In C++, Implement a Priority Queue(PQ) using an UNSORTED LIST. Use an array size of 20 elements. Use a circular array: Next index after last index is 0. Add the new node to next available index in the array. When you add an element, add 1 to index (hit max index, go to index 0). Test if array in full before you add. When you remove an element, from the list, move the following elements to the left to fill in the blank, etc ( Like prior program done with LISTS ) Create a class called Node: Have a Name and Priority. Data set - 1 is the highest priority, 10 is lowest priority. Enqueue and dequeue in the following order. Function Name, Priority Enqueue Joe, 3 Enqueue Fred, 1 Enqueue Tuyet, 9 Enqueue Jose, 6 Dequeue Enqueue Jing, 2 Enqueue Xi, 5 Enqueue Moe, 3 Dequeue Enqueue Miko, 7 Enqueue Vlady, 8 Enqueue Frank, 9 Enqueue Anny, 3 Dequeue Enqueue Xi, 2 Enqueue Wali, 2 Enqueue Laschec, 6 Enqueue Xerrax, 8 Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue
In C++, Implement a Priority Queue(PQ) using an UNSORTED LIST. Use an array size of 20 elements. Use a circular array: Next index after last index is 0. Add the new node to next available index in the array. When you add an element, add 1 to index (hit max index, go to index 0). Test if array in full before you add. When you remove an element, from the list, move the following elements to the left to fill in the blank, etc ( Like prior program done with LISTS ) Create a class called Node: Have a Name and Priority. Data set - 1 is the highest priority, 10 is lowest priority. Enqueue and dequeue in the following order. Function Name, Priority Enqueue Joe, 3 Enqueue Fred, 1 Enqueue Tuyet, 9 Enqueue Jose, 6 Dequeue Enqueue Jing, 2 Enqueue Xi, 5 Enqueue Moe, 3 Dequeue Enqueue Miko, 7 Enqueue Vlady, 8 Enqueue Frank, 9 Enqueue Anny, 3 Dequeue Enqueue Xi, 2 Enqueue Wali, 2 Enqueue Laschec, 6 Enqueue Xerrax, 8 Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue Dequeue
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
In C++, Implement a Priority Queue(PQ) using an UNSORTED LIST.
Use an array size of 20 elements. Use a circular array: Next index after last index is 0. Add the new node to next
available index in the array. When you add an element, add 1 to index (hit max index, go to index 0). Test if array in full
before you add. When you remove an element, from the list, move the following elements to the left to fill in the blank,
etc ( Like prior program done with LISTS )
Create a class called Node: Have a Name and Priority.
Data set - 1 is the highest priority, 10 is lowest priority.
Enqueue and dequeue in the following order.
Function Name, Priority
Enqueue Joe, 3
Enqueue Fred, 1
Enqueue Tuyet, 9
Enqueue Jose, 6
Dequeue
Enqueue Jing, 2
Enqueue Xi, 5
Enqueue Moe, 3
Dequeue
Enqueue Miko, 7
Enqueue Vlady, 8
Enqueue Frank, 9
Enqueue Anny, 3
Dequeue
Enqueue Xi, 2
Enqueue Wali, 2
Enqueue Laschec, 6
Enqueue Xerrax, 8
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Dequeue
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
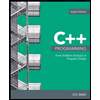
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
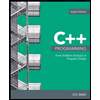
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning