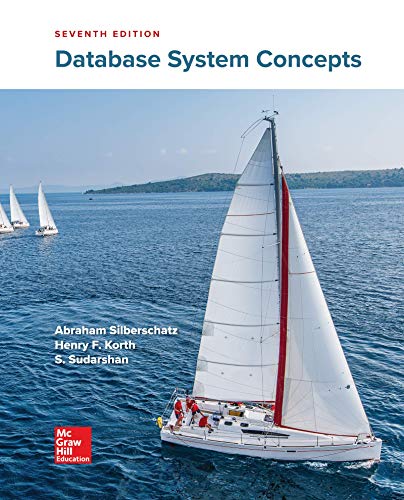
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
in java
Strings itemToFind and inputItem are read from input. Integer wordCount is initialized with 1. Write a while loop that iterates until inputItem is equal to "Done". In each iteration of the loop:
- Increment wordCount if inputItem is equal to itemToFind.
- Read string inputItem from input.
Click here for example
Note: Assume that input has at least two strings.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
import java.util.Scanner;
public class SimpleWhileLoop {
publicstaticvoidmain(String[] args) {
Scannerscnr=newScanner(System.in);
StringitemToFind;
StringinputItem;
intwordCount;
itemToFind=scnr.next();
inputItem=scnr.next();
wordCount=1;
/* Your code goes here */
System.out.println(itemToFind+" occurs "+wordCount+" time(s).");
}
}
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- String personName is read from input. Output personName left aligned with a width of 6, followed by " Taylor". End with a newline. Ex: If the input is Juno, then the output is: Juno Taylor Use Javaarrow_forwardJAVA PROGRAMMING LAB Write a Java program to takes 2 numbers from the user and print true if one or the other is teen, but not both. "teen" number is that number which lies in the range 13..19 inclusive.arrow_forwardRead string integer value pairs from input until "Done" is read. For each string read: • If the following integer read is less than 40, output the string followed by ": low on stock". • Otherwise, output the string followed by ": well stocked". End each output with a newline. Ex: If the input is Oven 43 Curtain 7 Pillow 1 Done, then the output is: Oven: well stocked Curtain: low on stock Pillow: low on stock 1 #include 2 #include 3 using namespace std; 4 5 int main() { 6 7 8 9 10 } * Your code goes here */ return 0;arrow_forward
- Java: Receives a phrase and returns the converted phrase to Pig Latin. A word is translated into Pig Latin according to the following rules: a. If there are no vowels in the word, then add “ay” to the end of it. (a, e, i, o, and u are all the vowels considered). b. If the word begins with a vowel, then add “yay” to the end of it. c. Otherwise, take all the letters up to the first vowel and move those letters to the end then add “ay”. Sample Input: System.out.println(toPigLatin("I am fluent in Pig Latin")); System.out.println(toPigLatin(“Hey”)); Sample Output: Iyay amyay uentflay inyay igPay atinLay Heyayarrow_forwardAccumulating Totals in a Loop Summary In this lab, you add a loop and the statements that make up the loop body to a Java program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed. The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X Variables have been declared for you, and the input and output statements have been written. Instructions Ensure the file named LeftOrRight.java is open. Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class. Execute the program by clicking Run and using the data listed above and verify that the output is correct.arrow_forwardMad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways. Write a program that takes a string and an integer as input, and outputs a sentence using the input values as shown in the example below. The program repeats until the input string is quit and disregards the integer input that follows. Ex: If the input is: apples 5 shoes 2 quit 0 the output is: Eating 5 apples a day keeps the doctor away. Eating 2 shoes a day keeps the doctor away.arrow_forward
- PrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardIn java, create a program that will use a do while loop with a "yes/no" prompt. The program should include user input and output with getters and setters. Once the end of the loop is reached, ask the user if they'd like to do the program again. Answering "Yes" will start the program over again. Answering "No" will end the program. Answering with anything else will be an invalid input. Once the loop begins again, the program should also discard all previous inputs so that there won't be any overlapping. Use Scanner.nextLine();, it will help.arrow_forwardPython only 1. Define printGrid Use def to define printGrid Use any kind of loop Within the definition of printGrid, use any kind of loop in at least one place. Use any kind of loop Within the loop within the definition of printGrid, use any kind of loop in at least one place. it should look like this when called ; printGrid(['abcd','efgh','ijkl']) Prints: a b c d e f g h i j k l 2. Define getColumns Use def to define getColumns Use any kind of loop Within the definition of getColumns, use any kind of loop in at least one place. Use a return statement Within the definition of getColumns, use return _ in at least one place. it should look like this when called ; getColumns(['abcd','efgh','ijkl']) Out[]: ['aei', 'bfj', 'cgk', 'dhl']arrow_forward
- In Coral Language 3.22 LAB: Loops: Countdown until matching digits Write a program that takes in an integer in the range 20-98 as input. The output is a countdown starting from the integer, and stopping when both output digits are identical. Ex: If the input is: 93 the output is: 93 92 91 90 89 88 Ex: If the input is: 77 the output is: 77 Ex: If the input is: 9 or any number not between 20 and 98 (inclusive), the output is: Input must be 20-98 For coding simplicity, follow each output number by a space, even the last one. Use a while loop. Compare the digits; do not write a large if-else for all possible same-digit numbers (11, 22, 33, …, 88), as that approach would be cumbersome for large ranges.arrow_forwardPython code easy way pleasearrow_forwardIntro to Java Instuctions: Design and implement a program that reads a series of 10 integers from the user and prints their average For each of the 10 numbers input from the user: one at a time, prompt the user to enter a number. Then read input from the user as a string. Attempt to convert it to an integer using the "Integer.parseInt" method. If the process throws a "NumberFormatExeption", print an appropriate error message and prompt the user for the number again. Continue prompting and reading in number until 10 valid integers have been read Print "The average is" and then print the average of the 10 numbers Code: import java.util.Scanner; public class Lab1MainClass { public static final int VALUES_TO_READ = 10; public static void main(String[] args) { // local variables int[] values = new int [VALUES_TO_READ]; double average; Scanner scan = new Scanner(System.in); int values Read = 0; // how many numbers the user has given us, a count of where we are String userInput; // read…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
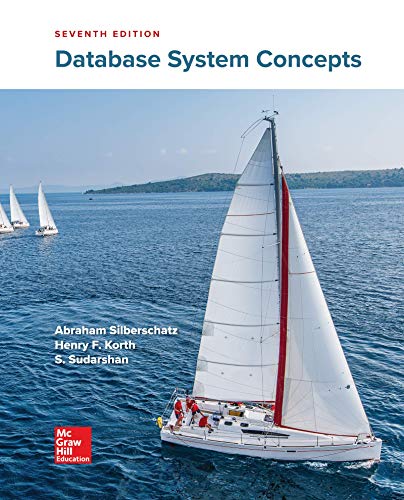
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
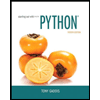
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
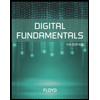
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
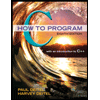
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
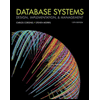
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
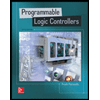
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education