In this lab, you complete a partially prewritten C++ program that uses an array. The program prompts the user to interactively enter eight batting averages, which the program stores in an array. The program should then find the minimum and maximum batting average stored in the array as well as the average of the eight batting averages. The data file provided for this lab includes the input statement and some variable declarations. Comments are included in the file to help you write the remainder of the program.
In this lab, you complete a partially prewritten C++ program that uses an array.
The program prompts the user to interactively enter eight batting averages, which the program stores in an array. The program should then find the minimum and maximum batting average stored in the array as well as the average of the eight batting averages. The data file provided for this lab includes the input statement and some variable declarations. Comments are included in the file to help you write the remainder of the program.
Instructions
-
Ensure the source code file named BattingAverage.cpp is open in the code editor.
-
Write the C++ statements as indicated by the comments.
-
Execute the program by clicking the Run button. Enter the following batting averages: .299, .157, .242, .203, .198, .333, .270, .190. The minimum batting average should be .157, and the maximum batting average should be .333. The average should be .2365.
GIVEN CODE

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

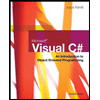
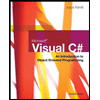