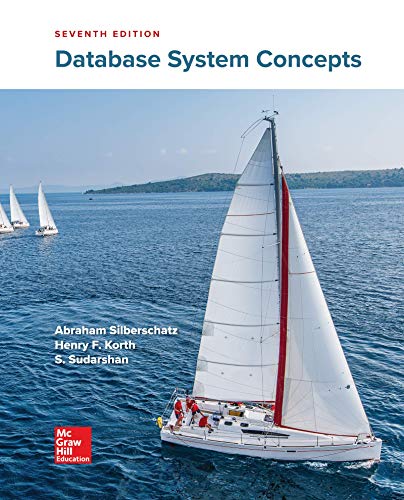
Concept explainers
import java.util.Scanner;
public class LabProgram {
// Recursive method to reverse a string
public static String reverseString(String str) {
// Base case: if the string is empty or has only one character, return the string as is
if (str.isEmpty() || str.length() == 1) {
return str;
} else {
// Recursive step: move the first character to the end and reverse the remaining substring
return reverseString(str.substring(1)) + str.charAt(0);
}
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String input, result;
input = scnr.nextLine();
// Call the reverseString() method
result = reverseString(input);
// Output the result
System.out.printf("Reverse of \"%s\" is \"%s\".%n", input, result);
}
}
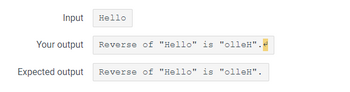

Step by stepSolved in 4 steps with 2 images

- Complete the following recursive function that returns the sum of all the numbers in a list that are positive and even numbers. Replace the bold text with the correct expression. #lang racket (define (positiveEvenNums 1st) (if (null? 1st) (if (THE CAR OF THE LIST IS POSITIVE AND EVEN) (+ (car 1st) (positiveEvenNums (cdr 1st))) (positiveEvenNums (cdr 1st))))) (positiveEvenNums '(-3 8 4 3 -2 0 5))arrow_forwardwrite program that uses recursion to calculate triangular numbers. Enter a value for the term number, n, and the program will display the value of the corresponding triangular number.shows the triangle.cpp program.arrow_forwardSolve in javaFXarrow_forward
- Write a recursive program that takes a positive integer as an input and returns the sum of the digits of the integer. (Example: If the input is 45678, then output will be 30.)arrow_forwardPython Using recursion only No loops Note that in a correct solution the isdigit method or in operator will never be applied to the entire string s. The function must return and not print the resulting string. def getDigits(s):arrow_forwardwrite a code in java (using recursion)arrow_forward
- Write a recursive function to see if the first letter matches the last letter, return the middle letters and check until only 0 or 1 letters are left. It returns True or False. Here is the original code that needs to be checked: # Returns the first character of the string str def firstCharacter(str): return str[:1] # Returns the last character of a string str def lastCharacter(str): return str[-1:] # Returns the string that results from removing the first # and last characters from str def middleCharacters(str): return str[1:-1] def isPalindrome(str): # base case #1 # base case #2 # recursive case pass Python codearrow_forwardWrite code in Java: -Must be recursive import java.util.*; import java.lang.*; import java.io.*; //*Analysis goes here* //*Design goes here* class AllPermutation { public static void displayPermutation(String s) { //*Code goes here* } public static void displayPermutation(String s1, String s2) { //*Code goes here* } } //*Driver class should not be changed* class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); AllPermutation.displayPermutation(input.nextLine()); } }arrow_forwardComplete the following program Multiply.java. This program uses recursion to multiply two numbers through repeated addition. The output should look exactly like what is pictured below. The code should be completed where it states "complete here"arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
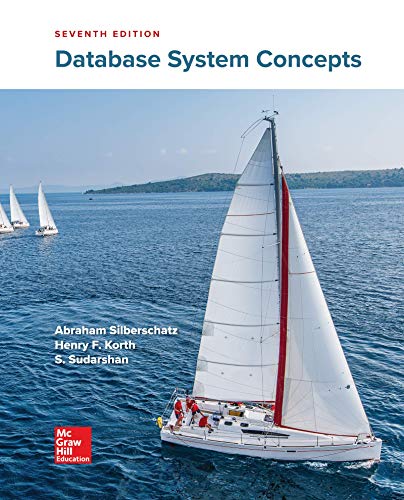
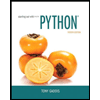
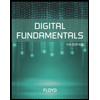
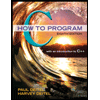
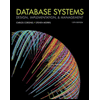
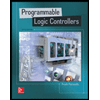