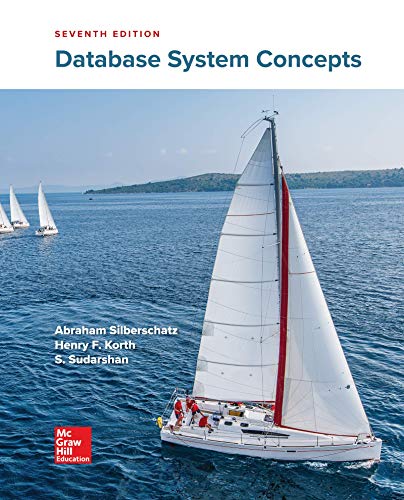
In this programming problem you'll code up Dijkstra's shortest-path
Download the following text file:
The file contains an adjacency list representation of an undirected weighted graph with 200 vertices labeled 1 to 200. Each row consists of the node tuples that are adjacent to that particular vertex along with the length of that edge. For example, the 6th row has 6 as the first entry indicating that this row corresponds to the vertex labeled 6. The next entry of this row "141,8200" indicates that there is an edge between vertex 6 and vertex 141 that has length 8200. The rest of the pairs of this row indicate the other vertices adjacent to vertex 6 and the lengths of the corresponding edges.
Your task is to run Dijkstra's shortest-path algorithm on this graph, using 1 (the first vertex) as the source vertex, and to compute the shortest-path distances between 1 and every other vertex of the graph. If there is no path between a vertex vv and vertex 1, we'll define the shortest-path distance between 1 and vv to be 1000000.
You should report the shortest-path distances to the following ten vertices, in order: 7,37,59,82,99,115,133,165,188,197. You should encode the distances as a comma-separated string of integers. So if you find that all ten of these vertices except 115 are at distance 1000 away from vertex 1 and 115 is 2000 distance away, then your answer should be 1000,1000,1000,1000,1000,2000,1000,1000,1000,1000. Remember the order of reporting DOES MATTER, and the string should be in the same order in which the above ten vertices are given. The string should not contain any spaces. Please type your answer in the space provided.
IMPLEMENTATION NOTES: This graph is small enough that the straightforward O(mn)O(mn) time implementation of Dijkstra's algorithm should work fine. OPTIONAL: For those of you seeking an additional challenge, try implementing the heap-based version. Note this requires a heap that supports deletions, and you'll probably need to maintain some kind of mapping between vertices and their positions in the heap.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- The implementations of the methods addAll, removeAll, retainAll, retainAll, containsAll(), and toArray(T[]) are omitted in the MyList interface. Implement these methods. Use the template at https://liveexample.pearsoncmg.com/test/Exercise24_01_13e.txt to implement these methods this the code i have for the write your own code part import java.util.Iterator; interface MyList<E> extends java.util.Collection<E> { void add(int index, E e); boolean contains(Object e); E get(int index); int indexOf(Object e); int lastIndexOf(E e); E remove(int index); E set(int index, E e); void clear(); boolean isEmpty(); Iterator<E> iterator(); boolean containsAll(java.util.Collection<?> c); boolean addAll(java.util.Collection<? extends E> c); boolean removeAll(java.util.Collection<?> c); boolean retainAll(java.util.Collection<?> c); Object[] toArray(); <T> T[] toArray(T[] a); int size();} and its…arrow_forwardWrite a python program that creates a dictionary whose values are lists of anagrams of words read from a file. using: def norm(s): def AnagramDicionary(f): def printWordList(L): def main():arrow_forwardPython please Use map(s) to program this formula: Σ [ (xi – x)(yi – y) ] / Σ [ (xi – x)^2] Note the Σ symbol means "SUM" where the sum goes from 0 to a limit. And the ^2 at the end of the equation means 'squared'.arrow_forward
- Task 4: Matplotlib Import the plotting function by the command: import matplotlib.pyplot as plt Plotting multiple lines Compute the x and y coordinates for points on sine and cosine curves and plot them on the same graph using matplotlib. Add x and y labels to the graph as well. Note: Please provide PYTHON (ANACONDA) code for above task.arrow_forwardFIX THIS CODE Using python Application CODE: import csv playersList = [] with open('Players.csv') as f: rows = csv.DictReader(f) for r in rows: playersList.append(r) teamsList = [] with open('Teams.csv') as f: rows = csv.DictReader(f) for r in rows: teamsList.append(r) plays=len(playersList) for i in range(plays): if playersList[i]['team']=='Argentina' and int(playerlist[i]['minutes played'])<200 and int(playersList[i] ['shots'])>20: print(playersList[i]['last name']) c0=0 c1=0 c2=0 for i in range(len(teamsList)): if int(teamsList[i]['redCards'])==0: c0=c0+1 if int(teamsList[i]['redCards'])==1: c1=c1+1 if int(teamsList[i]['redCards'])==2: c2=c2+1 print("Number of teams with zero redcards:",c0) print("Number of teams with zero redcards:",c1) print("Number of teams with zero redcards:",c2) ratio=0 for i in range(len(teamsList)): if int(teamsList[i]['games']>3) and if int(teamsList[i]['goalsFor'])/int(teamsList[i]['goalsAgainst'])<ratio:…arrow_forwardUse Java pl DataFileWords.txt: lovegentlensslovepeacelovekindnesslovepeaceunderstandingkindnessjoypeaceunderstandingfriendshipgentlenssgoodnesslovepeacegoodnesslovegentlensscontrolunderstandingunderstandinggentlensslovepeacepatiencecontrolkindnessgentlensslovepeacejoygoodnesspeacefriendshipgoodnesskindnesslovepeacegoodnesscontrolpatiencejoygentlensspatiencegentlensspatiencekindnessgoodnessjoygentlenssgentlenssarrow_forward
- python: In the Python terminal, the command dir(list) returns a list of operations that can be performed on lists. choose three (3) operations from this list and, in your own words, describe what it does, how to use it, and give your own example. Try to choose operations that have not been completed yet.arrow_forwardRevorse the vewels def reverse_vowels(text): Given a text string, create and return a new string constructed by finding all its vowels (for simplicity, in this problem vowels are the letters found in the string 'aeiouAEIOU') and reversing their order, while keeping all other characters exactly as they were in their original positions. However, to make the result look prettier, the capitalization of each moved vowel must be the same as that of the vowel that was originally in the target position. For example, reversing the vowels of 'Ilkka' should produce 'Alkki' instead of 'alkkI'. Applying this operation to random English sentences seems to occasionally give them a curious pseudo-Mediterranean vibe.Along with many possible other ways to perform this square dance, one straightforward way to reverse the vowels starts with collecting all vowels of text into a separate list, and initializing the result to an empty string. After that, iterate through all positions of the original text.…arrow_forwardputty program for unix can can not get the screen to show the mountain list. how do i do this?arrow_forward
- %matplotlib inlineimport numpy as npfrom matplotlib import pyplot as pltimport matharrow_forwardBEFPULSEMIN 70 75 80 82 65 75 74 73 65 67 80 72 69 81 82 83 68 73 74 76 81 64 85 65 66 62 81 82 74 71 72 76 79 80 84 85 73 68 68 67 68 75 77 72 76 78 82 67 80 76 74 77 73 74 72 76 74 81 75 70 TATISTICSSTUDENTSSURVEYFORR contains the column BEFPULSEMIN (a numerical variable that measures student pulses before completing an online survey). For education purposes, consider this dataset to be a sample of size 60 taken from a much wider population for statistics students. Use R to find a 99% confidence interval for the mean of the population. Choose the most correct (closest) statement below. a. Your confidence interval is (72.38912, 76.41088) beats per minute and it is narrower than a 90% confidence interval created from this data. b. Your confidence interval is (72.88831, 75.91169) beats per minute and is narrower than a 90% confidence interval. c. Your…arrow_forwardComplete the Python program below to list all language names and number of related articles in the order they appear in the URL below from urllib.request import urlopenfrom bs4 import BeautifulSouphtml = urlopen('https://www.wikipedia.org/')bs = BeautifulSoup(html, "html.parser") nameList = #TO DO -- Complete the Codefor name in nameList: print(name.get_text())arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
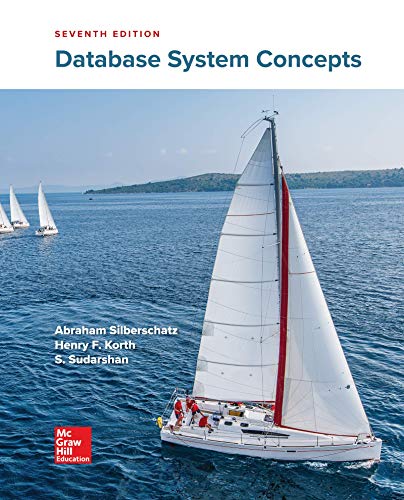
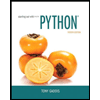
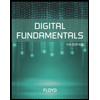
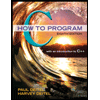
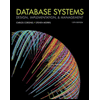
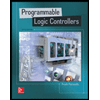