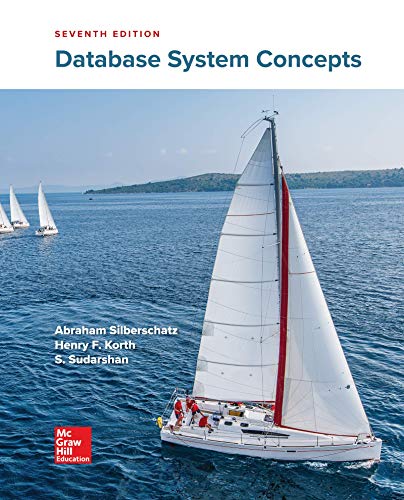
In Python:
Given 4 floating-point numbers. Use a string formatting expression with conversion specifiers to output their product and their average as integers (rounded), then as floating-point numbers.
Output each rounded integer using the following:
print('{:.0f}'.format(your_value))
Output each floating-point value with three digits after the decimal point, which can be achieved as follows:
print('{:.3f}'.format(your_value))
My original code:
import math
num1 = float(input())
num2 = float(input())
num3 = float(input())
num4 = float(input())
num_product_float = num1 * num2 * num3 * num4
num_product = int(num_product_float)
num_average_float = (num1 + num2 + num3 + num4) / 4
num_average = int(num_average_float)
print('{:.0f} {:.0f}'.format(num_product, num_average))
print('{:.3f} {:.3f}'.format(num_product_float, num_average_float))
num_product_float = num1 * num2 * num3 * num4
num_product = math.ceil(num_product_float)
So the first input set (8.3 10.4 5.0 4.8) is now coming out correct, but the second is wrong:
num_average_float = (num1 + num2 + num3 + num4) / 4
num_average = math.floor(num_average_float)
Now I got the first and second input values correct, however the third is STILL coming out wrong:

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Write a python program that computes the pay and performs the following tasks: - prompt the user to enter the worked hours; the prompt should use the string "Enter Hours: " - prompt the user to enter the rate; the prompt should use the string "Enter Rate: "- compute the pay-print the pay as: "Pay: Scalculated_pay" - the program should support floating point numbersarrow_forwardA County collects property taxes on the assessment value of the property, which is 60 percent of the property's actual value. For example if an acre of land is valued $10,000.00 its assessment value is $6000. The property tax is 72 cents for each $100.00 of the assessment value. The tax for the assessed at $6000 will be $43.20. Write a PYTHON program using FUNCTIONS that asks for the actual value of a piece of property and displays the assessment value and property taxarrow_forwardPYTHON write a program that assigns the principal amount of P10000 to variable P, assign to n the value 12, and assign to r the interest rate of 8%. then have the program prompt the user for the number of years t that the money will be compounded for. calculate and print the final amount after t years. use the formula below.arrow_forward
- PYTHON The metric system uses as a basic measure the meter and the imperial system has its basic measure as feet and inches. Let’s implement two functions, one to convert feet to meter and another to convert from meter to feet. The conversion formulas are the following:\n", " $$1 meter = 3.2808 feet \\\\\n", " 1 foot = 0.3048 meters $$\n", "\n", "Now implement a program that asks the user what type of conversion he wants to make and asks what value he wants to convert. Process the information and prints the value converted. Test your code with the values:\n", "```\n", "10 meters ==> 32.808 feet\n", "10 feet ==> 3.048 meters\n",arrow_forwardCode should be in Python, please 1. Write a program with a car's miles/gallon and gas dollars/gallon (both floats) as input, and output the gas cost for 10 miles, 50 miles, and 400 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print('{:.2f}'.format(your_value)) Ex: If the input is: 20.0 3.1599 the output is: 1.58 7.90 63.20 Your program must define and call the following driving_cost() function. Given input parameters driven_miles, miles_per_gallon, and dollars_per_gallon, the function returns the dollar cost to drive those miles. Ex: If the function is called with: 50 20.0 3.1599 the function returns: 7.89975 def driving_cost(driven_miles, miles_per_gallon, dollars_per_gallon) Your program should call the function three times to determine the gas cost for 10 miles, 50 miles, and 400 miles.arrow_forwardRun a python program that will receive a chemical formula as an input and will give its molar mass as an output.you can get a dictionnary of the molar Mass of each component (Hydrogen, Oxygen, .) Inputs: Print(" Enter the Chemical Formula" ) : H2O a. Chemical Formula like H2O and with parantheses Fe(C0)3 and with brackets [ ] Output a. Molar Mass : 18 Please create a program like this. If not familiar with chemistry, please create a similar program.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
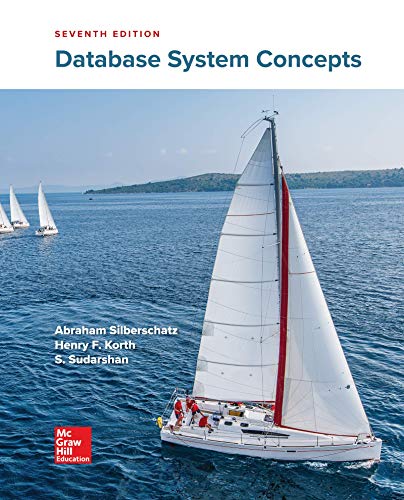
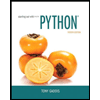
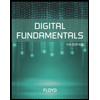
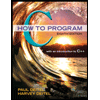
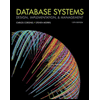
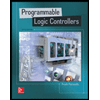