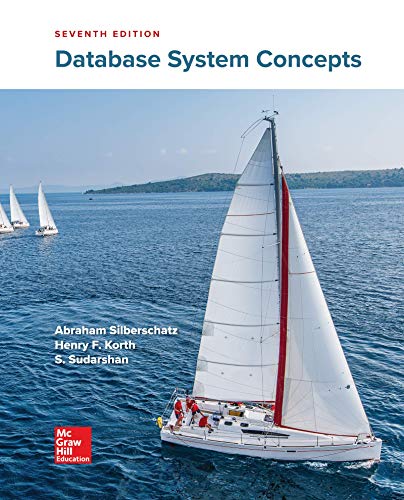
Please answer point 3 and 4
In Data Structures, you studied binary heaps. Binary heaps support the insert and extractMin
functions in O(lgn), and getMin in O(1). Moreover, you can build a heap of n elements in
just O(n). Refresh your knowledge of heaps from chapter no. 6 of your algorithms text book.
Now implement Merge Sort, Heap Sort, and Quick Sort in C++ and perform the following
experiment:
1. Generate an Array A of 10^7 random numbers. Make its copies B and C. Sort A using
Merge Sort, B using Heap Sort, and C using Quick Sort.
2. During the sorting process, count the total number of comparisons between array ele-
ments made by each
function to compare numbers, which increments a count variable each time it is called.
3. Repeat this process 5 times to compute the average number of comparisons made by
each algorithm.
4. Present these average counts in a table. These counts give you an indication of how the
dierent algorithms compare asymptotically (in big-O terms) for a large value of n.
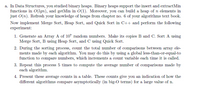

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Q3 QuickSort Given the QuickSort implementation from class, provide an 18-element list that will take the least number of recursive calls of QuickSort to complete. As a counter-example, here is a list that will cause QuickSort to make the MOST number of recursive calls: public static List input() { return Accaxsaslist(0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0); } wwwwwww And here's the QuickSort algorithm, for convenience: algorithm QuickSart Input: lists of integers 1st of size N Output: new list with the elements of lat in sorted order if N < 2 return st pivot Jat[N-1] left = new empty list right = new empty list for index = 0, 1, 2, if lst[i]<= pivot left.add(lat[i]) = else N-2 right add(lat[i]) return QuickSort(left) + [pivot] + QuickSort (right)arrow_forwardI need help with this pratice run question. In C++, Implement a Hash Table of size 8191 (= 2^13 - 1) to store integers based on quadratic probing. Design your own hash function that is, in your view, sufficiently non-trivial and efficient to compute. Compare the average number of probes for the following cases:Generate 4000 integer random numbers in the range 0 to 10^6 - 1, and insert them into the hash table. Compute and print the total number of probes.Repeat step (a) above 10 times, and find the average number of probes over these 10 trialsarrow_forwardWrite a C++ program that: (1) defines and implements a hash class that constructs a 15 element array (may be implemented using a vector, a deque, or a list, if you prefer, (using the STL implementations), storing strings, using the following hash function: ((first_letter) + (last_letter) - (second_letter))% 15 (2) the driver program should: a. query the user for ten words and store them using the hash technique described above. b. print out the contents of each position of the array (or vector, deque, or whatever you used), showing vacant as well as filled positions. Remember, only 10 of the 15 positions will be filled. c. repeatedly query the user for a target word, hash the word, check for its inclusion in the list of stored words, and report the result. Continue doing this task until the user signals to stop (establish a sentinel condition).arrow_forward
- PLEASE READ: Do not code unless the question asks for it, then code in java. Importantly and clearly answer the question by explaining with words fully first, thank youarrow_forwardyou’re going to be creating C++ a Heap Sorter. It should be able to take input (using CIN), place it into proper Max Heap form in an array, and once it’s done accepting input, put the array in order using a Heap Sort. For the sake of simplicity, our heap trees will be made of an array that’s only seven elements long. Make sure to define your arrays ahead of time with garbage filler data before you start putting in your CIN data.Remember to use the binary heap traversal trick shown in lecture when dealing with your array-based tree.Please sort the following lists of inputs:1.) 12, 40, 2, 6, 88, 90, 52.) 1, 2, 3, 4, 5, 6, 73.) 7, 6, 5, 4, 3, 2, 14.) 42, 64, 355, 113, 101, 13, 355.) 12, -5, 24, -4, 48, -3, 96arrow_forwardJava Quick Sort but make it read the data 10, 7, 8, 9, 1, 5 from a file not an array // Java implementation of QuickSort import java.io.*; class GFG { // A utility function to swap two elements static void swap(int[] arr, int i, int j) { int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } /* This function takes last element as pivot, places the pivot element at its correct position in sorted array, and places all smaller (smaller than pivot) to left of pivot and all greater elements to right of pivot */ static int partition(int[] arr, int low, int high) { // pivot int pivot = arr[high]; // Index of smaller element and // indicates the right position // of pivot found so far int i = (low - 1); for (int j = low; j <= high - 1; j++) { // If current element is smaller // than the pivot if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
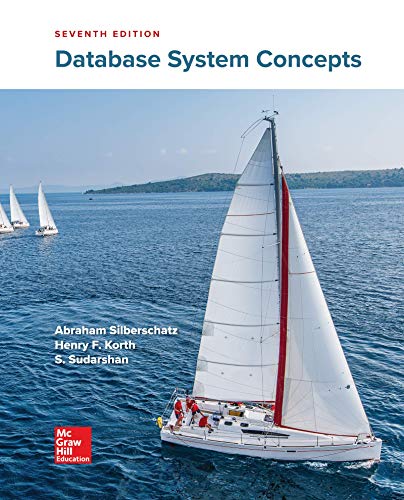
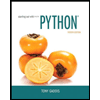
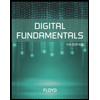
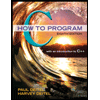
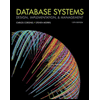
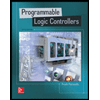