digital Magician", "Information Age", "Internet", "Personal Computer", "c"); question2.check(); question2.showResults(); MultipleChoiceQuestion question3 = new MultipleChoiceQuestion("Buying and selling on the internet is called?", "Consumerism", "E-Commerce", "Globalization", "The Free Market", "b"); question3.check(); question3.showResults(); MultipleChoiceQuestion question4 = new MultipleChoiceQuestion("What does URL stand for?", "Universal Resource Locale", "Universal Reading Location", "Universal Resource Logistic", "Uniform Resource Locator", "d"); question4.check(); question4.showResults(); MultipleChoiceQuestion question5 = new MultipleChoiceQuestion("Data information is stored in the computer as ?", "Files", "Floppies", "Directories", "Processors", "a"); question5.check(); question5.showResults(); MultipleChoiceQuestion.showResults(); } private static MultipleChoiceQuestion question() { // TODO Auto-generated method stub return null; } }
Please help me with my Java assignment. I need to know why I can't seem to get rid of my bug errors and if I had indented wrong because I'm supposed to get my dialog box pop up and it doesn't even if I did overcame my bugs. My assignment is below this message as follows:
import javax.swing.JOptionPane;
public class MultipleChoiceQuestion {
static int correct = 0;
static int incorrect = 0;
String question;
String correctAnswer;
MultipleChoiceQuestion(String query, String a, String b, String c, String d,
String e, String Answer) {
question = query+"\n";
question = "A. "+a+"\n";
question = "B. "+b+"\n";
question = "C. "+c+"\n";
question = "D. "+d+"\n";
question = "E. "+e+"\n";
correctAnswer = Answer.toUpperCase();
}
String ask() {
while (true) {
String answer = JOptionPane.showInputDialog(question);
answer =answer.toUpperCase();
}
String ask() {
while (true) {
String answer = JOptionPane.showInputDialog(question);
answer=answer.toUpperCase();
boolean valid = (answer.equals("A")||answer.equals("B")||
answer.equals("C")|| answer.equals("D")||answer.equals("E"));
If(valid) return answer;
JOptionPane.showMessageDialog(null,"Invalid answer. Please enter" A, B, C, D, or
E.");
}
}
void check() {
nQuestions++;
String answer = ask();
if (answer.equals(correctAnswer)) {
JOptionPane.showMessageDialog(null,"Correct!");
int nCorrect = 0;
nCorrect++;
}else {
JOptionPane.showMessageDialog(null,"Incorrect. The correct answer is + "+correctAnswer+".");
}
}
static void showResults() {
Object nCorrect = null;
Object nQuestions = null;
JOptionPane.showMessageDialog(null,nCorrect +"correct out of "+ nQuestions +" questions");
}
}
public class Quiz {
public static void main(String[] args) {
MultipleChoiceQuestion question = new MultipleChoiceQuestion("What is a quiz?",
"a test of knowledge, especially a brief informal test given to students",
"42",
"a duck",
"to get to the other side",
"To be or not to be, that is the question.",
question.check();
question.showResults();
MultipleChoiceQuestion question1 = new MultipleChoiceQuestion("Who was the first president of the United States?",
"Washington",
"Jefferson",
"Lincoln",
"Clinton",
"a");
question1.check();
question1.showResults();
MultipleChoiceQuestion question2 = new MultipleChoiceQuestion("The World Wide Web is also known?",
"Digital Magician",
"Information Age",
"Internet",
"Personal Computer",
"c");
question2.check();
question2.showResults();
MultipleChoiceQuestion question3 = new MultipleChoiceQuestion("Buying and selling on the internet is called?",
"Consumerism",
"E-Commerce",
"Globalization",
"The Free Market",
"b");
question3.check();
question3.showResults();
MultipleChoiceQuestion question4 = new MultipleChoiceQuestion("What does URL stand for?",
"Universal Resource Locale",
"Universal Reading Location",
"Universal Resource Logistic",
"Uniform Resource Locator",
"d");
question4.check();
question4.showResults();
MultipleChoiceQuestion question5 = new MultipleChoiceQuestion("Data information is stored in the computer as ?",
"Files",
"Floppies",
"Directories",
"Processors",
"a");
question5.check();
question5.showResults();
MultipleChoiceQuestion.showResults();
}
private static MultipleChoiceQuestion question() {
// TODO Auto-generated method stub
return null;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

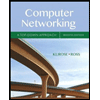
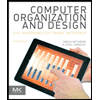
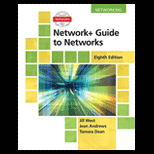
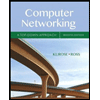
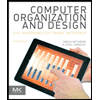
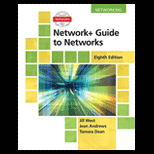
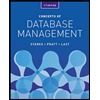
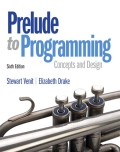
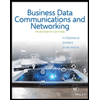