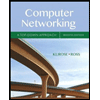
in java eclipse. Please add comments in each step. I have stared the code please in the same code add the following question. Thank you
I have done this part(this is only for reference):
Part 1:
a. Create a 1D array of integers to store 50 integers.
b.Store values from 0 to 49 into the array you just created.
c.Create a new String Object using no-arg constructor.
d.Using for loop append the array elements one by one to the String (one per loop iteration)
e.Record and display a run-time it took to append all integers to the String (record run-time of 1.d.)).
E.g. “It took ______ nanoseconds to append 50 integers to the String
This part is what I want please.
f. Create a new StringBuilder Object using no-arg constructor.
g. Using for loop append the array elements one by one to the StringBuilder (one per loop iteration)
h. Record a run-time it took to append all integers to the StringBuilder (record run-time of 2.g.))
E.g. “It took ______ nanoseconds to append 50 integers to the StringBuilder
i. Repeat the testing with 500 and 5,000 elements by again creating new integer arrays with 500 and 5000 sizes respectively.
j. Record and display the run-time it took to append all integers to the String and StringBuilder respectively.
k. Submit the screenshot of results and a small summary in the form of a table below :
Time / size | 50 | 500 | 5,000 |
String | |||
StringBuilder |
The image is the code started.
I'll appreciate your help. I have asked the same questions multiple times.
![```java
package package2;
public class VsStringBuilder {
public static void main(String[] args) {
// change the SIZE to 500, 5000 to repeat for big array size
final int SIZE = 50;
System.out.println("Array Size: " + SIZE);
//a
int[] array = new int[SIZE];
//b
for (int i = 0; i < SIZE; i++) array[i] = i;
//c
String stringObject = new String();
// d record time
long startTime = System.currentTimeMillis();
for (int i = 0; i < SIZE; i++) stringObject += array[i];
long endTime = System.currentTimeMillis();
//e
System.out.println("Time to append using string object: " + (endTime - startTime) + " ms.");
//f
StringBuilder builder = new StringBuilder();
startTime = System.currentTimeMillis();
for (int i = 0; i < SIZE; i++) builder.append(array[i]);
endTime = System.currentTimeMillis();
//e
System.out.println("Time to append using string builder object: " + (endTime - startTime) + " ms.");
}
}
```
### Explanation
This Java program compares the performance of using a `String` object versus a `StringBuilder` to append integers from an array.
1. **Array Initialization**:
- A constant `SIZE` determines the array's size.
- An integer array `array` is initialized with a size `SIZE`.
2. **Array Population**:
- The array is populated with integers from `0` to `SIZE - 1`.
3. **Appending with String**:
- A `String` object `stringObject` is used.
- The time taken to append array elements to `stringObject` is measured and printed.
4. **Appending with StringBuilder**:
- A `StringBuilder` object `builder` is used.
- The time taken to append array elements to `builder` is measured and printed.
### Performance Consideration
- **`String`**:
- Strings are immutable in Java, meaning every modification creates a new `String` object, leading to increased overhead in appending operations.
- **`StringBuilder`**:
- A `](https://content.bartleby.com/qna-images/question/e4e72d86-d216-4977-8ee6-fe536869a207/04e964bf-000b-45eb-913a-262e2e64cb1e/j2v5wuf_thumbnail.jpeg)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 6 images

- Hello! I need some help with my Java homework. Please use Eclipse Please add comments to the to program so I can understand what the code is doing and learn Create a new Eclipse project named so as to include your name (eg smith15 or jones15). In this project, create a new package with the same name as the project. In this package, write a solution to the exercise noted below. Implement the following method that returns the maximum element in an array: public static <E extends Comparable<E>> E max(E[] list) Write a test program that generates 10 random integers, invokes this method to find the max, and then displays the random integers sorted smallest to largest and then prints the value returned from the method. Max sure the the last sorted and returned value as the same!arrow_forwardDraw a square with a Pentagram hole! The 3×3 square extends from −0.7 to 2.3 on the X axis and from −0.3 to 2.7 on the Y axis. Keep the X and Y arrays named xp and yp, respectively. The template code it's below. I need help with the ellipsis. import matplotlib.pyplot as plt xp = [0, 0.81, 1.62, 1.31, 2.12, 1.12, 0.81, 0.5, -0.5, 0.31, 0] + [None] +[..., ..., ..., ..., ...] yp = [0, 0.59, 0, 0.95, 1.54, 1.54, 2.49, 1.54, 1.54, 0.95, 0] + [None] + [..., ..., ..., ..., ...] plt.axis("equal") plt.axis("off") plt.fill(xp, yp) plt.show() Expected result:arrow_forwardin Java, please. Please add comments. Thank youarrow_forward
- 4. Now examine the ArrayList methods in the above table, a) Which method retrieves elements from the ArrayList? b) Which method replaces the value of an element that already exists in the ArrayList? c) Which two methods initializes the value of an element? d) How do the two methods in (c) differ? Which method(s) would be appropriate in the above Java program (after we convert it to work with ArrayLists)?arrow_forwardMethod Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forwardin java eclipse. Please add comments. I have stared the code please in the same code add the following question. Thank you f. Create a new StringBuilder Object using no-arg constructor. g. Using for loop append the array elements one by one to the StringBuilder (one per loop iteration) h. Record a run-time it took to append all integers to the StringBuilder (record run-time of 2.g.)) i. Repeat the testing with 500 and 5,000 elements by again creating new integer arrays with 500 and 5000 sizes respectively. j. Record and display the run-time it took to append all integers to the String and StringBuilder respectively. k. Submit the screenshot of results and a small summary in the form of a table below : Time / size 50 500 5,000 String StringBuilder The image is the code started.arrow_forward
- Create an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time.arrow_forward1. Declare an array to hold eight integers. Use a for loop to add eight random integers, all in the range from 50 to 100, inclusive, to this array. Duplicates are okay. Next, pass the array to a method that sorts the array and returns another array containing only the largest and smallest elements in the original array. Print these two values in main. Then use a foreach loop to display all elements of the sorted array on one line separated by a single space. This latter loop should also count the odd and even numbers in the array and determine the sum of all elements in the array. SAMPLE OUTPUT The lowest element is 59 The highest element is 96 Here is the array 59 64 76 77 80 88 91 96 Evens: 5, odds: 3 Total: 631arrow_forwardJavascript Use a for/of loop to iterate over the array of students. For each student, use a template literal to print out their name, age, and major in a formatted string. (e.g. John is 18 years old and is studying Computer Science.) Within the template literal, use object dot notation to access the name, age, and major properties of the student object. Use a console.log() to print out the formatted string for each student. Test the code by running it and verifying that it prints out the details of each student in a formatted string.============================================================================== const students = [ { name: "John", age: 18, major: "Computer Science" }, { name: "Newton", age: 19, major: "Mathematics" }, { name: "Barry", age: 20, major: "Physics" }, ]; // Iterate through the array of objects students using for/of // Print a message to the console that includes the student's name, age, and major // Example: John is 18 years old and…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
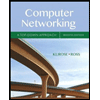
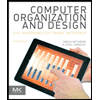
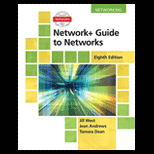
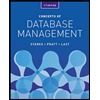
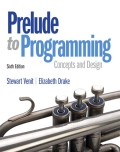
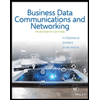